Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial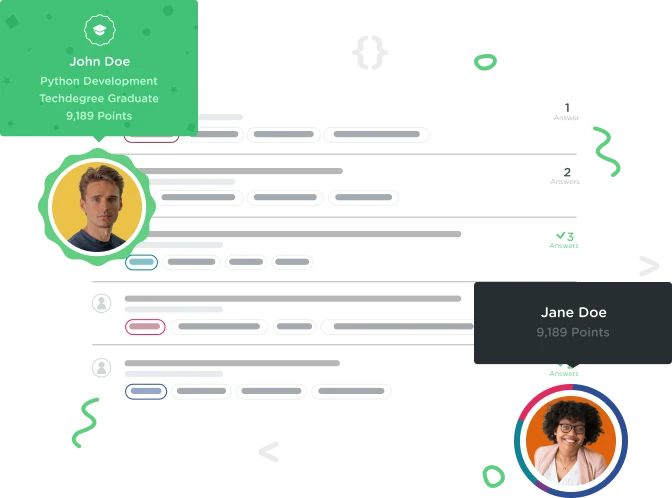

Joseph Carrillo
2,137 PointsThe Random Challenge Solution
Hello All,
I was trying to create a random generator but i think i did it correctly. Please if you can verify that i did do it correctly.
I am taking in two inputs from the prompts.
Taking the strings and converting to integers.
Adding those two values together then dividing it to get one single value.
Then i am taking that value and working with the Math.random object.
Here is my code below:
document.write('<h1> This is a program that will take two number inputs from 1 to 10.</h1><br /><br />'); document.write('<h3> Then it will use the two numbers to return a random number from the two inputs.</h3><br /><br />');
var numOne = prompt('Please put the first number in from 1 to 10');
resultOne = parseInt(numOne);
var numTwo = prompt('Please put the second number in from 1 to 10');
resultTwo = parseInt(numTwo);
randomNum = (resultOne + resultTwo) / 2;
document.write(Math.floor((Math.random() * randomNum) + 1));
Thank you for your help!
JC
3 Answers
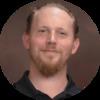
Michael Davis
Courses Plus Student 12,508 PointsOk, so here is your code as I understand it.
document.write('<h1> This is a program that will take two number inputs from 1 to 10.</h1><br /><br />'); document.write('<h3> Then it will use the two numbers to return a random number from the two inputs.</h3><br /><br />');
var numOne = prompt('Please put the first number in from 1 to 10');
resultOne = parseInt(numOne);
var numTwo = prompt('Please put the second number in from 1 to 10');
resultTwo = parseInt(numTwo);
randomNum = (resultOne + resultTwo) / 2;
document.write(Math.floor((Math.random() * randomNum) + 1));
Now, if you are trying to get a random number between 1 and the average of the two numbers... you're close.
However, if you're wanting to get a random number between resultOne
and resultTwo
, a few changes are in order.
Math.random()
will generate a number between 0 and 1, without including 1. The random number will never be 1. This is why we add 1 at the end. If you tried Math.random(10)
you could get any number from 0 to 10, without ever reaching 10. It could be 9.99999999, but you cannot get 10. We use Math.floor()
which will drop everything after the decimal. So if Math.random(10)
produces 9.898989, Math.floor()
turns it into 9.
If you wanted to get a random number between 10 and 30, then you would use
Math.floor( Math.random(21) + 10 )
// Possible numbers are 10 - 30
Formula:
Math.floor(Math.random()*(max-min+1)+min);

Joseph Carrillo
2,137 PointsI see what you mean now. I am going to dissect it to how i understand it.
So we have the formula
document.write(Math.floor(Math.random() * (max - min) + min));
Math.floor will round down to nearest whole number.
Math.random will give us a from 0 to 1 without calculating 1.
max - min which in my case would be 10 - 1, which would give me random number 0 - 9
the + min would add 1 to the random number which would give me 1 - 10 based upon the input.
Whatever you put in the parameters of the Math.random object would be the max number you would have a random number to ?
For example, Math.random(25) * (25 - 1) + 1
Revised Code:
document.write('<h1> This is a program that will take two number inputs from 1 to 10.</h1><br /><br />'); document.write('<h3> Then it will use the two numbers to return a random number from the two inputs.</h3><br /><br />');
var numOne = prompt('Please put the first number in from 1 to 10');
resultOne = parseInt(numOne);
var numTwo = prompt('Please put the second number in from 1 to 10');
resultTwo = parseInt(numTwo);
document.write(Math.floor(Math.random() * (resultTwo - resultOne) + resultOne ));
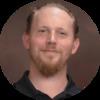
Michael Davis
Courses Plus Student 12,508 PointsYour Revised code:
document.write('<h1> This is a program that will take two number inputs from 1 to 10.</h1><br /><br />'); document.write('<h3> Then it will use the two numbers to return a random number from the two inputs.</h3><br /><br />');
var numOne = prompt('Please put the first number in from 1 to 10');
resultOne = parseInt(numOne);
var numTwo = prompt('Please put the second number in from 1 to 10');
resultTwo = parseInt(numTwo);
document.write(Math.floor(Math.random() * (numOne - numTwo) + numOne ));
Everything is right on, except:
document.write(Math.floor(Math.random() * (numOne - numTwo) + numOne ));
This should be:
document.write( Math.floor(Math.random() * (maxNum - minNum + 1) + minNum ) );
Remember the formula: Math.floor(Math.random()*(max-min+1)+min);
Also, test it in the browser console.
Type:
Math.floor(Math.random() * (20 - 10 + 1) + 10);
It'll give you a random number between 10 and 20 every time.

Joseph Carrillo
2,137 PointsI see if i do not add the + 1 to the max - min then i will only calculate up to 9.
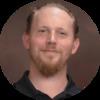
Michael Davis
Courses Plus Student 12,508 PointsExactly :-)
Joseph Carrillo
2,137 PointsJoseph Carrillo
2,137 PointsMichael,
Thanks for your reply. I see what I am doing now. If I am inputting 6 and it adds 6 then that is 12 / 2 which is 6. Then it will give me a random number between 1 and 6.
I am trying to get a number between 1 and 10 using the numbers I input. So I am assuming I would use the solution you advised.
I am still unclear about why (max - min + 1) + min. Could you explain a little further and dissect what this means.
I am thinking if it takes 10 and 5 as the inputs. Which would be the result 5 + 1 which is 6. Then it would add 5 which would be 11. So then would it give you a random number from 1 and 11?
Thanks, JC
Michael Davis
Courses Plus Student 12,508 PointsMichael Davis
Courses Plus Student 12,508 PointsYou're close. Using you're example, here is some pseudo-code.
Let's break this down:
So we had 10.9899, but we wanted a number between 1 and 10, right? Well, since we introduce our friend
Math.floor()
this is what happened.I hope this helps!
Joseph Carrillo
2,137 PointsJoseph Carrillo
2,137 PointsMichael will you see my revised post! I believe i did it correctly. (max - min) does matter if you put 1 in the first prompt or the second prompt. Or 10 in the first and 1 in the second. It will still do the calculations correctly.
Joseph Carrillo
2,137 PointsJoseph Carrillo
2,137 PointsThanks Michael! I think if I break it down further would be less confusing. It is so much easier to hard code the number than working with variables. I am a novice so it was hard understanding the formula. I get the concepts but the logic is not there for me.
Michael Davis
Courses Plus Student 12,508 PointsMichael Davis
Courses Plus Student 12,508 PointsBiggest thing to remember is that
Math.random()
will give a random number between 0, and just before 1. So anything you multiply it by will be between 0 and just before that number.So If I did
Math.random() * 40
, I could get any number between 0 and 39.999999999.Also,
Math.floor()
removes everything after the decimal. It does not round, it simply ignores anything after the decimal. So the same 39.99999999 then becomes 39.So, given the above, my
Math.random() * 40
is actually a 0 - 39 random. This is why we add 1 after the number is generated. This makes it a number between 1 and 40, inclusive.