Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial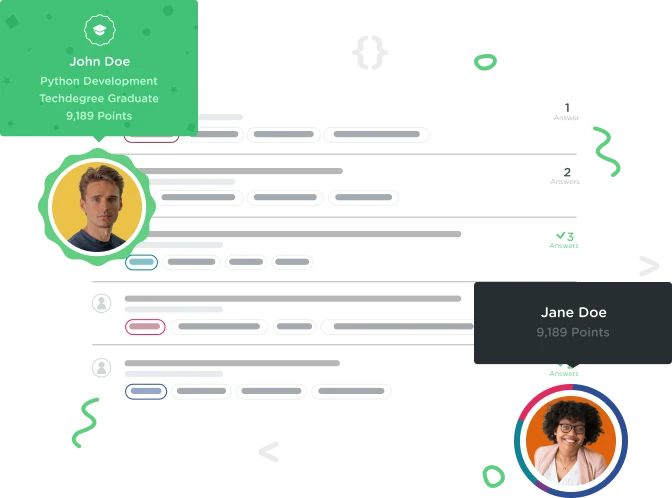

T K
21,479 PointsThe importance of "var" before a variable.
Hey all,
After confidently completing this little challenge I tested it, and it seemed to work perfectly.
However afterwards I noticed that, after focussing on Python for sometime, I forgot to put "var" before making a variable! ...yet the program still worked.
Purely out of interest, what are the repercussions of forgetting that var keyword?
Thanks :)
Tayler
2 Answers

Matt F.
9,518 PointsHi Tayler,
Leaving off the var can lead to inadvertently setting/changing global variables. For example:
color = "blue";
function a() {
var color = "green";
alert('I just set a local variable in function a and it is set to: ' + color);
}
function b() {
color = "yellow";
alert('In function b however, I omitted the var and now my color (' + color + ') will leak into the globa scope!')
}
alert("Before either function runs, the color is: " + color);
alert("Runnign function a");
a();
alert('After function a ran, the color is still: ' + color);
alert("Running function b");
b();
alert('Uh oh, now the color outside of the function is: ' + color);
function secretHolder() {
var favoriteDrink = prompt("What is your favorite drink?");
}
function notASecretHolder() {
favoriteFood = prompt('What is your favorite food?');
}
var favoriteDrink = "unknown";
var favoriteFood = "unknown";
secretHolder();
alert('Favorite drink: ' + favoriteDrink)
notASecretHolder();
alert('I can still access favoriteFood outside of the function, because the var was omitted: ' + favoriteFood);
So let's look at the favorite drink/food example in more depth. The browsers of today are very complex with how they compile/run the code - but the basic idea is this:
There is a function statement for secretHolder. Since we are in the global scope, I will store it there to be accessed anywhere in the application. I will also store the function's code now. (Repeat for notASecretHolder)
There is a variable declaration for favoriteDrink. Since we are in the global scope, I will set aside space in memory for it in the global scope, but set it to undefined for now. (Repeat for secretHolder)
Okay, second passthrough: I have a reference to a favoriteDrink, was this declared in the global scope? Since it was, its "unknown" string value will get assigned to it. (Repeat for favoriteFood)
The code wants to run secretHolder
does it exist? - Yes
is it a function? - Yes
Running it...
Creating a new scope for this instance of secretHolder
There is a variable declaration for favoriteDrink. since I am inside the secretHolder scope, I will set aside memory for it inside this current (local) scope.
Second passthrough - Ok, run the prompt and assign the value to favoriteDrink
Does favoriteDrink exist on the secretHolder scope? = Yes, assign the value to it Exit secretHolder function instance
The code wants to run notASecretHolder
does it exist? Yes
is it a function? Yes
Running it
Creating a new scope for this instance of notASecretHolder
There are no variable declarations NOTE THE DIFFERENCE HERE
Second passthrough - Ok run the prompt and then assign it to FavoriteFood
Does favoriteFood exist on the notASecretHolder scope? - No
Checking the next scope out, which is the global scope - does favoriteFood exist here? - Yes -assign the value of the prompt to the favoriteFood variable on the global scope.
Exiting notASecretHolder
(As a side note, if it did not exist on the global scope, it would then create the variable on the global scope, unless in Strict Mode)
(My understanding of this comes largely from Kyle Simpson's Scope and Closures book in the You Don't Know JS series. My interpretation is probably imperfect, so I would recommend you check out that book).

Damien Watson
27,419 PointsHi Tayler,
As Matt said, but it took me a little to write up so I'll still post :)
'var' defines the variable in the local instance. If inside a function you use a variable that is already declared globally it will use the global setting and update the global variable accordingly. Defining it inside the function using 'var' means it is set inside that function locally and doesn't affect the global definition.
This can all sound confusing, so a quick example following:
var width = 5;
var height = 5;
for (i=0; i<width; i++) {
document.write('<br>first loop: '+i+'<br>');
loopIt();
}
function loopIt() {
document.write('second loop<br>');
for (i=0; i<height; i++) {
document.write('*');
}
}
The 'i' inside the first loop starts at '0'. The function is called which loops it up to 5 which is set globally because there is no 'var' in front. When the program comes back to the first loop 'i' is now set to '5' which is not less than '5' so it exits the loop.
If you change the function to have a 'var', it will loop through as expected:
function loopIt() {
document.write('second loop<br>');
for (var i=0; i<height; i++) {
document.write('*');
}
}

Matt F.
9,518 PointsHi Damien,
Thank you. I think your example and explanation is very good also.

T K
21,479 PointsThank you Damien, I really appreciate you taking the time. Both of your examples were great!
Damien Watson
27,419 PointsDamien Watson
27,419 PointsMatt, your comment is easier to understand, nice and clean. :)
T K
21,479 PointsT K
21,479 PointsMatt, thank you very much for such a detailed answer! I understand now.
Going from one language to another is a minefield of stuff like this I'm finding.