Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial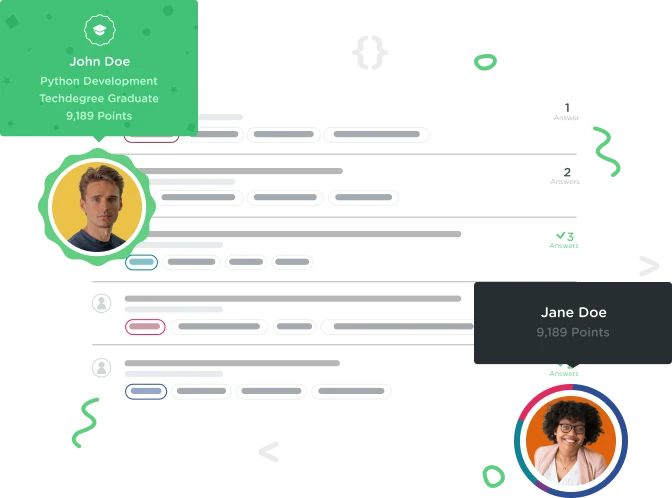

Steven Worley
3,615 PointsTest fails before and after todo_list.reload
I've triple checked everything, and can't find the problem. I apologize if I've missed something simple.
My code:
require 'spec_helper'
describe "Editing todo lists" do
it "updates a todo list successfully with correct information" do
todo_list = TodoList.create(title: "Groceries", description: "Grocery list.")
visit "/todo_lists"
within "#todo_list_#{todo_list.id}" do
click_link "Edit"
end
fill_in "Title", with: "New title"
fill_in "Description", with: "New description"
click_button "Update Todo list"
todo_list.reload
expect(page).to have_content("Todo list was successfully updated")
expect(todo_list.title).to eq("New title")
expect(todo_list.description).to eq("New description")
end
end
Test result:
Finished in 0.05977 seconds
1 example, 1 failure
Failed examples:
rspec ./spec/features/todo_lists/edit_spec.rb:4 # Editing todo lists updates a todo list successfully with correct information
Randomized with seed 45596

Steven Worley
3,615 PointsHere is the full error message from the console:
F
Failures:
1) Editing todo lists updates a todo list successfully with correct information
Failure/Error: within "#todo_list_#{todo_list.id}" do
Capybara::ElementNotFound:
Unable to find css "#todo_list_1"
# ./spec/features/todo_lists/edit_spec.rb:8:in `block (2 levels) in <top (required)>'
Deprecation Warnings:
--------------------------------------------------------------------------------
RSpec::Core::ExampleGroup#example is deprecated and will be removed
in RSpec 3. There are a few options for what you can use instead:
- rspec-core's DSL methods (`it`, `before`, `after`, `let`, `subject`, etc)
now yield the example as a block argument, and that is the recommended
way to access the current example from those contexts.
- The current example is now exposed via `RSpec.current_example`,
which is accessible from any context.
- If you can't update the code at this call site (e.g. because it is in
an extension gem), you can use this snippet to continue making this
method available in RSpec 2.99 and RSpec 3:
RSpec.configure do |c|
c.expose_current_running_example_as :example
end
(Called from /Users/Fuzz/.rbenv/versions/2.1.5/lib/ruby/gems/2.1.0/gems/capybara-2.1.0/lib/capybara/rspec.rb:20:in `block (2 levels) in <top (required)>')
--------------------------------------------------------------------------------
--------------------------------------------------------------------------------
RSpec::Core::ExampleGroup#example is deprecated and will be removed
in RSpec 3. There are a few options for what you can use instead:
- rspec-core's DSL methods (`it`, `before`, `after`, `let`, `subject`, etc)
now yield the example as a block argument, and that is the recommended
way to access the current example from those contexts.
- The current example is now exposed via `RSpec.current_example`,
which is accessible from any context.
- If you can't update the code at this call site (e.g. because it is in
an extension gem), you can use this snippet to continue making this
method available in RSpec 2.99 and RSpec 3:
RSpec.configure do |c|
c.expose_current_running_example_as :example
end
(Called from /Users/Fuzz/.rbenv/versions/2.1.5/lib/ruby/gems/2.1.0/gems/capybara-2.1.0/lib/capybara/rspec.rb:21:in `block (2 levels) in <top (required)>')
--------------------------------------------------------------------------------
If you need more of the backtrace for any of these deprecations to
identify where to make the necessary changes, you can configure
`config.raise_errors_for_deprecations!`, and it will turn the
deprecation warnings into errors, giving you the full backtrace.
2 deprecation warnings total
Finished in 0.05567 seconds
1 example, 1 failure
Failed examples:
rspec ./spec/features/todo_lists/edit_spec.rb:4 # Editing todo lists updates a todo list successfully with correct information
Randomized with seed 23358
As I am a beginner, and this is my first course in any type of development, I don't know what you mean by "You should also show the files that cause the error, i.e. appropriate views and maybe controllers as well."
I showed my code for edit_spec.rb. I thought that was the file that was causing the error?
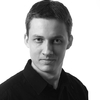
Maciej Czuchnowski
36,441 PointsOK, now we know what exactly went wrong: "Unable to find css "#todo_list_1"". This can be caused by many different factors, so we need to look at the view and controller of the model that we are dealing with here. Please paste your code from app/views/todo_lists/index.html.erb and app/controllers/todo_list_controller.rb. This will give us some ground to work with.

Steven Worley
3,615 Pointsapp/views/todo_lists/index.html.erb:
<h1>Listing todo_lists</h1>
<table>
<thead>
<tr>
<th>Title</th>
<th>Description</th>
<th colspan="3"></th>
</tr>
</thead>
<tbody>
<% @todo_lists.each do |todo_list| %>
<tr id="<%= dom_id(todo_list) %>>
<td><%= todo_list.title %></td>
<td><%= todo_list.description %></td>
<td><%= link_to 'Show', todo_list %></td>
<td><%= link_to 'Edit', edit_todo_list_path(todo_list) %></td>
<td><%= link_to 'Destroy', todo_list, method: :delete, data: { confirm: 'Are you sure?' } %></td>
</tr>
<% end %>
</tbody>
</table>
<br>
<%= link_to 'New Todo list', new_todo_list_path %>
app/controllers/todo_list_controller.rb:
class TodoListsController < ApplicationController
before_action :set_todo_list, only: [:show, :edit, :update, :destroy]
# GET /todo_lists
# GET /todo_lists.json
def index
@todo_lists = TodoList.all
end
# GET /todo_lists/1
# GET /todo_lists/1.json
def show
end
# GET /todo_lists/new
def new
@todo_list = TodoList.new
end
# GET /todo_lists/1/edit
def edit
end
# POST /todo_lists
# POST /todo_lists.json
def create
@todo_list = TodoList.new(todo_list_params)
respond_to do |format|
if @todo_list.save
format.html { redirect_to @todo_list, notice: 'Todo list was successfully created.' }
format.json { render :show, status: :created, location: @todo_list }
else
format.html { render :new }
format.json { render json: @todo_list.errors, status: :unprocessable_entity }
end
end
end
# PATCH/PUT /todo_lists/1
# PATCH/PUT /todo_lists/1.json
def update
respond_to do |format|
if @todo_list.update(todo_list_params)
format.html { redirect_to @todo_list, notice: 'Todo list was successfully updated.' }
format.json { render :show, status: :ok, location: @todo_list }
else
format.html { render :edit }
format.json { render json: @todo_list.errors, status: :unprocessable_entity }
end
end
end
# DELETE /todo_lists/1
# DELETE /todo_lists/1.json
def destroy
@todo_list.destroy
respond_to do |format|
format.html { redirect_to todo_lists_url, notice: 'Todo list was successfully destroyed.' }
format.json { head :no_content }
end
end
private
# Use callbacks to share common setup or constraints between actions.
def set_todo_list
@todo_list = TodoList.find(params[:id])
end
# Never trust parameters from the scary internet, only allow the white list through.
def todo_list_params
params.require(:todo_list).permit(:title, :description)
end
end
2 Answers
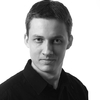
Maciej Czuchnowski
36,441 PointsAlright, this line in your view has one quote missing:
<tr id="<%= dom_id(todo_list) %>>
Change it to
<tr id="<%= dom_id(todo_list) %>">
and let me know if that helped.

Steven Worley
3,615 PointsThat fixed it. Thanks!
Maciej Czuchnowski
36,441 PointsMaciej Czuchnowski
36,441 PointsThis is not a full error message from the console, so it's impossible to pinpoint the problem. You should also show the files that cause the error, i.e. appropriate views and maybe controllers as well.