Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial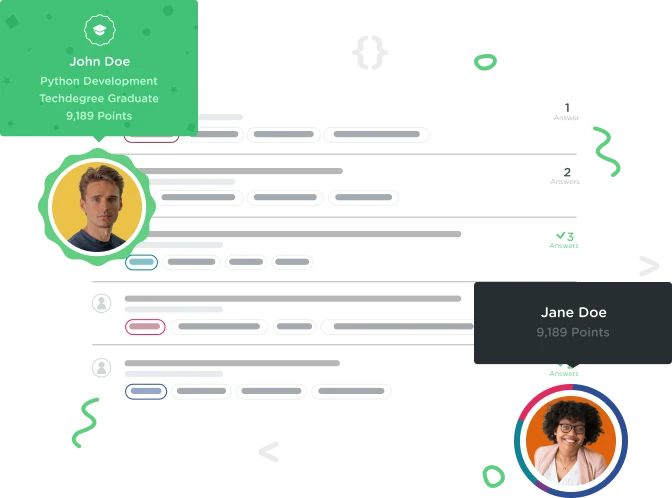
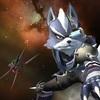
Heaven Lee
6,495 PointsThe Conditional Challenge - My Solution (feedback appreciated)
This is my solution to the conditional challenge. If anyone has any suggestions or tips I would love to hear them! :)
/*
1. Ask 5 Questions
2. Keep track of the number the user answered correctly
3. Provide a Final message after the quiz letting the user know the number of questions they got right
4. rank the player
*/
questions = 5;
var correct = 0;
var messageCorrect = "That is correct! ";
var messageWrong = "Wrong! ";
alert("5 Question Pokemon Quiz - Type true or false");
//question 1
var question1 = prompt("Snorlax is the heaviest pokemon. " + questions + "/5");
if ( question1.toUpperCase() === "FALSE" ) {
correct += 1;
alert(messageCorrect);
} else {
alert(messageWrong);
}
//question 2
questions -= 1;
var question2 = prompt("Poison moves are super-effective against grass. " + questions + "/5");
if ( question2.toUpperCase() === "TRUE" ) {
correct += 1;
alert(messageCorrect);
} else {
alert(messageWrong);
}
//question 3
questions -= 1;
var question3 = prompt("Kadabraβs spoon is made from gold. " + questions + "/5");
if ( question3.toUpperCase() === "FALSE" ) {
correct += 1;
alert(messageCorrect);
} else {
alert(messageWrong);
}
//question 4
questions -= 1;
var question4 = prompt("The Boulder badge is received after defeating Gym Leader Misty. " + questions + "/5");
if ( question4.toUpperCase() === "FALSE" ) {
correct += 1;
alert(messageCorrect);
} else {
alert(messageWrong);
}
//question 5
questions -= 1;
var question5 = prompt("Mr. Mime can be a female. " + questions + "/5");
if ( question5.toUpperCase() === "TRUE" ) {
correct += 1;
alert(messageCorrect);
} else {
alert(messageWrong);
}
alert("You got " + correct + " correct!");
//medal reward tier
var gold = "You received a GOLD medal!";
var silver = "You received a SILVER medal!";
var bronze = "You received a BRONZE medal!";
var noMedal = "Sorry, no medal for you today. Try again later!"
if ( correct === 5) {
alert(gold);
} else if ( correct === 4 || correct === 3 ) {
alert(silver);
} else if ( correct === 2 || correct === 1 ) {
alert(bronze);
} else if ( correct === 0 ) {
alert(noMedal)
}