Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial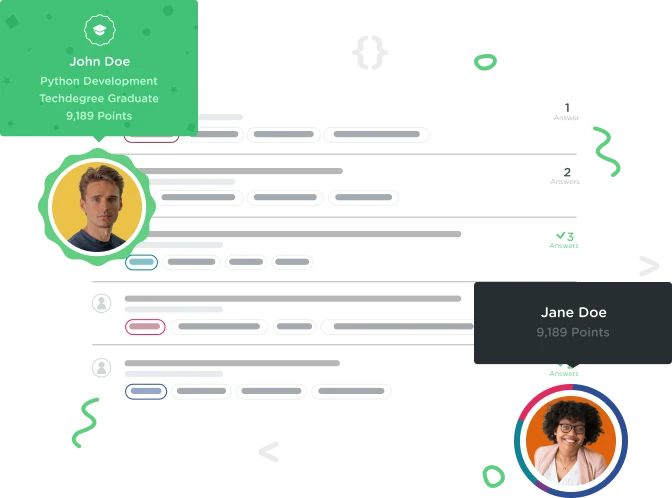

Frank Sherlotti
Courses Plus Student 1,952 PointsTeacher stats: Challenge 3 of 4. Need some help please.
Didn't get the expected output. Got ['Andrew Chalkley', ['jQuery Basics', 'Database Foundations', 'Building Social Features in Ruby on Rails', 'Build an Interactive Website']]. ---- This is my error message and it looks to me that I've changed the dictionary that I had into a list. But the problem is that it wants me to change the format of the list and I don't think I've learned that before. Was wondering if that's the problem i'm having, and if it is then could someone please explain to me how to change the formatting on a list, THANKS!
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
def most_classes(a_dict):
maxcount = 0
teacher = ''
for key, value in a_dict.items():
teacher_value = len(value)
if teacher_value > maxcount:
maxcount = teacher_value
teacher = key
return teacher
def num_teachers(a_dict):
max_count = 0
for k in a_dict:
k = max_count
if k == max_count:
max_count += 1
return max_count
def stats(a_dict):
teachers = []
a_dictlist = []
for key, value in a_dict.items():
teachers = [key, value]
a_dictlist.append(teachers)
return teachers
3 Answers
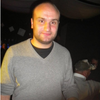
Vittorio Somaschini
33,371 PointsHello Frank.
I see 2 problems in your code:
1) inside the for loop, teachers should be a list with what you called the key, but it should not have the values (courses in our case). Instead it should get and use the number of courses, which happens to be the length of this value variable we are creating.
2) Have a close look at the return statement, you are returning the wrong thing!
I am pretty sure you can easily correct these little errors, if not let me know!
Vittorio

Frank Sherlotti
Courses Plus Student 1,952 PointsI now have this which I think I understood what you were trying to point out to me. I also believe you wanted me to get the length of value which I tried but I don't really know how to write it out properly.
def stats(a_dict):
a_dictlist = []
teachers = []
for key, value in a_dict.items():
teachers = [key], len(value)
a_dictlist.append(teachers)
return a_dictlist
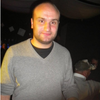
Vittorio Somaschini
33,371 PointsYou are very very close.
The only thing is that also len(value) needs to be inside the list together with "key"
After that you should be good to go
;)

Frank Sherlotti
Courses Plus Student 1,952 PointsThank you very much I really do appreciate the help!

Frank Sherlotti
Courses Plus Student 1,952 PointsQuestion: Write a function named courses that takes the dictionary of teachers. It should return a list of all of the courses offered by all of the teachers.
def courses(a_dict):
courses = []
classes = []
for key, value in a_dict.items():
classes = [len(value)]
courses.append(classes)
return courses
Could actually use some help with this as well if your still around. Shouldn't this just be like the last code I wrote and take the length of value(courses) and return it as a list? Or am just misunderstanding the question?

Iain Simmons
Treehouse Moderator 32,305 PointsIn this case, you want to get the value
for each teacher, which will be a list of courses, and then combine them all together and return that.
Note that the returned list should be only one dimension though (so, not a list of lists).