Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial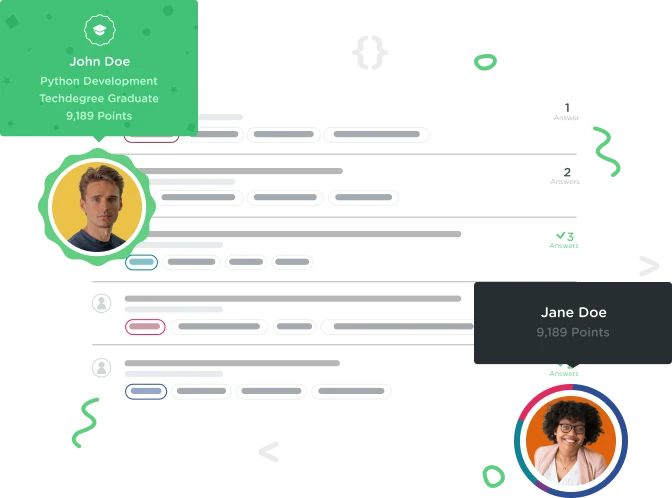
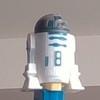
Teacher Russell
16,873 PointsThere are three list items in the index.html file. We want to be able to enter a 0, 1 or 2 in the text field to embolden
I'm baffled as to what the challenge is even asking here, and it seems I'm not alone. I don't understand the goal, and .....this almost seems like it belongs in another section or course.
const laws = document.getElementsByTagName('li');
const indexText = document.getElementById('boldIndex');
const button = document.getElementById('embolden');
button.addEventListener('click', (e) => {
const index = parseInt(indexText.value, 10);
for (let i = 0; i < laws.length; i += 1) {
let law = laws[i];
// replace 'false' with a correct test condition on the line below
if (false) {
law.style.fontWeight = 'bold';
} else {
law.style.fontWeight = 'normal';
}
}
});
<!DOCTYPE html>
<html>
<head>
<title>Newton's Laws</title>
</head>
<body>
<h1>Newton's Laws of Motion</h1>
<ul>
<li>An object in motion tends to stay in motion, unless acted on by an outside force.</li>
<li>Acceleration is dependent on the forces acting upon an object and the mass of the object.</li>
<li>For every action, there is an equal and opposite reaction.</li>
</ul>
<input type="text" id="boldIndex">
<button id="embolden">Embolden</button>
<script src="app.js"></script>
</body>
</html>
4 Answers

Martin Lecke
14,385 PointsHey You are looking for the if statement
i == index
Why? We check if the li number is the same as written in the textbox. All other li will be font-weight normal
const laws = document.getElementsByTagName('li');
const indexText = document.getElementById('boldIndex');
const button = document.getElementById('embolden');
button.addEventListener('click', (e) => {
const index = parseInt(indexText.value, 10);
for (let i = 0; i < laws.length; i += 1) {
let law = laws[i];
// replace 'false' with a correct test condition on the line below
if (i == index) {
law.style.fontWeight = 'bold';
} else {
law.style.fontWeight = 'normal';
}
}
});
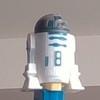
Teacher Russell
16,873 PointsThanks. Frustrated I needed an answer given to me. It's been a while. I was trying to use the law var that stored i to make the comparison to the input. I also don't get the second argument in the parseInt function. Can you explain that?

Iain Simmons
Treehouse Moderator 32,305 PointsThe index
is the position in the list of the item that should be made bold. In JavaScript, indexes start at 0 (zero). So 0, 1, or 2 would correspond to the first, second and third laws respectively.
The code gets the value
of the boldIndex
/indexText
field to determine which one to make bold (i.e. the number that the user entered).
So the loop goes through the list items, and if the index of the list item (which would be the value of i
in that iteration of the loop) is equal to the index
that the user entered, change the font weight of the text to bold
, otherwise it should be normal
.
Hope that helps!
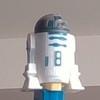
Teacher Russell
16,873 PointsI was trying to use the law var that stored i to make the comparison to the input. I also don't get the second argument in the parseInt function.

Iain Simmons
Treehouse Moderator 32,305 PointsThe second argument of the parseInt function is the base. You almost always want it to be 10 so you're dealing with our normal base 10 counting system.
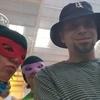
Robbie Thomas
31,093 PointsI did the same thing that Teacher Russell did, that didn't work.
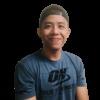
Faiz Hamdan
5,215 Pointslet law = laws[i]
I thought it make sense to put 'law' == index? isn't it the same with i == index?
On the selector.
const laws = document.getElementByTagName('li');
In general, laws is 'li'?
Putting :
law[i] === index
There's still laws which is the li and [i] is the 0, 1, 2.
Dave StSomeWhere
19,870 PointsDave StSomeWhere
19,870 PointsMy guess is that the goal is to accept a user entered value (one of three values), triggered by a button click event that will toggle the font weight of one of the list items (one of three list items). So, everything is there except the IF condition. Does that help?