Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial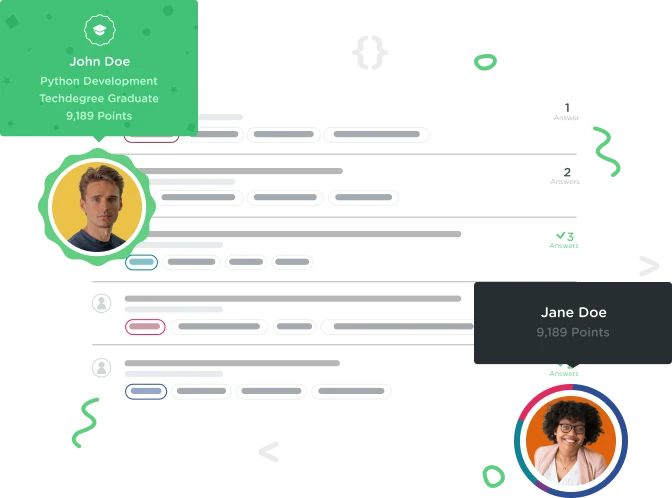

Enemuo Felix
1,895 PointsSuper conditional challenge
I'm a little bit confused here. Anybody care to help out?
var money = 9;
var today = 'Friday'
if ( money >= 100 || today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 || today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 || today === 'Friday' ) {
alert("Time for a movie");
} else if ( today !== 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers
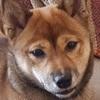
Katie Wood
19,141 PointsHey there,
This challenge is basically asking you to fix these conditional statements so that it will correctly test the day AND the amount of money. The main issue is that the current script is using OR logic (||) where it should use AND logic (&&). Using &&, it should test that it is Friday AND there is enough money to do the various activities - the way it is now, it will always print the first message, because with OR logic it just needs one of the conditions to be satisfied. Since the day is set to Friday, it will always be true - it needs to use AND logic so that it will require it to be Friday and for there to be enough money. The statements inside the last two conditions also need to be switched - the one that says it's Friday but there isn't enough money starts out inside the condition that checks to see if it isn't Friday.
The code should end up looking something like this:
var money = 9;
var today = 'Friday'
if ( money >= 100 && today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 && today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 && today === 'Friday' ) {
alert("Time for a movie");
} else if ( today !== 'Friday' ) {
alert("This isn't Friday. I need to stay home.");
} else {
alert("It's Friday, but I don't have enough money to go out");
}
Does that make sense?

Enemuo Felix
1,895 PointsThanks, this helped a lot