Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial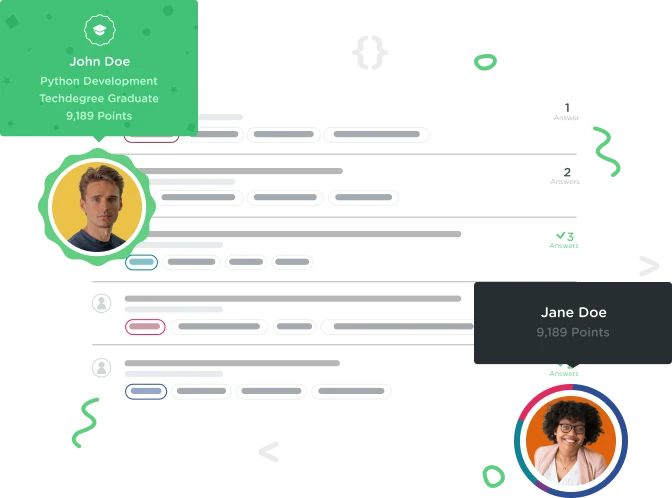

David Ton-Lai
3,268 PointsThe challenge can't find my most_courses function.
The challenge isn't able to find my function.
# The dictionary will look something like:
#{'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'], 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(dict):
return len(dict)
def num_courses(dict):
list1 = []
for value in dict.values():
list1.append(len(value))
return sum(list1)
def courses(dict):
list1 = []
for value in dict.values():
list1.extend(value)
return list1
def most_courses(dict):
count = 0
list1 = []
for value in dict.values():
list1.append(value)
for item in list1:
if len(item) > count:
count = (len(item))
for key in dict:
if count == len(dict1[key]):
return key
2 Answers
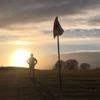
Stuart Wright
41,120 PointsI would probably have avoided the need to use a list by simply keeping a max_teacher variable for the name of the teacher who currently has the most courses, and update it as necessary throughout the loop. And once we finish the loop, return max_teacher. This method requires only one loop rather than three:
def most_courses(teachers_info):
max_count = 0
for teacher, courses in teachers_info.items():
if len(courses) > max_count:
max_count = len(courses)
max_teacher = teacher
return max_teacher
Your solution is correct of course and there's nothing wrong with it, but when working with larger sets of data, avoiding multiple loops normally helps to keep the run time down.
I also think that using a descriptive name for the dictionary such as teachers_info helps to keep track of what's going on when you're working on bigger programs.
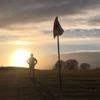
Stuart Wright
41,120 PointsIt looks like you have named your dictionary 'dict' in some places in your function, and 'dict1' in one place. If you make these consistent your code will pass the challenge.
Note that naming your variable 'dict' isn't good practice because it has a special meaning in Python (notice how it gets highlighted in orange in your code). It's best to choose names for variables that have no special meaning. I've used 'dict1' in this solution:
def most_courses(dict1):
count = 0
list1 = []
for value in dict1.values():
list1.append(value)
for item in list1:
if len(item) > count:
count = (len(item))
for key in dict1:
if count == len(dict1[key]):
return key

David Ton-Lai
3,268 PointsAh, thanks so much, this isn't the first time Syntax has messed me up, I need to be more careful!
Just curious, what do you think of my solution to this challenge, how would you have done it differently?
David Ton-Lai
3,268 PointsDavid Ton-Lai
3,268 PointsYes! Thanks for your input, I'm very new to this so I'm just improvising this stuff as I'm going along. Your solution is much more elegant!
You're absolutely right about using descriptive names, I was just being lazy since the code doesn't get saved. It's a bad habit, I know. :P
Taig Mac Carthy
8,139 PointsTaig Mac Carthy
8,139 PointsHi Stuart. Your solution is very elegant and simple – thanks for posting it. Maybe you can help me out: could you please explain why are you using
teacher, courses
with.items()
?I would normally do something like:
for teacher in teachers.values(): for course in courses:
But it doesn't work – so I was wondering if you could explain your code, please and thank you.Stuart Wright
41,120 PointsStuart Wright
41,120 PointsHi Taig. We use .items() when we want to have easy access to the key (teacher, in this case) and the corresponding value (list of courses, in this case) on the same loop iteration. If we only iterate over .values(), we have access to a list of courses on each iteration, but we do not know which teacher they belong to, which is fine in certain cases, but in this case it would make the challenge difficult to solve since we are asked to return the name of a teacher.