Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial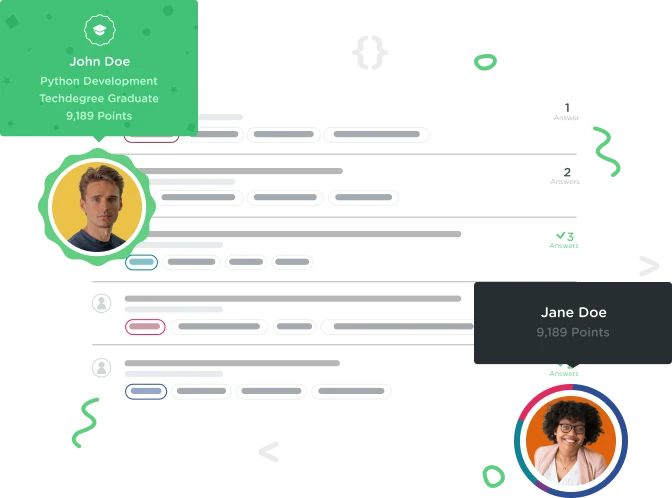
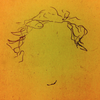
bristel
1,252 PointsThe ranking portion of the code doesn't let the program run. When I remove it, the program runs perfectly. Why?
var correct = 0;
var q1 = prompt("what is your name?");
if (q1.toUpperCase === 'BRIEN') {
correct =+ 1;
}
var q2 = prompt("what is your brothers name?");
if (q2.toUpperCase === 'JOHN') {
correct =+ 1;
}
var q3 = prompt("what is your moms name?");
if (q3.toUpperCase === 'HEATHER') {
correct =+ 1;
}
var q4 = prompt("what is your dads name?");
if (q4.toUpperCase === 'HANS') {
correct =+ 1;
}
var q5 = prompt("question 5 goes here");
if (q5 === '41') {
correct =+ 1;
}
document.write("Congrats, you finished! You answered "+ correct +" correctly.");
// if i erase the ranking portion below the program runs but when its left it the program won't even start. Why?
if (correct === 5) {
document.write('You got the GOLD!');
} else if (correct >= 3) {
document.write('You got the SILVER!');
} else if (correct >= 1) {
document.write('You got the BRONZE!');
} else (correct === 0) {
document.write('You placed 4th. Not good.');
5 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Brien,
You can't have a condition on your else
clause. Only on the if's.
You'd want it to be like this:
} else {
document.write('You placed 4th. Not good.');
}
Also, I don't think your correct answers are being incremented correctly. You're setting it to plus one each time because you reversed the +=
It should be correct += 1;
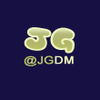
Jonathan Grieve
Treehouse Moderator 91,253 PointsHi there Brien,
It looks like you didn't close out your last else condition.
So
if (correct === 5) {
document.write('You got the GOLD!');
} else if (correct >= 3) {
document.write('You got the SILVER!');
} else if (correct >= 1) {
document.write('You got the BRONZE!');
} else (correct === 0) {
document.write('You placed 4th. Not good.');
}
Should work for you. :-)
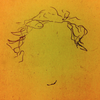
bristel
1,252 PointsHi Jonathan,
Thank you for responding so quickly! And my apologies for the vulgarity of my 5th question. I completely forgot I wrote that before posting publicly. : /
Either way, below is my code for the ranking portion. I appears I didn't copy and paste all of it. But upon re check it and testing it the program still doesn't run.
// if i erase the ranking portion below the program runs but when its left it the program won't even start. Why? if (correct === 5) { document.write('You got the GOLD!'); } else if (correct >= 3) { document.write('You got the SILVER!'); } else if (correct >= 1) { document.write('You got the BRONZE!'); } else (correct === 0) { document.write('You placed 4th. Not good.'); }
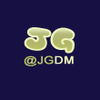
Jonathan Grieve
Treehouse Moderator 91,253 PointsHi Brien
I didn't even notice :) I've taken the liberty of editing that particular part of your code so you're alright now. :)
As for your code, it looks right to me. There are a number of ways of tacking the solution to the challenge as the video suggests but this good.
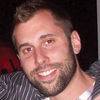
Aaron Chiandet
9,826 PointsI hope this isn't viewed as a hijack but I can't seem to get mine to run either. I've reviewed the solutions suggested and my code doesn't appear to have the same concerns. However OP still can't get his to run so I guess this is still an open issue. Do we need to do anything to the html file?
var answers = 0;
// Ask at least five questions
var question1 = prompt("what color is the sky?");
if (question1.toUpperCase() === "BLUE") {
answers += 1;
}
var question2 = prompt("what color is grass?";
if (question2.toUpperCase() === "GREEN") {
answers += 1;
}
var question3 = prompt("what color is milk?";
if (question3.toUpperCase() === "WHITE") {
answers += 1;
}
var question4 = prompt("what color is an apple?";
if (question4.toUpperCase() === "RED") {
answers += 1;
}
var question5 = prompt("what color is my vape?";
if (question5.toUpperCase() === "BLACK") {
answers += 1;
}
// Keep track of the number of questions the user answered correctly
document.write("You got " + answers + "right");
// Provide a final message after the quiz letting the user know the number of questions he or she got right.
/* Rank the player.
If the player answered all five correctly, give that player the gold crown:
3-4 is a silver crown;
1-2 correct answers is a bronze crown and
0 correct is no crown at all.
*/
if (answers === 5) {
document.write("You earned the gold crown");
} else if (answers >= 3){
document.write("You earned the silver crown");
} else if (answers >= 1) {
document.write("You earned the bronze crown");
} else {
document.write("No crown for you!");
}

Jason Anello
Courses Plus Student 94,610 PointsHi Aaron,
You're missing the closing right parenthesis on your call to prompt()
for the 2nd through 5th questions.
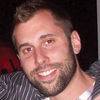
Aaron Chiandet
9,826 PointsDoh. Gracias Jason!
Jonathan Grieve
Treehouse Moderator 91,253 PointsJonathan Grieve
Treehouse Moderator 91,253 PointsOf course!!! :)
bristel
1,252 Pointsbristel
1,252 PointsYou're both awesome! Thank you. :)