Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial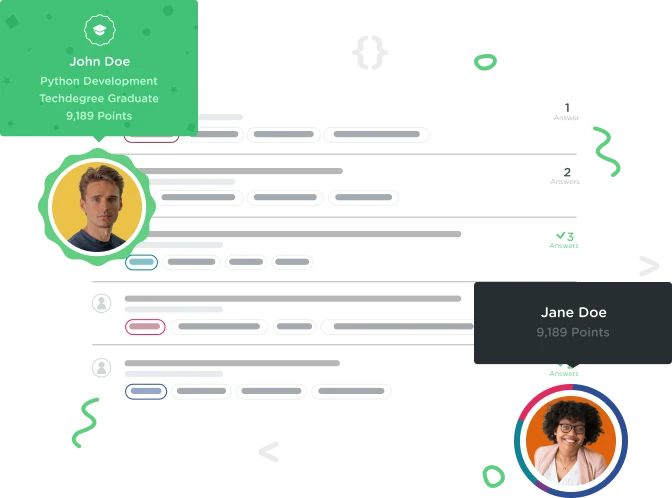
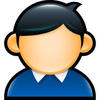
Daniel Hildreth
16,170 PointsThe Student Record Search Challenge (Further Work)
At the end of the Search Challenge for finding students, it asks us to see if we can go further and create code to see if there are multiple students with the same name, display those names, alert them when there's not a student found with that name, and etc. Can someone help walk me through this extra step so maybe I have a better understanding of JavaScript? This is the code I currently have. When I preview the code, it stops working. Why does it stop working all because I added that last else if statement in it?
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport( student ) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt('Search student records: type a name [Jody] (or type "quit" to end)');
if (search === null || search.toLowerCase() === 'quit') {
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name === search) {
message = getStudentReport( student );
print(message);
} else if {
if (student.name !== search) {
alert('There is no student with that name found.');
}
}
}
4 Answers

johansundstrom
Courses Plus Student 3,007 PointsWith only a quick look at the code, the "else if"-statement have wrong syntax and ought to be the problem. Try: "else if(YOUR STATEMENT){".
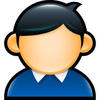
Daniel Hildreth
16,170 PointsOk I fixed that syntax error. Two more questions. If you put my new code into the workspaces then hit preview, it displays "Student not found" like 4 times on the page instead of once, and also keeps it displayed if I put another student into the search that is listed. How do I change this to only one text stuff per search (if that makes sense)? My other question is if I had 2 students with the same name, how do I search for those 2 students individually?
Here's my code:
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport( student ) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt('Search student records: type a name [Jody] (or type "quit" to end)');
if (search === null || search.toLowerCase() === 'quit') {
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name === search) {
message = getStudentReport( student );
print(message);
} else if (student.name !== search) {
document.write('<p>There is no student with that name found.</p>');
}
}
}
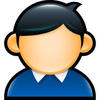
Daniel Hildreth
16,170 PointsI changed the code for the student records as well (added a student with the same name).
var students = [
{
name: 'Dave',
track: 'Front End Development',
achievements: 158,
points: 14730
},
{
name: 'Jody',
track: 'iOS Development with Swift',
achievements: '175',
points: '16375'
},
{
name: 'Jordan',
track: 'PHP Development',
achievements: '55',
points: '2025'
},
{
name: 'John',
track: 'Learn WordPress',
achievements: '40',
points: '1950'
},
{
name: 'Trish',
track: 'Rails Development',
achievements: '5',
points: '350'
}
{
name: 'John',
track: 'Rails Development',
achievements: '100',
points: '1500'
}
];

Eric Flood
3,920 PointsI am having this problem too. It cycles through the loop for each possible candidate and alerts each time. How can this be avoided?

J.R. Champ
13,263 PointsIf that can you help guys.
To be able to print all the same names from a single search, you need to declare your variable "message" outside of the "for loop", then when a student name match the search you need to add "+=" it in the "message" variable so when the loop restart it will add the second name also and so on.
To be able to print "Student Not found" when the search doesn't match any student, you can add, outside de "for loop" a variable named "found" then set it to false. Then each time search match a student in your array, set the found variable to true. Then after the "for loop" just add a condition to see if the variable "found" is not true, if so print the "student not found" string.
var answer = "";
while (true){
answer = prompt("Search for a student. Type the name of the student or type [Quit] to exit");
if ( answer === null || answer.toLowerCase() === "quit"){
break;
}
if (answer != "") {
var message = "";
var found = false;
for (i=0; i < students.length; i++) {
var student = students[i];
if (answer.toLowerCase() === students[i].Name.toLowerCase() ) {
message += getReport( student );
print(message);
found = true;
}
if (!found) {
print("<p>Student named " + answer + " not found</p>");
}
}
}
}
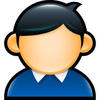
Daniel Hildreth
16,170 PointsSo I have not been back to this thread in awhile because I forgot about it. I need help getting my code when it run to not repeat names and such, and that when new names are found the old ones are deleted off the window. I also need help getting my code to be able to find someone that has the same name as another student.
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsThis is what Johan refers to (syntax highlighting for ya buddy :)