Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial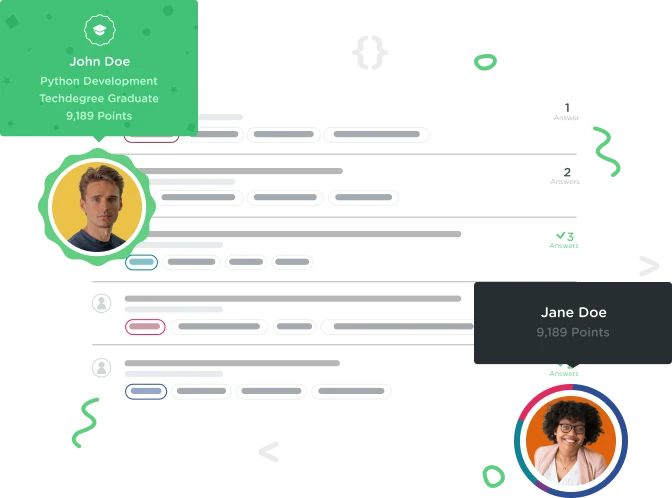

Amanda Nkosiyane
432 PointsThe div with the ID colorDiv should change red when the red button is clicked, and blue when the blue button is clicked.
The div with the ID colorDiv should change red when the red button is clicked, and blue when the blue button is clicked. Currently, though, only the red button is working. Can you figure out how to alter the javascript code without adding any lines to the blue button's event handler? Make sure a reference to colorDiv is held in a constant named colorSquare
const redButton = document.getElementById('redButton');
const blueButton = document.getElementById('blueButton');
redButton.addEventListener('click', (e) => {
const colorSquare = document.getElementById('colorDiv');
colorSquare.style.backgroundColor = 'red';
});
blueButton.addEventListener('click', (e) => {
// const colorSquare = document.getElementById('colorDiv');
colorSquare.style.backgroundColor = 'blue';
});
div {
height: 50px;
float: left;
padding-top: 40px;
padding-left: 20px;
}
#colorDiv {
padding: 0;
width: 100px;
height: 100px;
background-color: gray;
}
button {
height: 30px;
border-radius: 10px;
}
#redButton {
background-color: #ff5959;
border-color: red;
}
#blueButton {
background-color: lightblue;
border-color: #7C9EFC;
}
2 Answers

Joe Peel
14,384 PointsGot stuck as well on this for a while.
You need to move the - const colorSquare = document.getElementById('colorDiv'); up to the top next to the other variables. Otherwise the blue button handler can't access it.
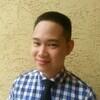
Tom Nguyen
33,500 PointsThis is what it would look like:
const redButton = document.getElementById('redButton');
const blueButton = document.getElementById('blueButton');
const colorSquare = document.getElementById('colorDiv');
redButton.addEventListener('click', (e) => {
colorSquare.style.backgroundColor = 'red';
});
blueButton.addEventListener('click', (e) => {
colorSquare.style.backgroundColor = 'blue';
})
Brian Foley
8,440 PointsBrian Foley
8,440 PointsThank you!
Sean Flanagan
33,235 PointsSean Flanagan
33,235 PointsHi Joe Peel.
Thank you for this; I moved the constant ColorSquare up to the line below the other two constants.
Sean
Daron Anderson
2,567 PointsDaron Anderson
2,567 PointsLOL I was like he literally just showed us in the video.