Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial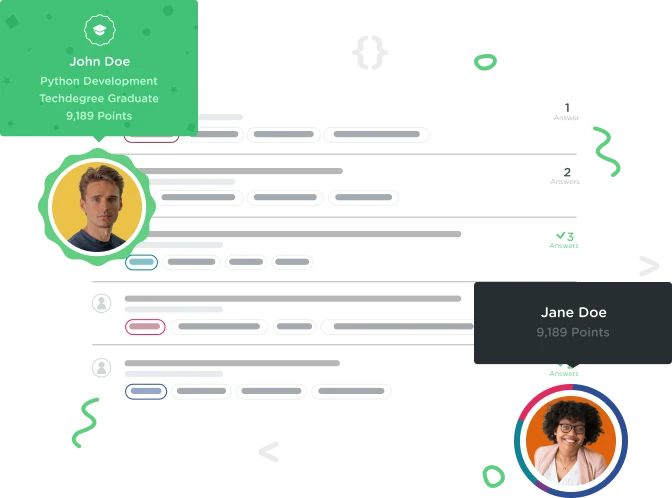
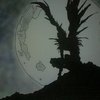
Lucas Santos
19,315 PointsStuck on this javascript loop
Use a for or while loop to iterate through the values in the temperatures array from the first item -- 100 -- to the last -- 10. Inside the loop, log the current array value to the console.
var temperatures = [100,90,99,80,70,65,30,10];
temperatures.sort(function(a, b){return a-b});
for( i = 0; i > temperatures.length; i++){
console.log( temperatures[i] );
}
tried my best and could of sworn I got this correct because iv done this before.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
16 Answers
William Li
Courses Plus Student 26,868 PointsYou don't need to sort the items in the Array, so delete this line temperatures.sort(function(a, b){return a-b});
just use the for loop to log out each item
for (var i = 0; i < temperatures.length; i++) {
console.log(temperatures[i]);
}

James Spence
17,563 PointsCheck the condition in your for loop ' i > temperatures.length '
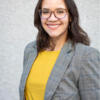
Katiuska Alicea de Leon
10,341 PointsYou need "temperatures" in console.log(temperatures[i]); because it is the name of the array.

Samuel Daw
Front End Web Development Techdegree Graduate 16,525 PointsTry this:
var temperatures = [100,90,99,80,70,65,30,10];
for ( var i = 0; i < temperatures.length; i += 1) {
console.log(temperatures[i]);
}
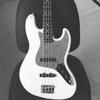
Lee Puckett
12,539 Pointstoo bad the they don't actually teach you how to do the challenge in the video

Rose M
3,156 Pointsfacts!
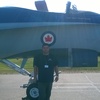
Arturo Espinoza
9,181 PointsIs +=1 same as i++ ? I don't recall this being in any of the lessons.

Kenny Jeurissen
3,894 PointsYes, it is. It's not in any of the lessons, I found it out while doing CodeCademy's Javascript course.
Fernando Guevara
6,172 Pointsyes the same happend to me with (++) pass but with (+=) gives me an error, i don't understand this if both are supposed to be the same
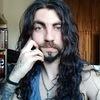
Emil Pesaxov
3,684 PointsYes, it is.
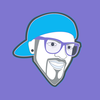
CJ Williams
34,372 Pointswon't work with a semi-colon after the for loop.
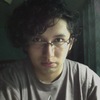
JJ RomMar
9,906 Pointsvar temperatures = [100,90,99,80,70,65,30,10]; for (i = 0; i < temperatures[7]; i+= 1) { // Can use: for (i = 0; i < temperatures.lenght; i++) console.log(temperatures[i]); // but it doesn't run. }

Adarsh Prabhu
15,394 PointsYou have a typo...
should be
temperatures.length
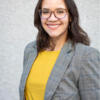
Katiuska Alicea de Leon
10,341 PointsI had a typo on length too, that's why it wasn't running! Lol
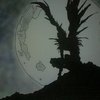
Lucas Santos
19,315 Pointshmm I guess I was rite I just passed by adding var before the i in my for loop. Don't get why you need the var tho, iv been writing my javascript for loops for a long time without the var. Believe javascript already knows that the first thing in the for has to be a variable.
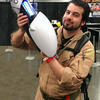
John Grillo
30,241 Pointsthat's the answer; is should be i < temperatures.length also, a var i = 0 at the beginning wouldn't hurt.
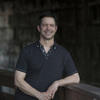
Tyler _
6,651 Points????

Riza Barone
4,973 Pointswhat does temperature.length mean in this for loop? I am having a hard time understanding it...
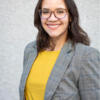
Katiuska Alicea de Leon
10,341 PointsAs I understand it temperature.length takes the place of the number you would have put there as the number of times the loop needs to run. Since items can be added or subtracted from an array at any time, for any reason, without you being there all the time to make sure the loop runs for the number of items in the array, you place the .length property, which will ALWAYS have the number of items in the array, hence the number of times the loop has to run.
Ryan Roybal
4,682 PointsThis may be a stupid question but does the [i] variable only count for that loop?
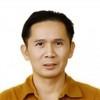
Bao Tran
Front End Web Development Techdegree Student 18,959 PointsRyan Roybal , Not sure if this answer your question but may also help others. It is not really a count.
i itself is just a makeup shorthand variable, like i or j from previous lessons. What I've found very interesting is when in JavaScript, if we set the " var i = 0 " , now that happen to match up exactly as the array index value.
So when we do " console.log(temperature[i]) " , it print out all the value from the array , in this case 0,1,2,3,4,5,6,7 because of the for loop " for (var i = 0; i < temperatures.length; i += 1) "
Short answer [i] here in this problem is the stored values from the for loop.
Hope this help.

Ousama Berrag
426 PointsWhat did I do wrong
var temperatures = [100,90,99,80,70,65,30,10];
for ( var i = 0; i < temperatures.length; i += 1; ) {
console.log(temperatures[i]);
}

Koosha Modabbernia
7,098 PointsI think there shouldn't be ";" after the i += 1
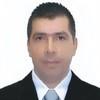
Omar Ocampo
3,166 PointsWow. Again, the videos, not the teachers (because you call teachers when you get answers to your questions/inquiries and I'm stuck in Laravel, I never received the support), never teach too much, the challenges are to make you learn how bad trained are you in this, anyway, I got the correct answer to this challenge and the response below
var temperatures = [100,90,99,80,70,65,30,10]; for ( var i = 0; i < temperatures.length; i += 1) { console.log(temperatures[i]); }

sofia Heikkila Svensson
3,695 PointsHi,
I´m getting the code wrong :
for (var i = 0;i < temperatures.length; i++);{ console.log(temperatures[i]); }
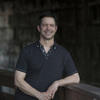
Tyler _
6,651 PointsI believe this will work too:
var temperatures = [100,90,99,80,70,65,30,10];
for (i = 0; temperatures <= [7]; i += 1) {
console.log(temperatures[i]);
}
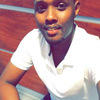
Abdifatah Mohamed
5,289 PointsHow can I reply like this blackboard please?
Lucas Santos
19,315 PointsLucas Santos
19,315 PointsYeah I was just missing the var before the i. Thanks
Tage Smith
2,997 PointsTage Smith
2,997 PointsWhat is the significance of the "++" behind the 'i' in that code?
William Li
Courses Plus Student 26,868 PointsWilliam Li
Courses Plus Student 26,868 Points++ in JavaScript, as well as many other programming languages, is an increment operator,
i++
means increment i by 1, it's a shorthand fori = i + 1
.Trung Nguyen
4,844 PointsTrung Nguyen
4,844 PointsHello, I did console.log([i]); and its wrong. Could you tell me why you need temperatures[i]?
Omar Ocampo
3,166 PointsOmar Ocampo
3,166 PointsThanks, this was the best answer I found.