Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial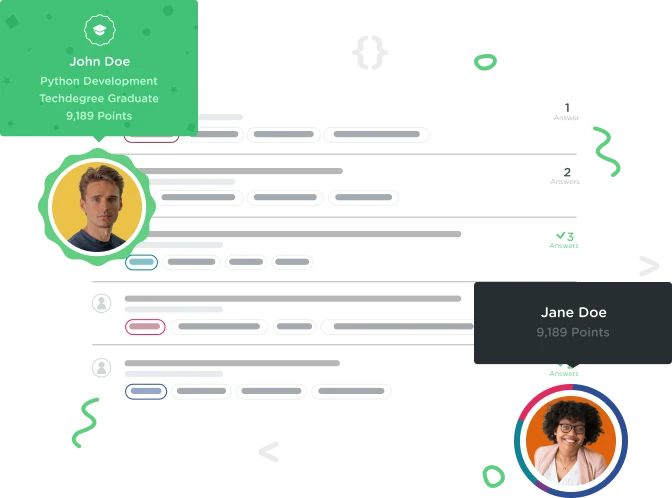

Lucas Keown
11,911 PointsThe filterCheckBox function isn't working for me
Hello! New to JavaScript. I've been following along with Guil's videos and haven't had this issue before. My filter checkbox isn't even showing up anymore and I'm getting the error 'Uncaught SyntaxError: Identifier 'i' has already been declared'. When submitting a name to the input form, the page just refreshes. I've looked over the code and didn't see any typos, but I would appreciate some extra eyes on it!
const form = document.getElementById('registrar');
const input = form.querySelector('input');
const mainDiv = document.querySelector('.main')
const ul = document.getElementById('invitedList'); //global constants
const div = document.createElement('div');
const filterLabel = document.createElement('label');
const filterCheckBox = document.createElement('input');
filterLabel.textContent = "Hide those who haven't responded";
filterCheckBox.type = 'checkbox';
div.appendChild(filterLabel);
div.appendChild(filterCheckBox);
mainDiv.insertBefore(div, ul);
filterCheckBox.addEventListener('change', (e) => {
const isChecked = e.target.checked;
const lis = ul.children;
if(isChecked) {
for (let i = 0, i < lis.length, i += 1) {
let li = lis[i];
if (li.className === 'responded') {
li.style.display = '';
} else {
li.style.display = 'none';
}
}
} else {
for (let i = 0, i < lis.length, i += 1) {
let li = lis[i];
li.style.display = '';
}
}
});
function createLI(text) { // createLI function is called when you hit the submit button -- line 26
const li = document.createElement('li');
const span = document.createElement('span');
span.textContent = text;
li.appendChild(span);
const label = document.createElement('label');
label.textContent = 'Confirmed';
const checkbox = document.createElement('input');
checkbox.type = 'checkbox';
label.appendChild(checkbox);
li.appendChild(label);
//edit button
const editButton = document.createElement('button');
editButton.textContent = 'edit';
li.appendChild(editButton);
//remove button
const removeButton = document.createElement('button');
removeButton.textContent = 'remove';
li.appendChild(removeButton);
ul.appendChild(li)
}
form.addEventListener('submit', (e) => { // when submitting this form, the inputted text is used to populate the createLI function
e.preventDefault();
const text = input.value;
input.value = '';
const li = createLI(text);
ul.appendChild(li);
});
ul.addEventListener('change', (e) => { // handles the checkbox in the list item
const checkbox = event.target;
const checked = checkbox.checked;
const listItem = checkbox.parentNode.parentNode;
if (checked) {
listItem.className = 'responded';
} else {
listItem.className = '';
}
});
ul.addEventListener('click', (e)=>{ // remove and edit button handler
if (e.target.tagName==='BUTTON' ){
const button = e.target;
const li = e.target.parentNode;
const ul = li.parentNode;
if (e.target.textContent === 'remove'){
ul.removeChild(li);
} else if(e.target.textContent === 'edit'){
const span = li.firstElementChild;
const input = document.createElement('input');
input.type = 'text';
input.value = span.textContent;
li.insertBefore(input, span);
li.removeChild(span);
button.textContent = 'save';
} else if(e.target.textContent === 'save'){
const input = li.firstElementChild;
const span = document.createElement('span');
input.type = 'text';
span.textContent = input.value;
li.insertBefore(span, input);
li.removeChild(input);
button.textContent = 'edit';
}
}
});
1 Answer

Lucas Keown
11,911 PointsFound my issue! I used commas in my for loops instead of semi-colons.