Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial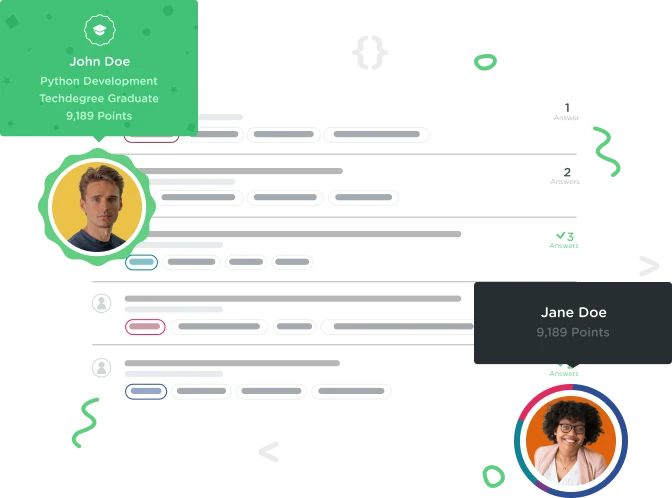

Kenan Xin
16,139 PointsThe Student Record Search Challenge Solution
A simple solution for sharing
var message = '';
var student;
var found = false;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport(student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
var input = prompt("Search student records: type a name or type 'quit' to end");
if (input === null || input.toLowerCase() === "quit") break;
else {
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (input === student.name) {
message += getStudentReport(student);
found = true;
}
}
if (found == false){
print("No student named "+ input + " is found.")
}else{
print(message);
}
}
}
4 Answers

Kristin Anthony
11,099 PointsVery helpful! I was pulling my hair out. But I noticed that, after the first time you find someone, if you search again for someone who isn't there, the message doesn't print. This is because the found variable remains true. To solve this, I inserted
found = false
in the while loop before the for loop.
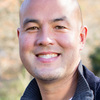
Ernest Son
13,532 PointsI did it like this.....please offer feedback!
var message = 'Your student could not be found. Please check the spelling';
var student;
var search;
var cycles = 0;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport (student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while ( true ) {
search = prompt("Search student records: type a first and last name [Jody Barnes] (or type 'quit' to end)");
if ( search === null || search.toLowerCase() === 'quit' ) {
break;
} else {
for ( var i = 0; i < students.length; i += 1 ) {
student = students[i];
if ( student.name.toLowerCase() === search.toLowerCase() ) {
message = getStudentReport(student);
}
}
print(message);
}
}

Riks Fontein
7,523 PointsI can't find my bug, can someone please review my code:
var message = ''; var student; var search;
function print(message) { var outputDiv = document.getElementById('output'); outputDiv.innerHTML = message; }
function getStudentReport( student ){ var report = '<h2>Student: ' + student.name + '</h2>'; report += '<p>Track: ' + student.track + '</p>'; report += '<p>Points: ' + student.points + '</p>'; report += '<p>Achievements: ' + student.achievements + '</p>'; return report }
while (true){ var answer = prompt('Search student records: type a name or type quit to end'); if (answer === null || answer.toLowerCase() === 'quit'){ break; } else { for (var i = 0; i < students.length; i += 1) { student = students[i]; if ( student.name === search) { message = getStudentReport( student ) }; }; }; }; print(message)
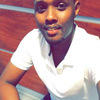
Abdifatah Mohamed
5,289 PointsYou are missing variable students which is undefined here. You need to list var students = [ {}, {}, {}, {}, {},]; after that you will be able to see your bug.
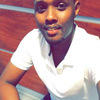
Abdifatah Mohamed
5,289 PointsThis is my solution when you type a name that doesn't exist in the set of students:
else { if (student.name != search) { print( 'No student ' + search + ' was found.'); } }
Kenan Xin
16,139 PointsKenan Xin
16,139 Points