Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial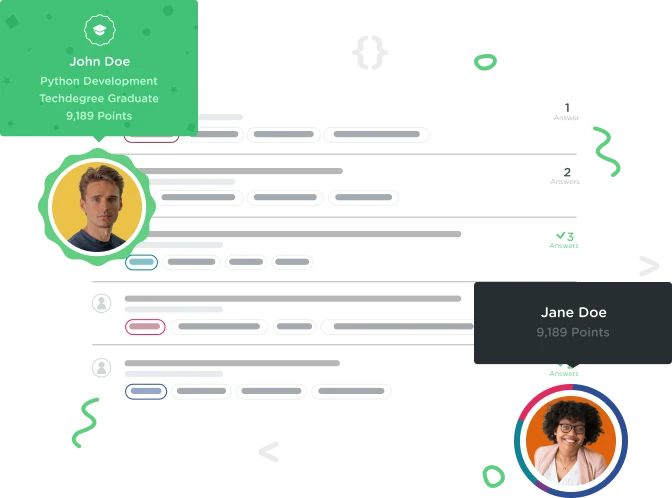
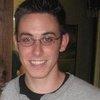
David Evans
10,490 PointsStuck on Challenge for Implementing Interfaces
Receiving the following error message when testing.
Doesn't make sense as execute shouldn't be returning a boolean.
Bummer! Uncaught Error: Call to a member function execute() on boolean in sqlRepository.php:17
<?php
class sqlRepository extends PDO implements RepositoryInterface
{
public function all($entity)
{
$db = new PDO("sqlite:".__DIR__."/database.db");
$query = $db->query("SELECT * FROM " . $entity);
$data = $query->fetchAll(PDO::FETCH_OBJ);
return $data;
}
public function find($table, $value, $field = 'id')
{
$db = new PDO('sqlite:' . __DIR__ . '/database.db');
$query = $db->prepare("SELECT $field FROM $table WHERE value=:value");
$query->execute(array(':value' => $value));
//$result = $query->fetch(PDO::FETCH_ASSOC);
}
}
2 Answers
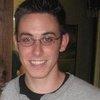
David Evans
10,490 PointsUnsure what was causing the error message in the first place, but I have passed the challenge after modifying my code a bit.
This is what I ended up with.
<?php
class sqlRepository extends PDO implements RepositoryInterface
{
public function all($entity)
{
$query = $this->prepare('SELECT * FROM ' . $entity);
$query->execute();
$results = $query->fetchAll(PDO::FETCH_OBJ);
return $results;
}
public function find($entity, $id, $field='id')
{
$query = $this->prepare('SELECT * FROM ' . $entity . ' WHERE ' . $field . '=:id');
$query->bindValue(':id', $id);
$query->execute();
$results = $query->fetchAll(PDO::FETCH_OBJ);
return $results;
}
}
I swapped out the creation of the PDO object and used $this since we are extending the PDO class.
I could have, and probably should have created a variable for the SQL Query outside of the prepare statement and just used that variable inside the prepare method, but meh it works for now.
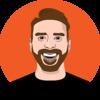
Jorge Saucedo
16,837 PointsHaha! You're great man! You're answering your own question. Hey David, did you find this challenge out of order? I felt that I needed to know a lot more than it was given on the videos to be able to do this challenge.

Arfan Liaqat
1,957 PointsThank you!
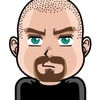
Daryl Peterson
7,812 PointsSince you are extending the PDO object look at the __construct for the PDO object. It make more since to call the parent object __construct and having access to the pdo properties using $this.
<?php class sqlRepository extends PDO implements RepositoryInterface { protected $file;
public function __construct($file)
{
$this->file = $file;
$file_name = str_replace("sqlite:", '', $file);
if (!file_exists($file_name)) {
die('Invalid sqlite file: '.$file_name);
}
try {
parent::__construct($file);
} catch (PDOException $e) {
die($e->getMessage());
}
}
public function all($entity)
{
$sql = "SELECT * FROM $entity";
$stmt = $this->query($sql);
return $stmt->fetchAll(PDO::FETCH_OBJ);
}
public function find($entity, $value, $field = 'id')
{
$sql = "SELECT * FROM $entity WHERE $field = :value";
$stmt = $this->prepare($sql);
$stmt->bindParam(':value', $value, PDO::PARAM_STR);
$stmt->execute();
return $stmt->fetchAll(PDO::FETCH_OBJ);
}
} ?>
David Evans
10,490 PointsDavid Evans
10,490 PointsI noticed that the SQL statement inside the prepare should be:
SELECT * FROM $table WHERE $field=:value
But I still receive the error.