Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial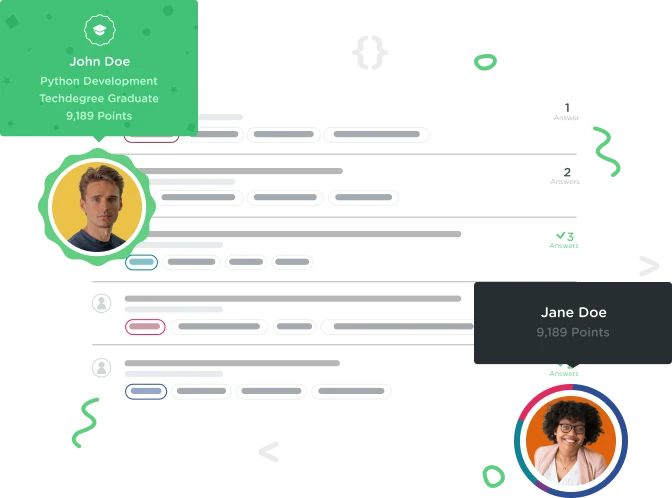
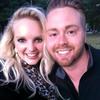
Jesse Fister
11,968 PointsThe code in app.js just opens a prompt, asks for a password, and writes to the document. Something is missing.
I know this one is simple simple but I'm stuck on this one. The code in app.js just opens a prompt, asks for a password, and writes to the document. Something is missing. Add a while loop, so that a prompt keeps appearing until the user types "sesame" into the prompt. I understand the while loop
while ( ) {
}
I'm just not sure what to put in it. A hint would be great. Thanks.
var secret = prompt("What is the secret password?");
while ( ) {
}
document.write("You know the secret password. Welcome.");
//while ( guess !== RandomNumber ) {
// guess = RandomNumber( upper );
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="app.js"></script>
</body>
</html>
13 Answers

Mark Long
15,763 PointsRemember that "the response from the prompt gets stored in the variable (var secret = response)." This means that "sesame" ought to be compared against the "response" which is the "var secret" variable. When the response does not equal the "sesame" password the expression evaluates "True" and the statement inside the while loop is executed. If it evaluates "False" the loop is broken and the next line of code is parsed.
In the loop below any answer other than "sesame" will cause the expression to evaluate "True" because the wrong answer is not equal (!==) to the right answer (it is "True" that the Response is not equal (!==) to the Password). That being the case the statement inside the while loop will execute and it causes a new prompt to occur requesting the secret password.
Once the user enters the correct password the expression will evaluate "False" because the correct password is equal to the correct password (it is "False" that the Response is not !== to the Password because the Response is equal (==) to the Password which make the expression evaluate "False.") and we jump the loop.
so...
var secret = prompt("What is the secret password?");
while (secret !== "sesame") { secret = prompt("Wrong Answer! What is the secret password?"); }
document.write("You know the secret password. Welcome.");

Chris Feltus
3,970 PointsThe answer posted by @Ali Amirazizi is incorrect and causes an infinite loop. It will pass the treehouse code challenge test for some reason, but if you run it in your browser it will create an infinite loop. It will not exit the loop even if you provide the correct answer 'sesame'.
Here is the correct answer
var secret = prompt("What is the secret password?");
while ( secret !== "sesame" ) {
secret = prompt("What is the secret password?");
}
document.write("You know the secret password. Welcome.");
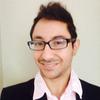
Ali Amirazizi
16,087 PointsThanks for the correction, I will delete my comment.
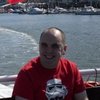
Sean Flanagan
33,235 PointsHi Chris.
I just tried your solution successfully. I would give you Best Answer but there's no such option under your comment. I've voted it up all the same.
Thanks
Sean :-)
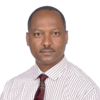
Yosef Ali
15,182 PointsThanks!

Christian Solorzano
6,606 PointsI don't understand this. Why is
secret = prompt("What is the secret password?");
underneath
while ( secret !== "sesame" ) {
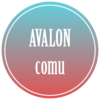
AVALONcomu Profile
36,146 PointsTry this. You should check if password is "sesame"
while (guess !== "sesame")
P.S. put one more secret = prompt() inside while to check password again and again.

Mekale Mccraney
6,035 Pointsdo{secret= prompt(“What is the secret password?”); } while(secret !== “sesame”) alert(“You know the secret password. Welcome!”)

hamcrjside
4,599 PointsI did it slightly different but it works.
var secret = prompt("What is the secret password?");
var password = "sesame"
while ( secret !== password ) {
secret = prompt("Secret password incorrect. Please try again.");
}
document.write("You know the secret password. Welcome.");
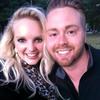
Jesse Fister
11,968 PointsThank you, Ali!

Manuel Pérez Vallenas
11,862 PointsThank you!!

Danny McCollins
731 PointsThanks for the help, guys. I was confused by having to add another var secret = ...
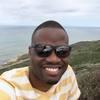
Jacques Simon
6,594 Pointshmm interesting. Thanks guys!
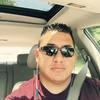
Wilton cruz-ramirez
10,159 Pointsthanks you so much guys!.
Mehran Moradi
11,140 PointsI am very confused. What is the reason to have another variable while we have a text name "sesame"???
var secret = prompt("What is the secret password?"); var password = "sesame"
while ( secret !== password ) { secret = prompt("Secret password incorrect. Please try again."); }
document.write("You know the secret password. Welcome.");
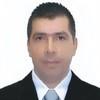
Omar Ocampo
3,166 Pointsvar secret = prompt("What is the secret password?"); var password = "sesame"
var count = 0 while ( secret !== password ) { secret = prompt("Secret password incorrect. Please try again."); }
document.write("You know the secret password. Welcome.");
"This was my answer because it was stated for only once"
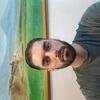
Vahe Gharagiozyan
10,088 PointsHere is another way of doing it.
let secret = "sesame";
do{secret = prompt("What is the secret password?"); }while(secret != "sesame") alert("You know the secret password. Welcome!")

Mekale Mccraney
6,035 PointsThis would be the correct answer
Lindsay Groves
4,811 PointsLindsay Groves
4,811 PointsThank for you this alternate explanation - it made a lot of sense to me!
damian celentano
3,822 Pointsdamian celentano
3,822 PointsBrilliant explanation, thank you very much
Timothy Nisonger
6,177 PointsTimothy Nisonger
6,177 PointsThanks Mark!