Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial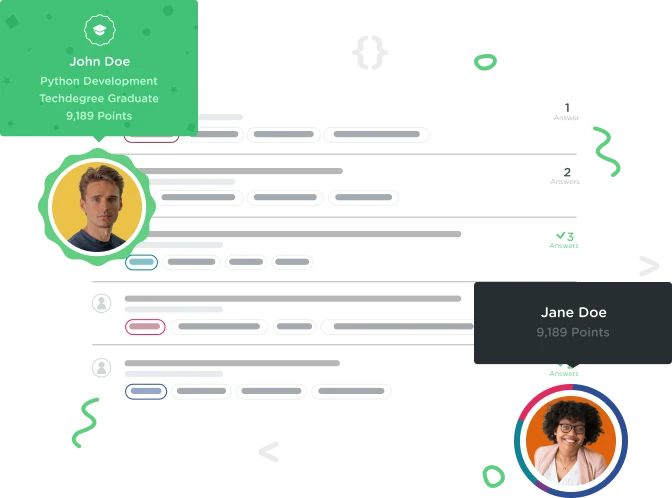

Brandon Walker
1,621 PointsTake a peak at my code?
Let me know what you all think! Not gunna lie, I'm sort of super proud of myself (completely new to coding)
function print(message) {
document.write(message);
}
function toAList(list){
var htmlChange = '<ol>';
for (x=0; x < list.length; x++){
htmlChange += "<li>" + list[x][0] + "</li>";
}
htmlChange += '</ol>'
print(htmlChange);
}
var score = 0;
var quiz=[
["What is 2 + 3?", 5],
["What is 3 - 1?", 2],
["What is 8 - 2?", 6]
]
var correct = [];
var wrong = [];
for (i=0; i < quiz.length; i++){
var userAnswer = parseInt(prompt(quiz[i][0]));
if (userAnswer === quiz[i][1]){
correct[correct.length] = quiz[i];
score +=1;
}else{
wrong[wrong.length] = quiz[i];
score -=1;
}
}
print("You got " + correct.length + " question(s) correctly!<br>");
print("Your final score is " + score + "<br>");
print("<b>These are the questions you got correct:</b>")
toAList(correct);
print("<b>These are the questions you got wrong:</b>");
toAList(wrong);
3 Answers
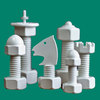
Steven Parker
230,995 PointsFor a new programmer, having your code run without errors and successfully accomplish the task is always an occasion to reflect on with pride.
Here's a gold star for you!
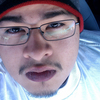
Chyno Deluxe
16,936 PointsGreat job on your coding. It is D.R.Y. and almost error free. Because your code is not using the *"use strict" * statement; errors are ignored. but once you invoke the statement then your code fails to run.
Here are a few things to change.
- move all variables to the top.
- declare x and i variable
- (optional) change correct[correct.length] = quiz[i] to correct.push(quiz[i])
var score = 0;
var quiz = [
["What is 2 + 3?", 5],
["What is 3 - 1?", 2],
["What is 8 - 2?", 6]
]
var correct = [];
var wrong = [];
function print(message) {
document.write(message);
}
function toAList(list) {
var htmlChange = '<ol>';
for (var x = 0; x < list.length; x++) {
htmlChange += "<li>" + list[x][0] + "</li>";
}
htmlChange += '</ol>'
print(htmlChange);
}
for (var i = 0; i < quiz.length; i++) {
var userAnswer = parseInt(prompt(quiz[i][0]));
if (userAnswer === quiz[i][1]) {
correct.push(quiz[i]);
score += 1;
} else {
wrong.push(quiz[i]);
score -= 1;
}
}
console.log(score);
print("You got " + correct.length + " question(s) correctly!<br>");
print("Your final score is " + score + "<br>");
print("<b>These are the questions you got correct:</b>")
toAList(correct);
print("<b>These are the questions you got wrong:</b>");
toAList(wrong);
I hope this helps. Keep Coding.

Brandon Walker
1,621 PointsThanks for the response! I have a question if that is ok? I thought about using correct.push(quiz[i]) but I was unsure if it would remove the value from the quiz array and then when the code went through the next loop it would skip to the wrong index. What I mean is:
First run though, pushes quiz[0], i is now = 1, next run through what use to be quiz [1] is now quiz[0] so when it goes to push quiz [1] it is actually pushing the old quiz[2].
Is this not the case? Does it leave the value in quiz[] and push a "copy" into correct[]? Sorry I'm completely new to this and some things are not apparent right off the bat!
Also! Are my global variables not at the top? I thought it was best practice to declare variables used within loops only inside the loops so that its local... or is that functions only? Are variables declared inside of loops also global?
Last question, is "use strict" considered best practice? I actually have not come across 'use strict' in my studies yet so I'm not exactly sure what this is (i'll read on it though!) What errors am I missing? Thanks for answering all of these questions, it only makes me better!
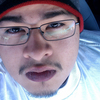
Chyno Deluxe
16,936 PointsHey Brandon,
RE: Push[i]
So what is happening when you push(quiz[i]) you are creating an entirely new array, a copy, into either correct or wrong array. After the user has completed the quiz. The arrays within correct and wrong are then sent to the toAList() method, which iterates through each and only prints out the question (i.e **quiz[x][0]) leaving the value behind but not deleting it.
RE: Global Variables
Your variables where originally below your functions, which is fine for the size of this project but can become troublesome as the project grows and if you were wanting to access a global variable within your functions, you would be unable to do so because your functions were searching for a variable which has not yet been declared.
RE: Local Variables
Yes, variables declared inside of a loop are local variables and not accessible outside of its scope, like a variable inside of functions.
RE: use strict
This is something that you will learn later as you move forward. It is best practice to use this statement in your projects as it is limited to strict programming guidelines and will not function if errors occur.
For example, you original code when I added the "use strict" failed to run because the variable i was not declared. This error was referring to the i in your for loop.
Once i was declared, by adding var in front, The quiz began prompting the questions. BUT, it later failed when it reached the toAList() method stating that x was not declared. So when declared and run again, the quiz was able to run completely.
I hope this helps and feel free to continue asking me questions if you are still confused.
Brandon Walker
1,621 PointsBrandon Walker
1,621 PointsIts been awhile since I've had this feeling of accomplishment! Motivates me to continue on with my career change to hopefully become a dev! :] Thanks