Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial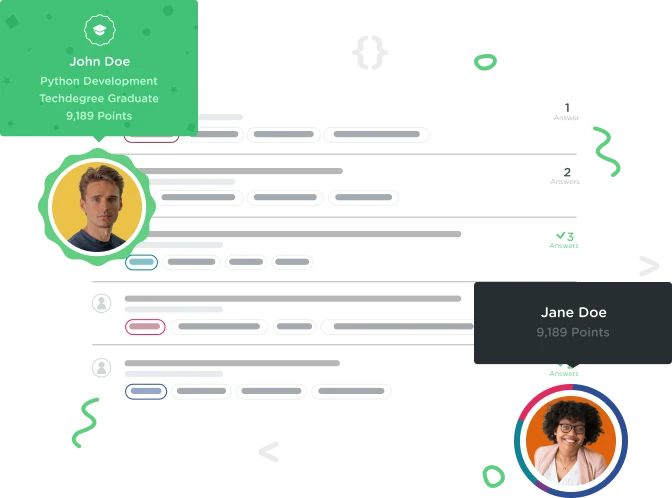
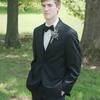
Jamison Imhoff
12,460 PointsStumped Code Challenge DICT
Write a function named courses that takes the dictionary of teachers. It should return a list of all of the courses offered by all of the teachers.
Having issues with final part of code challenge. Not sure how to get the list to give each of the courses. I keep getting "5 but expeted 18" error. I think my code is grouping ie [1,2] [3,4] [5,6] and instead of that count being 6, is 3 (groups of 2). Not sure how to get it to work as intended.
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
# Your code goes below here.
def most_classes(test):
max_count = 0
busy_teacher = ''
for teacher1,teacher2 in test.items():
if len(teacher2) > max_count:
max_count = len(teacher2)
busy_teacher = teacher1
return busy_teacher
def num_teachers(test2):
return len(test2)
def stats(test3):
new_list = []
for key in test3:
holder = []
holder.append(key)
holder.append(len(test3[key]))
new_list.append(holder)
return new_list
def courses(test4):
output = []
for key in test4:
output = test4.values()
return output
3 Answers
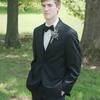
Jamison Imhoff
12,460 PointsFINALLY! solved it:
def courses(test4): #define function
output = [] # output empty
for total_course in test4.values(): #for values in test4 do the following
for course in total_course: #for values in total_course do the following
output.append(course) #add the list of values to the blank variable of output
return output #return the updated output variable
took some tinkering and searching through reading, but it passed the challenge
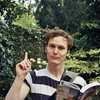
Bart Bruneel
27,212 PointsHello Jamison,
Putting a list function around test4.values, your code returns a list of lists. I think a double for-loop suits the function better. First, you need to iterate over the keys in the dictionary and then in this for-loop, you'll will need to loop over the items in the value (dict[key]), which you can then append to the output list.
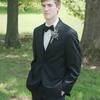
Jamison Imhoff
12,460 Pointscould you possibly provide a type of example? I am new to coding so trying to filter through the jargon can be a bit overwhelming at times. I tend to understand better with examples shown and then explained why each step is done that way. If that makes any sense....
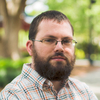
Kenneth Love
Treehouse Guest TeacherThis is where you're going to want to use the .extend()
method. test4[key]
will get you the values of the key which you can then add onto your output
variable.
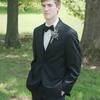
Jamison Imhoff
12,460 PointsI tried this but I am still not sure what to do. I've had a hard time with understanding this whole dict section when it comes to using it practically. I understand the concepts, and everything makes sense when I follow along in the workspace, but when I try to get it to function as I want, I can't get it to work.
def courses(test4):
output = []
for key in test4:
output = test4[key]
output.extend(test4)
return output
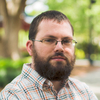
Kenneth Love
Treehouse Guest TeacherLet's look at what you've got.
def courses(test4): # We're calling the dict that comes in "test4".
output = [] # We make a blank list named "output"
for key in test4: # We loop over the keys in "test4" and call each one "key"
output = test4[key] # We change "output" to be the value of the key in "test4"
output.extend(test4) # We extend "output" with the entire "test4" dictionary. This will blow up if "output" isn't a list
return output # We return our "output" object
Hopefully the above shows you a couple of mistakes you've made already before I point them out.
Ready? OK, here we go.
Everything is good until you get into your for
loop. The for
loop is good but inside, we're blowing away our output
variable. Now it's going to be the content of the key. We don't want it to be that, we want output
to stay nice and whole.
Then, on the next line, we're trying to .extend()
output. But we're extending it with a dictionary. This'll work but not the way you're expecting. Let's look at this:
>>> a = {'a': 1}
>>> b = [2, 3]
>>> b.extend(a)
>>> b
[2, 3, 'a']
So that won't give us what we want. We want to use .extend()
on output
, but we want to extend output
with the the contents of the key. We get that with test4[key]
. So that line should be output.extend(test4[key])
.
The return
is fine, too.
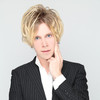
Mikael Enarsson
7,056 PointsIsn't there going to be an issue here with .extend() concatenating the list and the dict regardless of whether a course is already in the list or not?
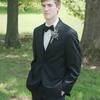
Jamison Imhoff
12,460 PointsI actually tried that as well and it did not work. So I tried again once you suggested that and it only returned 8 (not 15) also, if i recheck, it returns only 4 so it changes between 4 or 8 each time I check it which seems peculiar.
def courses(test4):
output = []
for key in test4:
output = test4[key]
output.extend(test4[key])
return output
I am really wanting to move on to the next section, but I just can't seem to figure out this last challenge =/
Kenneth Love
Treehouse Guest TeacherKenneth Love
Treehouse Guest TeacherCongrats on getting it. If you look at your last submission, it's 100% correct with just one unnecessary line (the first one inside of your
for
loop).