Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial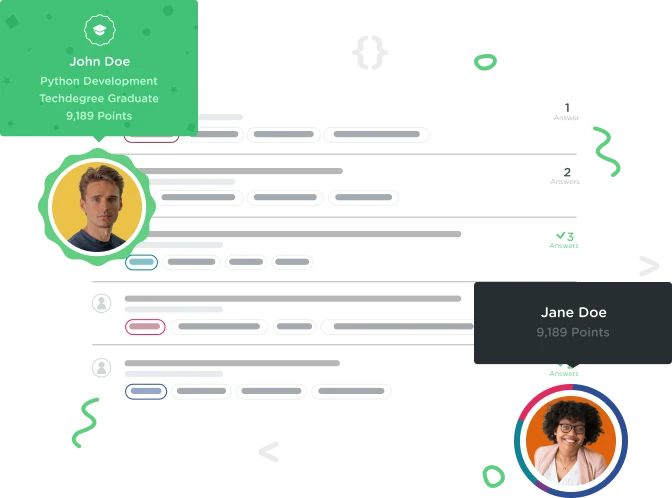

Rodwell Mazambara
1,402 Pointsstring_factory throwing KeyError
When I run this code I get a KeyError for the key 'name'. Where am I getting it wrong?
# Example:
# values = [{"name": "Michelangelo", "food": "PIZZA"}, {"name": "Garfield", "food": "lasagna"}]
# string_factory(values)
# ["Hi, I'm Michelangelo and I love to eat PIZZA!", "Hi, I'm Garfield and I love to eat lasagna!"]
template = "Hi, I'm {name} and I love to eat {food}!"
def string_factory(list_of_dicts):
food_preferences_list=[]
for food_dict in list_of_dicts:
for name,food in food_dict.items():
template.format(name,food)
food_preferences_list.append(template)
return food_preferences_list
1 Answer
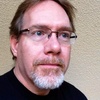
Chris Freeman
Treehouse Moderator 68,461 PointsYour solution is close. There are some items to fix:
- Using the dict method
.items()
is not going to work yo intended as it return the key / value pair for each entry in the dict, such as "food", "Pizza". Perhaps you meant.values()
? - The
format
method creates a new string (because strings are immutable) this newly formatted string is not captured by another variable so gets dropped. The code is appending the sametemplate
every time. - The template string is using named fields, to be sure the
format
arguments are properly received keyword arguments are needed:...format(name=name, food=food)
- using
food_dicts.values()
will return both items, but since it is a dictionary, the order of the items is not guaranteed, meaning that it might be food,name or name, food. There are various ways around this:- drop the inner
for
loop and use double-asterisk expansion: `...format(**food_dict) - explicitly expand the food_dict
...format(name=food_dict['name'],food=food_dict['food'])
- drop the inner
Post back if you need more help. Good luck!!
Edited: Misread using items
instead of values
. Corrected the above text.
Rodwell Mazambara
1,402 PointsRodwell Mazambara
1,402 PointsThanks Chris. You are a saviour! This worked: