Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial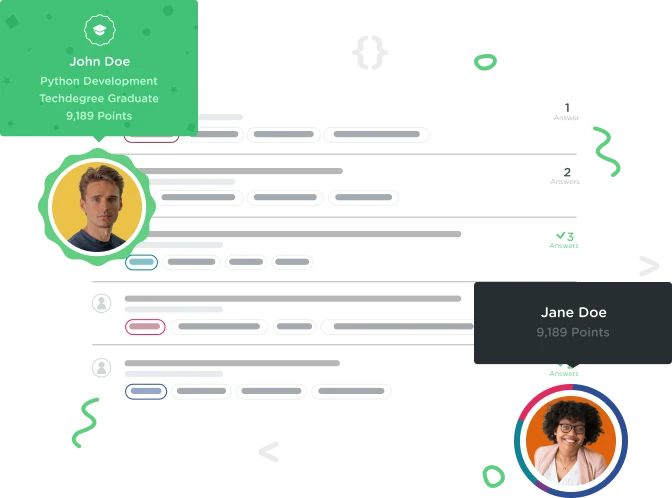

Dennis Moriarity
Courses Plus Student 940 PointsStuck on next steps
Not sure how to finish this task
# EXAMPLES
# squared(5) would return 25
# squared("2") would return 4
# squared("tim") would return "timtimtim"
def squared(num)
try:
return num ** 2:
2 Answers

Thomas Fildes
22,687 PointsHi Dennis,
This is quite a tricky challenge. Please see the code I used below to pass this challenge:
def squared(num):
try:
convertedNumber = int(num) # Try to convert your argument to an integer and store it in variable
return convertedNumber ** 2 # return variable squared (**2)
except ValueError: # except the Value Error you could receive
return num * len(num) # return the num argument multiplied by its length
Hope this helps! Happy Coding!!!
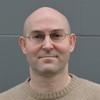
Ted Dunn
43,783 PointsIn your try block you need to take into account that the argument passed in might be a string. Remember, there is a function you can use to cast a string into an integer. You should use that function inside of the try block with the variable num as it's argument.
The second part you will need to do is to use an except clause which will "activate" if the function in the try clause throws an error (check to see what kind of error the function you called in the try block will throw) which should be included in the except condition. Inside the except clause, multiply the num variable by the length of the variable.
def squared(num):
try:
return [some function](num) ** 2
except [some kind of error]:
return num * [some other function](num)