Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial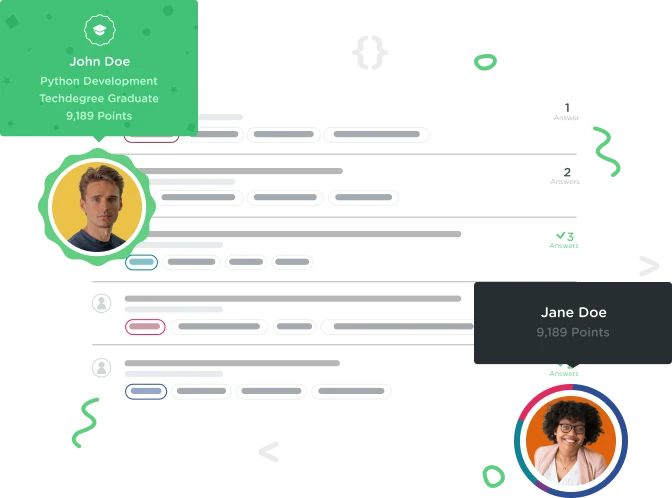

Andrew McLane
3,385 Pointsteachers.py challenge, step 2.
Trying to return the length of a list of values, but the length only counts a list within the list as one value.
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(my_dict):
return len(my_dict)
def num_courses(my_dict):
num_values = my_dict.values()
return (len(num_values))
1 Answer

Krishna Pratap Chouhan
15,203 PointsSee through the comment...
def num_courses(my_dict):
#this would return the list containing all the values in the dictionary, here its 2, as there are two key value pairs.
num_values = my_dict.values()
count = 0
for i in my_dict.values():
count = count + len(i)
return (count)
Andrew McLane
3,385 PointsAndrew McLane
3,385 PointsThis make sense, but why does the for loop see each value in the dict as a single entity, while taking the len(num_values) doesn't?
Andrew McLane
3,385 PointsAndrew McLane
3,385 PointsSo I just tried this, and for a dict such as my_dict = {"Kenneth Love" : ["Python Basics", "Python Collections"]}, the return value is only 1 instead of 2. This is the error i was getting with my code as well. A list of values only gets counted as one.
Krishna Pratap Chouhan
15,203 PointsKrishna Pratap Chouhan
15,203 PointsWell, things are modulated (divided into modules).
So... dictionary in python has elements as it's child. Each child is divided into key&value. Value again is a single entity and could contain any other element. To address this element we have to treat it like the object of whatever class it may be.
In this case, it is a list. Since the value is a single list so when you try to find the length of value its one. But if you want to find the length of object stored in the value, you will get it as 2.
Once again, the length of value of dictionary element is one but the length of value is 2.