Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial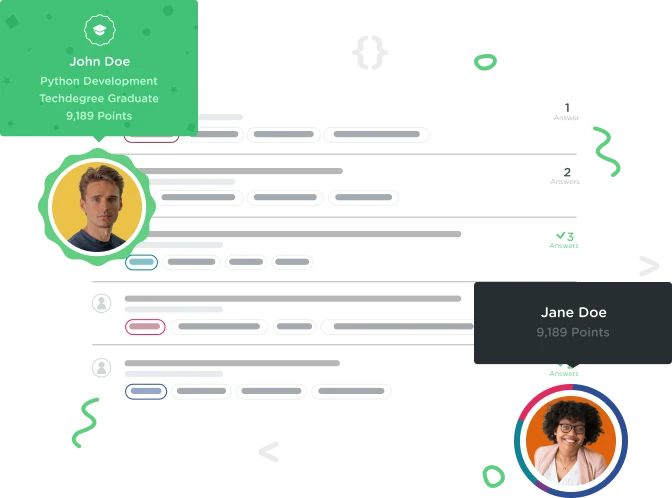

Marc Muniz
Courses Plus Student 3,315 Pointsteachers.py task 3 help!
So, I've gotten as far as making a LIST OF LISTS using the def courses function...but cannot figure out how to convert it into ONE LIST like the task is asking for. Any help would be appreciated!
```python
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
teach_dict = {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'], 'Kenneth Love': ['Python Basics', 'Python Collections']}
def num_teachers(teach_dict):
teach_count = 0
for key in teach_dict:
teach_count += 1
return teach_count
def num_courses(teach_dict):
course_count = 0
for value in teach_dict.values():
course_count += len(value)
return course_count
def courses(teach_dict):
my_list = []
for value in teach_dict.values():
my_list.append(value)
print(my_list)
2 Answers

Joshua Dam
7,148 PointsYeah, you're creating a list of lists. Like James said, you need to dig deeper into those lists to pull individual values out.
You should have at most 3 FOR statements. You can do it with just two. Let me know if you want to see how that's written out so you can study it
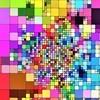
james south
Front End Web Development Techdegree Graduate 33,271 Pointsthe values in teach_dict are lists, so when you append each one, you get a list of lists. to reach into each of those lists one thing you could do is nest another for loop in your existing one, and then you would have: for list in dict, then for element in list, append element. this will place the individual elements, not the lists, in your new list.

Marc Muniz
Courses Plus Student 3,315 Pointshey thx for the response....so I've tried that, but my initial code when run in workspaces returns this:
my_list = [['jQuery Basics', 'Node.js Basics'], ['Python Basics', 'Python Collections']]
so to me that means there is only 2 elements in the list...hence why idk how to pull the 4 values out to put into a single list?
Marc Muniz
Courses Plus Student 3,315 PointsMarc Muniz
Courses Plus Student 3,315 PointsYes, please show me lol I've been stuck on this task for awhile now -_-
Joshua Dam
7,148 PointsJoshua Dam
7,148 PointsSorry, I think I was wrong. You shouldn't have 3 FOR statements. Just two.
Marc Muniz
Courses Plus Student 3,315 PointsMarc Muniz
Courses Plus Student 3,315 PointsAhhh that makes a lot of sense I see where my attempts at nesting went wrong. Thx so much!