Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial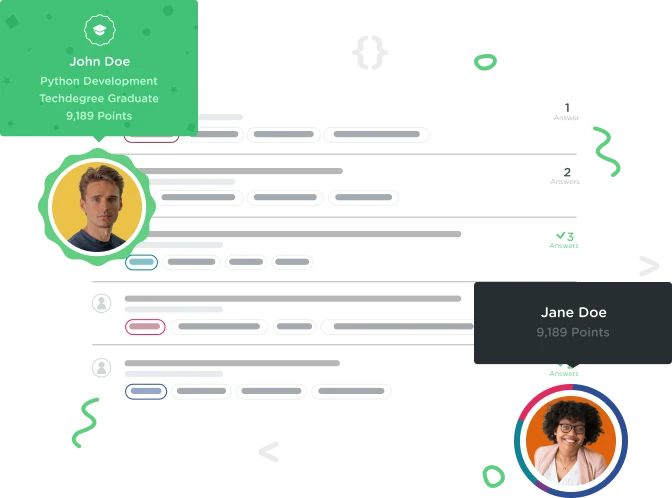

Alex Smith
5,133 PointsTemplate Literals
Trying to translate this over to template literal. Can someone please tell me what I'm doing wrong?
3 Answers
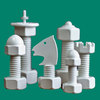
Steven Parker
241,956 PointsThanks for providing a snapshot! The code has these issues:
- stray parentheses after "rgbColor" on lines 14 and 15
- stray extra accent mark inside template literal on line 14
- missing closing parenthesis on line 14
- missing (optional) semicolon on line 5
// original:
rgbColor() = `rgb(`${randomRGB()}, ${randomRGB()}, ${randomRGB()}`;
html += ` <div style='background-color: ${rgbColor()}' ></div> `;
// corrected:
rgbColor = `rgb(${randomRGB()}, ${randomRGB()}, ${randomRGB()})`;
html += `<div style='background-color: ${rgbColor}'></div>`;
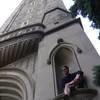
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsYes, but this still won't produce the output he's looking for. See second paragraph of my answer.
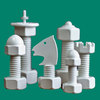
Steven Parker
241,956 PointsI tested it and it worked fine.
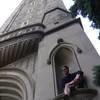
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsWhat browser? So did I with Chrome. It may depend on the browser's user agent stylesheet. At least in Chrome, without any content in the div
you merely get undefined
.

Alex Smith
5,133 PointsThank you, guys! I appreciate your help.
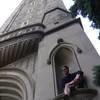
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsHi Alex, I have fixed your code so that it will work. There were a few things going on here. Firstly, here's the new code:
let html = '';
let rgbColor;
function randomRGB() {
return Math.floor(Math.random() * 256 )
}
for ( let i = 1; i <=10; i++ ) {
rgbColor = `rgb(${randomRGB()}, ${randomRGB()}, ${randomRGB()}`;
html += `<div style='background-color: ${rgbColor}'>Hello World</div>`;
}
document.write(html);
I did a minor refactor of your for
loop so it might look just a bit different. The main problem was that you were trying to call your rgbColor
variable like a function in several places. rgbColor
isn't a function. I assume this was just a simple typo. The other problem is that you had three backticks in the template literal you are assigning to rgbColor
. So these two issues would have led to an Unexpected Identifier
with the tree backticks, and rbgColor is not a function
.
However, this code still wouldn't have produced the output you expected even if there were no errors in the code. The reason is that an empty div
with no styling applied will have a height of 0. You could either add some CSS to give it a height, or add some text. I added some text into your div so that when you run it you'll see the coloured div
s appear.
Don't worry about these little errors. You'll make them less and less the longer you code, but you'll never stop making them. I've been coding for about three years now and I still occasionally waste time banging my head against my desk trying to figure out why my code won't work when it was just a typo that I didn't notice.
Good luck!
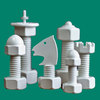
Steven Parker
241,956 PointsFully functional CSS was included in the snapshot!
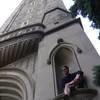
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsAh, I see. I don't like using snapshots. I usually just paste into my Chrome console so that's my bad.
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsMichael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsIf you feel like having a bit of fun with this code (and probably also crashing your browser in the process) you could wrap the whole thing in the
setInterval
API and run it continuously.