Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial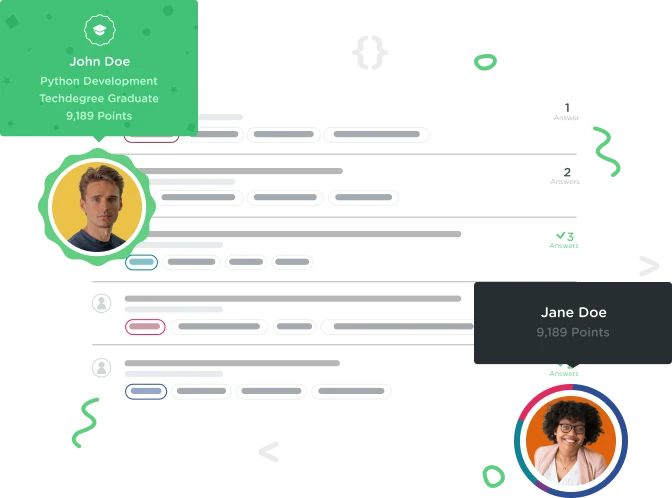

michaelco3
Courses Plus Student 2,814 PointsThe importance of the 1 inside or outside the Math.floor() method.
This question stumped me for hours. I am enjoying the course but I feel this step was too large based on the information received thus far. It was connect the dots but a few dots were missing. The placing of the 1 in my opinion needed more attention. This is how I got my head around it. It may help others. The significance of placing the 1 inside or outside the Math.floor method deserved a little more attention in my opinion. Enjoying the course, thanks dave and treehouse.
I only used the top and bottom outcomes for demonstration. I assigned Math.random() numbers for demonstration only. Remember it can produce any number from 0 to 0.99999 . It will never reach 1. Integer means a whole number. So Math.floor() ignores everything after a decimal point.
EG 1 : A number between 1 and whatever the user selects:
User selects 6 for this example. So the range is 1 – 6. var randomNumber = Math.floor(Math.random() * topNumber ) + 1; 1 added after Math.floor has been applied. It is outside the Math.floor parenthesis . 0 becomes 1, 5 becomes 6. If the 1 was not included outside the Math.floor method then 0 would be a possibility. Fig 1: topNumber = 6; Math.random produces .9999 (.9999 * topNumber)= 5.9994 Math.floor only wants the integer. So 5.9994 will become 5.
Fig 2: topNumber = 6; Math.random produces .01 (.01 * topNumber)= 0.06 Math.floor only wants the integer. So 0.06 will become 0.
The placing of the one outside the Math.floor method ensures the bottom possible number achievable is 1. It also ensures our top number is achievable.
EG 2 : A number between 0 and whatever the user selects:
User selects 6 for this example. var randomNumber = Math.floor(Math.random() * (topNumber + 1) ); 1 added inside the Math.floor parenthesis as 0 is an acceptable outcome and it allows the top number to be achieved. Fig 1: topNumber = 6; Math.random produces .9999 (.9999 * (topNumber + 1))= 6.9994 Math.floor only wants the integer. So 6.9994 will become 6.
Fig 2: topNumber = 6; Math.random produces .01 (.01 * (topNumber + 1))= 0.07 Math.floor only wants the integer. So 0.07 will become 0.
EG 3 : A number between x and y that the user selects.
The user gives the bottomNumber and the topNumber. So the user is setting the range. Our number can be anything between and including 10 – 25. That is why we subtract the bottomNumber from the TopNumber. To get the range. The bottomNumber is then added at the end. It is the lowest number acceptable. Otherwise our random number would be below the bottomNumber the user set. It would fall outside the range. Because the bottom number is going to be added after the Math.floor method, 0 is an acceptable possibility from our Math.floor method because 0 + bottomNumber = bottomNumber.
var randomNumber = Math.floor(Math.random() * (topNumber - bottomNumber + 1)) + bottomNumber;
Fig 1: topNumber = 25; bottomNumber = 10 Math.random produces .9999 (.9999 * (25 – 10 +1))= 15.9984 Math.floor only wants the integer. So 15.9984 will become 15. Our bottomNumber is then added outside the Math.floor method which produces 25.
Fig 2: topNumber = 25; bottomNumber = 10 Math.random produces .01 (.01 * (25 – 10 +1))= 0.16 Math.floor only wants the integer. So 0.16 will become 0. Our bottomNumber is then added outside the Math.floor method which produces 10.

aldom
1,989 PointsYour analysis is awesome and really helped me to understand how this method works.
Thank very much!
1 Answer
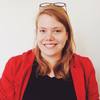
Anna Neyman
6,157 PointsI completely agree with you that the importance of + 1 and where it should go was not explained well enough prior to this exercise. What I also found confusing was the fact that the challenge asked to produce a random number BETWEEN the top and bottom numbers selected by the user - so I understood it as "a number between, but excluding the top and bottom numbers".
Anyways, thanks for this explanation, Michael!
lucybeck
6,055 Pointslucybeck
6,055 PointsAwesome!