Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial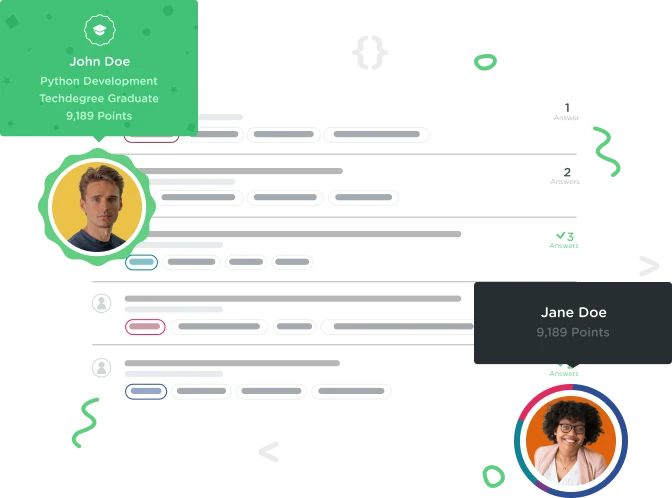

akhter ali
15,778 PointsTask 1 no longer passing....
This is my current code and I'm working on the last function "stats"
The question states that I need to return a list of lists meaning [[]], so this should be appropriate. I wish I knew what the expected output is supposed to be.
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
def most_classes(some_dict):
teacher = ''
classes = 0
for key in some_dict:
if len(some_dict[key]) > classes:
classes = int(len(some_dict[key]))
teacher = key
continue
return teacher
def num_teachers(some_dict):
return len(some_dict)
def stats(some_dict):
new_list = []
for key in some_dict:
new_list.append([key, len(some_dict[key])])
return new_list
I've tested this out on the IDE as well.
>>> test_dict
{'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'], 'Kenneth Love': ['Python Basics', 'Python Collections']}
>>> def stats(some_dict):
... new_list = []
... for key in some_dict:
... new_list.append([key, len(some_dict[key])])
... return new_list
...
>>> stats(test_dict)
[['Jason Seifer', 3], ['Kenneth Love', 2]]
>>>
3 Answers
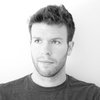
Matthew Rigdon
8,223 PointsI will give you a few hints. First, you want to make sure you return this:
def num_teachers(some_dict):
return int(len(some_dict))
My code was saying that code 1 was failing unless I returned the length of some_dict as an integer. Next, your other issue is in your stats portion. Your answer should be printed like this:
[['Jason Seifer', 3], ['Kenneth Love', 2]]
The answer is two separate lists inside of a list. See if you can figure it out now. If not, let me know and I will assist you more.

akhter ali
15,778 Points@Matthew Rigdon UPDATED
Thanks. One issue was def num_teachers(some_dict): return len(some_dict)
On stats method, I do get the same output as you got, however, I still get "task 1 no longer passing"
My new code is:
def most_classes(some_dict):
teacher = ''
classes = 0
for key in some_dict:
if len(some_dict[key]) > classes:
classes = int(len(some_dict[key]))
teacher = key
continue
return teacher
def num_teachers(some_dict):
return int(len(some_dict))
def stats(some_dict):
new_list = []
for key in some_dict:
new_list.append([key, len(some_dict[key])])
return new_list
Please do let me know what I'm doing wrong here, as it does work correctly in the IDE.

akhter ali
15,778 PointsAt the end this worked.
def most_classes(some_dict):
teachers = ''
classes = 0
for key in some_dict:
if len(some_dict[key]) > classes:
classes = int(len(some_dict[key]))
teacher = key
continue
return teacher
def num_teachers(some_dict):
return len(some_dict)
def stats(some_dict):
new_list = []
for key in some_dict:
new_list.append([key, len(some_dict[key])])
return new_list