Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial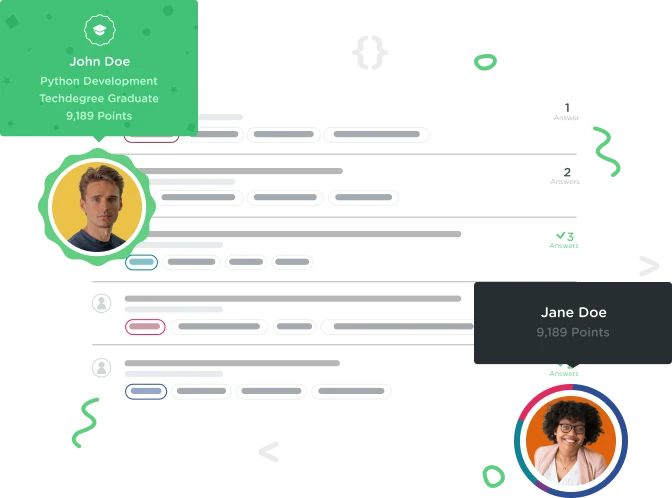

Waleed Aljarman
Courses Plus Student 947 Pointsteacher dict
i gave up on the last one, how i can do it ?
def num_teachers(t_dict):
return len(t_dict)
def num_courses(t_dict):
courses=0
for value in t_dict.values():
courses += len(value)
return courses
def courses (t_dict):
c_list=[]
for value in t_dict.values():
c_list.append(value)
other=[]
for i in c_list:
other.extend(i)
return other
def most_courses(t_dict):
most_course = 0
newTeacher = ""
for teacher in t_dict:
if len(t_dict[teacher]) > most_course:
most_course = len(t_dict[teacher])
newTeacher = teacher
return newTeacher
def stats(t_dict):
2 Answers
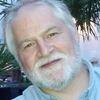
Jeff Muday
Treehouse Moderator 28,720 PointsYou got pretty far in that challenge. The solution to the challenge is to start a new empty "stats" list. Then you iterate over the "items" in each dictionary entry as a key/value pair. Finally, append the stats list with a list of just the teacher name and the class count.
Here is an example... it is not the solution, but you can use a similar approach you can use to solve the last part of the challenge.
Suppose we had a list of artists and vinyl albums-- and wanted a list of lists with the artist and the number of albums in your collection.
my_music = {'Beatles': ['Hard Days Night','Help','Abbey Road'], 'Pink Floyd': ['Dark Side of the Moon','The Wall']}
def music_stats(music_dict):
artist_stats = []
# the for loop iterates over the key/value pairs (items) in the list.
for artist, albums in music_dict.items():
artist_stats.append([artist, len(albums)]
return artist_stats
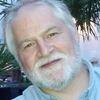
Jeff Muday
Treehouse Moderator 28,720 PointsThe impressive feature of the iterator music_dict.items() is that it returns key-value "pairs". Each time the key is matched with the value it holds.
For example
for key, value in music_dict.items():
print 'key = ', key
print 'value = ', value
The first time it goes through--
key = 'Beatles' value = ['Hard Days Night','Help','Abbey Road']
The second time it goes through
key = 'Pink Floyd' value = ['Dark Side of the Moon','The Wall']
We can use this feature to open the list of teachers and their associated courses
# this works in Python 2.7.x, you should use different syntax in Python 3.x for print statements
t_dict = {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'], 'Kenneth Love': ['Python Basics', 'Python Collections']}
for name, classes in t_dict.items():
print 'name = ', name
print 'classes = ', classes
count = len(classes)
print "{} teaches {} classes".format(name,count)
You can encapsulate this approach into the function "stats()"
def stats(t_dict):
stats_list = []
for name, classes in t_dict.items():
stats_list.append([name,len(classes)])
return stats_list
Waleed Aljarman
Courses Plus Student 947 PointsWaleed Aljarman
Courses Plus Student 947 Pointsi didn't understand what you did in the for loop statement, what does artist, mean. and just for clarification, is my_music the same as music.dict ?