Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial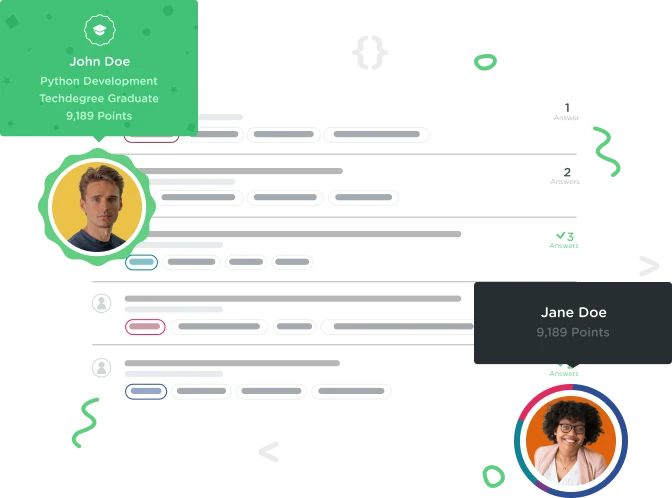
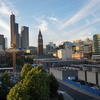
Samson Chemir
6,604 Pointsstuck on this question. else if ( today === 'Friday' && money < 9 ) { alert("It's Friday, but I don't have...
var money = 9;
var today = 'Friday'
if ( money >= 100 || today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 || today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 || today === 'Friday' ) {
alert("Time for a movie");
} else if ( today === 'Friday' && money < 9 ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers

Antonio De Rose
20,885 Pointstry change only the operators, do not add any additional conditions.
question mentions, to consider both the value and money, the moment question, mentions as such, there you go, you have to change 3 operators listed above.
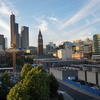
Samson Chemir
6,604 PointsThank You Ethan Miller and others for the guidance!
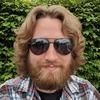
Ethan Miller
14,398 PointsHappy to help! :)
Ethan Miller
14,398 PointsEthan Miller
14,398 PointsIt looks like setting var today as Friday is triggering the first response "Time to go to the theater" because your conditional operator is the 'or' operator. Your first line of code runs, and it ignores all of the following else if cases.
Assuming you're trying to have the code bring up the alert text "It's Friday, but I don't have enough money to go out", I would say you should do two things:
1) Change all || operators to && so that both conditions MUST be true. Given that Friday is present in everything but the final else statement, the value of the var money seems to be the contingent factor.
2) You should change the fourth conditional statement to reflect money being anything less than 10. The way it stands currently, having money set to 9 will trigger the else statement, since money only needing to be less than 9 does not account for it being equal to 9.
The following code will alert "It's Friday, but I don't have enough money to go out"