Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial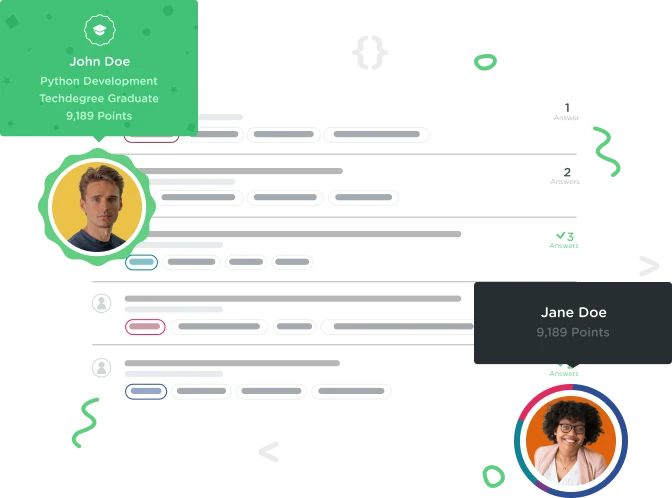

Ronnie Barua
17,665 PointsStuck and not sure if I understand the question
This is what I've done below:
def word_count(item):
count = 0
for word in item.split():
if word in item[word]:
count += 1
return word_count
10 Answers

Geoff Parsons
11,679 PointsYou're close!
The method needs to return a dict
object and since we're going to be manipulating it within the loop we'll want to declare it outside of the loop.
count = {}
Your loops is spot on and the logic inside of it is very close. Instead of checking if a word is in the count we should check to see if it is not in the count so that we can set a default value as we've not seen this word before.
if word not in count:
count[word] = 0
Then we're free to increment the value of that count on the following line like you already have
count[word] += 1
And finally, outside of the loop and conditional, we'll need to return the count.
return count
Putting all those pieces together you end up with something like this:
def word_count(string):
count = {}
for word in string.lower().split():
if word not in count:
count[word] = 0
count[word] += 1
return count

Ronnie Barua
17,665 PointsThanks Geoff! You make perfect sense but unfortunately it doesn't work.

Geoff Parsons
11,679 PointsHrm, seems to be working on this end. What response are you getting back from the code challenge?

Ronnie Barua
17,665 PointsBammer! where is word_count()?

Geoff Parsons
11,679 PointsHrm, it looks like this has happened before and may be a sign that something else is wrong in the code. Sorry for the unhelpful error.
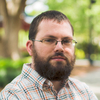
Kenneth Love
Treehouse Guest TeacherFWIW, I can't make the code Geoff Parsons provided fail. Can you give us your exact submission, Ronnie Barua ?

Ronnie Barua
17,665 PointsThanks for trying out.

Ronnie Barua
17,665 PointsThanks Kenneth!
It is this:
def word_count(string):
count = {}
for word in string.lower().split():
if word not in counts:
count[word] = 0
count[word] += 1
return word_count
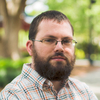
Kenneth Love
Treehouse Guest TeacherSo, looking at this, there's nothing in your function, which causes the interpreter to not register it, so it can't be found. You need to indent all of the content of your function so it's inside the function. like this:
def word_count(string):
count = {}
and so on. Notice that the count
line is indented compared to the line that starts with def
.

Ronnie Barua
17,665 PointsThanks Kenneth.

Ronnie Barua
17,665 PointsSorry! Just keeps on saying Bummer! where's word_count()?
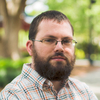
Kenneth Love
Treehouse Guest TeacherYou have some other errors in there. You return word_count
instead of count
. You have counts
when you should have count
. Also, of course, make sure you have the right indentation for the for
loop and the if
condition.

Ronnie Barua
17,665 PointsI've done everything as you said. Now it says some of the words seems to be missing. Just can't figure out what that is.
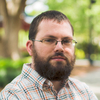
Kenneth Love
Treehouse Guest TeacherI did this and it passed:
def word_count(string):
count = {}
for word in string.lower().split():
if word not in count:
count[word] = 0
count[word] += 1
return count
This is exactly your code from above but with corrected variable names and indentation.

Geoff Parsons
11,679 PointsThat's my bad Ronnie Barua, my original answer had some typos between the in-line explanations and the final result. I've edited it to try to help anyone else that comes across this discussion in the future.

Ronnie Barua
17,665 PointsIt passed. I copied and pasted so that i don't have any error. Thanks so much.

Ronnie Barua
17,665 PointsGeoff I appreciated your help. Don't be silly I make mistakes lot more than you.