Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial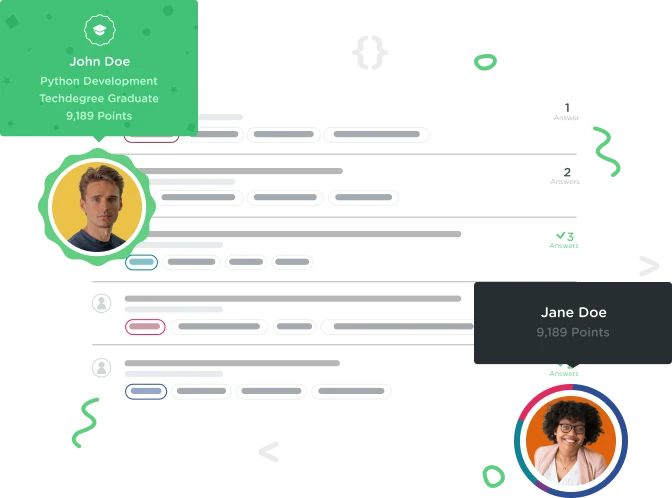
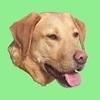
alastair cooper
30,617 PointsStudent Records Search Extension Challenge - My Solution
Big thanks to Treehouse and Dave McFarland for the excellent javascript lessons. I took on the extension challenge at the end of the objects course and here is my solution:
In the index.html file, I gave the h1 element above the output div an id of 'outputHeading'. Otherwise, it is unchanged.
In the students.js file, I added a load more students to the array with different data but some names matching.
The student_report.js file is below
// Prints message to div (id=output)
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
// Prints message to div (id=outputHeading)
function printHeading(message) {
var outputDiv = document.getElementById('outputHeading');
outputDiv.innerHTML = message;
}
// Accepts student object and
// returns formatted string of
// student data with html mark-up
function getStudData(stud) {
var mess = '<h2>Student: ' + stud.name + '</h2>';
mess += '<p>Track: ' + stud.track + '</p>';
mess += '<p>Points: ' + stud.points + '</p>';
mess += '<p>Achievements: ' + stud.achievements + '</p>';
return mess;
}
var message = '';
var student;
var search;
var toldToQuit = false;
while (toldToQuit === false) {
search = prompt('Enter the name of the student you wish to view.\nOr type "quit" to exit.');
if (search === null || search === '') {
printHeading(Students);
print('Please enter a name to search');
} else {
if (search.toLowerCase() === 'quit') {
toldToQuit = true;
} else {
var studsFound = [];
var found = false;
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name.toLowerCase() === search.toLowerCase()) {
found = true
studsFound.push(student);
}
}
if (found === false) {
message = 'Sorry, we have no-one called ' + search;
printHeading(message);
print('');
} else {
printHeading('There are ' + studsFound.length + ' students called ' + search);
message = '';
for (j = 0; j < studsFound.length; j += 1) {
message += getStudData(studsFound[j]);
}
print(message);
}
}
}
}
3 Answers

Bert-Jan van den Brink
2,309 PointsGood work Alastair
i see you used 2 different functions, to do the same thing twice. You output a message to two different divs. using the functions print and printHeading. I came up with a more DRY solutions. Just wanted to let you know:
// Prints message to div
function print(id , message) {
var outputDiv = document.getElementById('id');
id.innerHTML = message;
}
var randomMessage = '<p> just some random message </p>';
print(print, randomMessage);
print(printheading, randomMessage);
This way you don't have to write another function each time you want to write something to a div with another id.
Let me know your thoughts!
Kind regards, Bert-Jan

Chris Burbage
Courses Plus Student 4,693 PointsHi Bert-Jan Should the "id.innerHTML = message;" be "outputDiv.innerHTML = message" instead? regards CJ

Bert-Jan van den Brink
2,309 PointsHi Chris, you made me thinking and i can actually be more dry!
// Prints message to div
function print(id , message) {
var outputDiv = id.innerHTML = message;
}
var randomMessage = '<p> just some random message </p>';
print(print1, randomMessage);
print(printheading, randomMessage);
Thanks for commenting!

Andrii Kodola
Full Stack JavaScript Techdegree Student 16,870 PointsWouldn't it be better to change variable toldToQuit on just break; statement?
Also you won't let user cancel entering name by thouse lines if (search === null || search === '') { printHeading(Students); print('Please enter a name to search');