Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial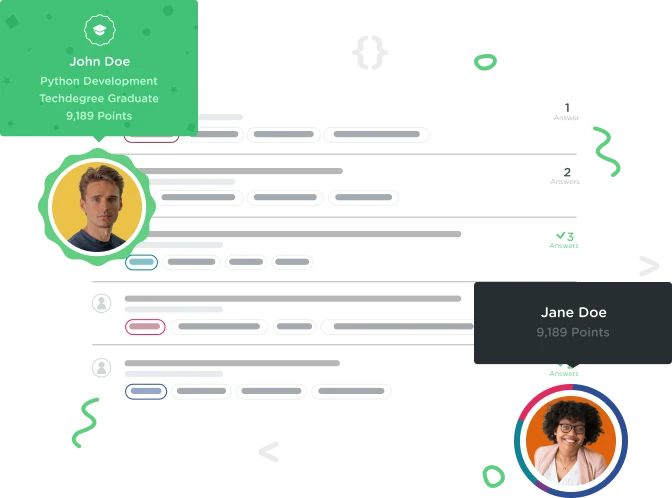
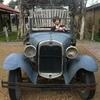
Brendan Moran
14,052 PointsThe javaScript Way...
... as opposed to the "Writing HTML strings in javaScript Way."
Here's my code (it works). I did it using the DOM and the functions that javaScript has to interact with the DOM. I am wondering, why write HTML strings in javaScript? Is there a performance benefit?
var employeeListDiv = document.getElementById("employeeList"),
xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if (xhr.readyState === 4) {
var employees = JSON.parse(xhr.responseText),
employeeStatusUL = document.createElement("ul");
employeeStatusUL.classList.add('bulleted');
for (var i = 0, length = employees.length; i < length; i++) {
var listItem = document.createElement("li"),
liClassList = listItem.classList;
//add employee name to <li>
listItem.textContent = employees[i].name;
//publish if present or not present
if (employees[i].inoffice === true) {
liClassList.add('in');
} else if (employees[i].inoffice === false) {
liClassList.add('out');
}
//append <li> to <ul>
employeeStatusUL.appendChild(listItem);
}
//update the employee list <div> with the new <ul>
employeeListDiv.appendChild(employeeStatusUL);
}
}
xhr.open('GET', 'data/employees.json');
xhr.send();
1 Answer
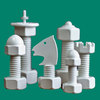
Steven Parker
231,007 PointsI doubt there's much of a performance difference, if any.
But the example shown in the video creates code that is a bit more concise, and a bit easier to comprehend at first glance. When performance is not compromised, easier to read and/or smaller code is a plus.
Brendan Moran
14,052 PointsBrendan Moran
14,052 PointsDuly noted. I agree that his code is much more instantly readable. I wasn't sure if he was using HTML because that is how he actually prefers to do it (or it is a best practice), or whether he was doing that to make a course that was more accessible to people who are maybe focusing more on design and less on javaScript. I'm in the full stack JS track now, and I noticed that in the intro to JS classes, Dave used a lot of HTML strings, whereas Andrew, in the later JS courses, went into the methods and properties that manipulate the DOM. I found that I much preferred using the JS methods, even if it meant a few more lines of code. The flow of the logic (create the element, then modify and append it) flowed better for me. I was surprised when we went back to the HTML strings way of doing things in this section; I had thought that that was included in the earlier videos just because we hadn't gone through all the DOM manipulation stuff yet. Hence, after seeing it come up here again, I was wondering whether this was a common real-world practice or not.
Thanks for the input!