Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial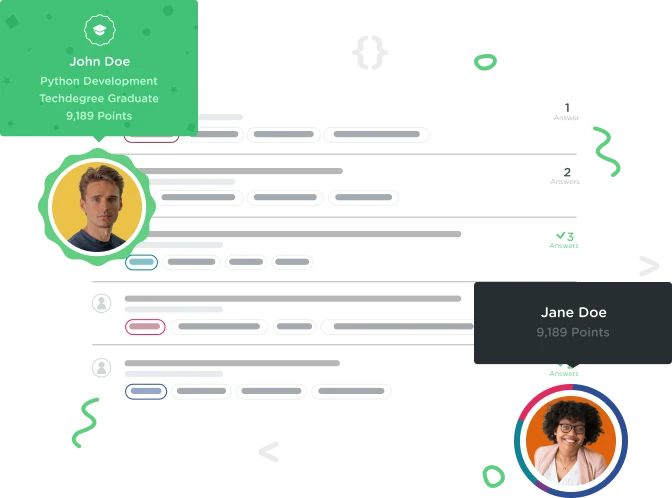

Atidaishe Chinodakufa
2,323 Pointsteacher stats challenge
help! I'm stuck here.
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
def most_classes(dicts):
count = []
maxclas=0
for teacher in dicts:
count.append(len(dicts[teacher]))
for num in count:
if num > maxclas:
maxclas = count
return teacher in maxclas==len(dicts[teacher])
13 Answers
William Li
Courses Plus Student 26,868 PointsThere're some syntax errors in your code, also, I don't 100% follow the logic of your code. But there's an obvious problem, this question is asking you to return the name of the teacher with most classes, and in your for loop, for teacher in dicts:
, you only append the number of classes each teacher has into the count list, however, your code didn't keep track of the teacher's name, which is what the return value of this function is supposed to be.
def most_classes(dicts):
most_class = "" # holds the name of teach with most class
max_count = 0 # max counter for classes
for teacher in dicts:
if len(dicts[teacher]) > max_count:
max_count = len(dicts[teacher])
most_class = teacher
return most_class
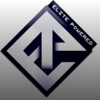
Abenezer Mamo
5,821 PointsThis is my solution to this problem. It's quite simple to understand and it's efficient.
def most_classes(my_dict):
teachers_list = {}
for teacher in my_dict:
teachers_list[teacher] = len(my_dict[teacher])
return max(teachers_list, key=teachers_list.get)
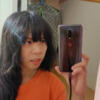
Name:GoogleSearch orJonathan Sum
5,039 Pointsexcuse me, what is return max?

Shadow Skillz
3,020 PointsHey Abenezer I've been stuck on this coding challenge for longer than I like to mention. Your solution helped me pass the second step if you could or anybody for that matter break down your code line by line in detail that would be greatly appreciated thanks.
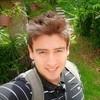
Sergio Niño
Full Stack JavaScript Techdegree Student 22,976 PointsWilliam Li gives the best answers in the world. Wow!!

fahad lashari
7,693 PointsThis is how I did it. I think this is the simplest way to achieve this task.
def most_courses(dict):
for key, value in dict.items():
if max(value):
return key

Atidaishe Chinodakufa
2,323 PointsCreate a function named most_classes that takes a dictionary of teachers and returns the teacher with the most classes.

Atidaishe Chinodakufa
2,323 PointsOk I got it. Thanks!

Trevor Currie
9,289 PointsYour return statement of
python return teacher in maxclas==len(dicts[teacher])
will not search through the dicts
for the teacher
key that has the same length as the your maxclas
variable. In order to do so, you would have to do a third for
loop for this to work:
[most_classes_teacher for teacher in dict if len(dict[teacher]) == maxclas]
Not very efficient!

Jose Vicente Jonas Franca
1,701 Pointsdef most_classes(teachers):
max_count = 0
most_class = ''
for teacher in teachers:
if len(teachers.get(teacher)) > max_count:
max_count = len(teachers.get(teacher))
most_class = teacher
return(most_class)
This worked for me.
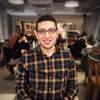
Oguz Dumanoglu
5,169 PointsHello guys,
I did this challenge in this way, but it did not worked. Pls help.
def most_classes(dict):
max_count = 0
for key in dict:
current_count = len(dict[key])
if max_count < current_count:
max_count = current_count
return key

Christopher Janke
11,054 PointsI know this post is old, but I have been stuck on this for 2 days now over thinking this problem. Thank you William for this answer. It really helped me to see that I was doing way to much code for such a simple answer. You helped to me "Refactor" my thinking!

yanyan Liu
3,954 Points <p>
def most_courses(dict):
list_comp = [ (teacher, len(courses) )for teacher, courses in dict.items()]
newlist = sorted(list_comp, key=lambda tup: tup[1])
return newlist[-1][0]
</p>

darcyprice
4,442 PointsThank you so much @William Li and @fahad Iashari!
I was able to get close on my own, but now I have figured it out :)
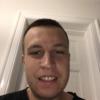
Andrii Gorokh
14,809 PointsWhy this doesn't work?
def most_courses(arg):
teacher = ""
max_count = 0
for value in arg:
if len(arg[value]) > max_count:
max_count = len(arg[value])
teacher = value
return teacher
Jan M
1,053 PointsJan M
1,053 Points@William Li could you please explain how you arrived at that answer? Why is the max count for classes 0?
Endrin Tushe
4,591 PointsEndrin Tushe
4,591 PointsCould you explain the most_class = teacher part a bit more? So I get the max count part, if the length of the values in the dictionary is greater than 0 which it will be in the first iteration then the dictionary will be whatever length of the value that it was comparing against. So let's say in the first iteration the for loop compared the length of Kenneth's love value 2 against max count. Since max count is 0 it was greater, then max count became 2. On the next iteration the loop compared the length of Jason's classes which was 3, and again since that's greater than 2 the new max_count now becomes 3. Okay so far so good...but here's where I get stuck how does the most_class=teacher, associate the value of 3 in the max_count with the key of Jason Seifer in the dictionary and then appends that key to the empty string most_class?
William Li
Courses Plus Student 26,868 PointsWilliam Li
Courses Plus Student 26,868 PointsHi, Endrin. Your understanding of max_counter is spot-on. Now, as for the most_class.
No, it doesn't append the key to empty string most_class, the equal sign is assignment operator, it assigns new value to most_class, and replaced whatever old value that's currently stored in the variable. Much like assigning new numerical value to max_count, this line
most_class = teacher
will only run when the condition if condition is satisfied.Say, using the examples you've given, while iterating through the dictionary, if the key "Kenneth Love" has 2 classes, and it's more than the 0 currently stored in max_count, 2 will be assigned to max_count, and the String "Kenneth Love" assigns to most_class; up next, Jason has 3 classes, then 3 will be assigned to max_count, and most_class becomes String "Jason Seifer".
The real question here is that, what purpose does most_class variable serves? Is it absolutely necessary to keep track of teacher's name every time the max_count gets assigned a new numerical value? The answer is YES. Because that's what the challenge requires you to do.
The challenge asked that the return value of the function to be the name of the teacher with most classes, therefore,
max_count
can't be used as return value. Does that make sense?Ryan Flyod
1,939 PointsRyan Flyod
1,939 PointsHi William,
This code doesn't seem to be accurate for me. Could you explain to me why it isn't working? Treehouse accepts it as a correct answer, but I don't think it is. Thanks. the "teacher1" key should be the answer, as it has 2 classes versus the other two keys, which have 1.
returned result is
('teacher2', 6)
indicating it grabbed the "teacher2" key because "class3" value is 6 characters long. When, in reality, it should have returned "teacher1" because it has two values, which is greater than the one value of the "teacher2" key