Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial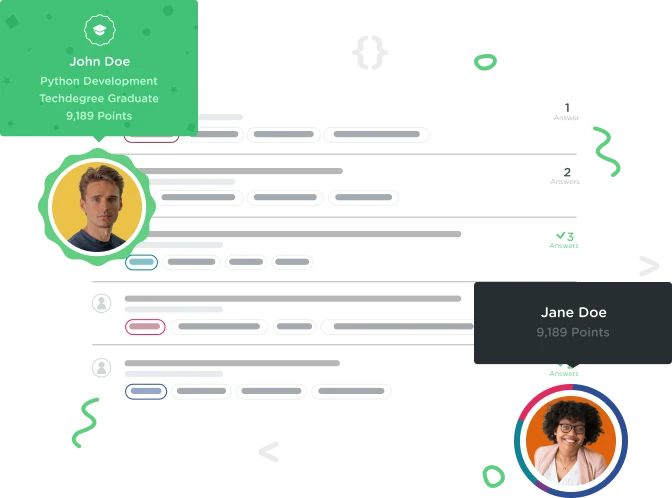

stephenallison
8,559 PointsStuck on Swift 3 Collections and Control Flow challenge task 1
Not sure what I'm doing wrong in my code.
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while sum < numbers.count {
sum++ print(numbers)
}
2 Answers
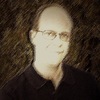
Jason Anders
Treehouse Moderator 145,860 PointsHey Stephen,
You're on the right track, but there are a few errors happening here:
- The instruction ask for a
while loop
to run as long as the counter is less then the length of the numbers array. But, your code is checking that the sum is less than the length of the numbers array. So, you will need to fix that up. - The first part of the challenge never asks you to print anything, so the print method needs to be deleted. Incidentally, if you needed to print, it would have to go on its own line anyways. You cannot combine two separate lines of code (commands) onto one line like that.
- The ++ increment operator was deprecated in Swift 2 and is no longer accepted in Swift 3, so you will need to use the += operator to increment the counter variable (again, not the sum variable).
Give it another go with those in mind. If your still stuck, leave a comment here. :)
Keep Coding!

Jorge Solana
6,064 PointsYou need to fully understand the Challenge task:
- variable "sum" is going to store the sum of all the array "numbers".
- variable "counter" is going to be our index for that array.
Clue: numbers[counter] will store each number from the array in that "counter" position (from 0 to 6 -less than 7-)
stephenallison
8,559 Pointsstephenallison
8,559 PointsI truly don't understand Swift 3 at all. And its becoming increasingly frustrating. This is my new code and I'm still receiving the error. I've corrected my while loop and its now less than the items in the array. Also, the print method has been removed.
while counter < numbers.count { counter += (numbers) }
Jason Anders
Treehouse Moderator 145,860 PointsJason Anders
Treehouse Moderator 145,860 PointsAlmost there.
I'll try and break it down for you. You've got the
while loop
started correctly, and we want it to run as long as the counter is less than the number of items in the array. This value is assigned tocounter
.There are 7 items in the array, and when we begin the loop, the
counter
value is zero, so the loop runs. We then need to add one to the counter (you were trying to add the array variable). So you would needAfter the first iteration, the counter will increment by one. Now going into the second loop, the value of the counter is one, so still less than 7 and will run again and increment by one again until it is no longer less than 7.
I understand frustration. When I first started out, many things were confusing, but with perseverance and persistence and a whack load of practice, it starts to make much more sense. Swift 3 is much like many of the other backend languages when it comes to loops, functions, arrays, etc. I see that you have been focusing on the Front-End development, so Swift will seem more difficult at first. Many people disagree, but I actually found that studying the basics of a few backend languages one after the other really helped in learning the fundamental concepts of all of them. JavaScript (when used more for Backend), Java, Ruby, Objective-C, and Swift really did help with the learning of each other one. I think it was because everyone teaches differently, so you would be learning pretty much the same concepts in a different way. Also, watching and re-watching the video played a big part too.
I'm sure it will come. If it doesn't, though, don't get discouraged... there are many languages out there, and one will just 'click'. For me, it's Java and Ruby, while Python and myself do not 'get along' at all.
Good Luck, and I hope you find that "Ah-ha!" moment soon! :)
stephenallison
8,559 Pointsstephenallison
8,559 PointsThank you Jason for the encouragement and support. I'm working my hardest to understand this language but its just not clicking. I will not stop practicing at all. But it's encouraging to see that seasoned professional as yourself not get along with a language. I was beginning to think it was just me.