Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial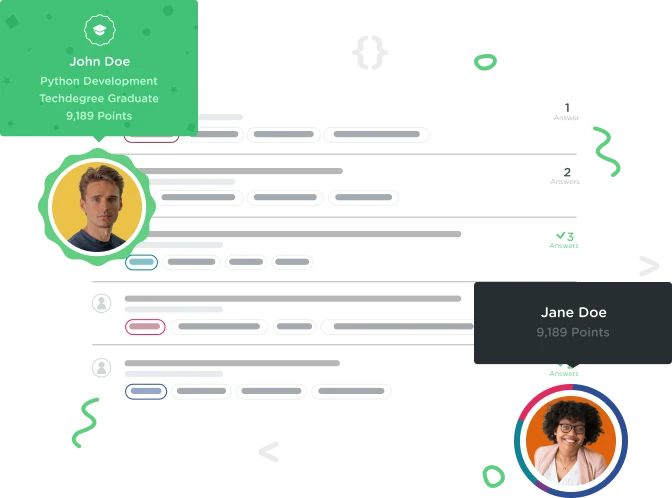
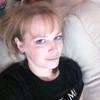
Carina De Jager
1,779 PointsThird section of code isn't passing
Ok guys... Where have I gone wrong in the Stats function... (sigh)
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
most_classes = {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
'Kenneth Love': ['Python Basics', 'Python Collections']}
def most_classes(my_dict):
teachers_list = {}
for teacher in my_dict:
teachers_list[teacher] = len(my_dict[teacher])
return max(teachers_list, key=teachers_list.get)
def num_teachers(teachers):
teacherNumber = 0
for teacher in teachers:
teacherNumber += 1
return teacherNumber
def stats(teachers):
newlist = []
for name, item in teachers:
newlist.append((teachers[name], len(teachers[item]))
return newlist
3 Answers
William Li
Courses Plus Student 26,868 PointsHi, Carina, This line
for name, item in teachers:
is not valid Python code, you can't have more than 1 iterating variable while doing for loop. Additionally, on the following line
newlist.append((teachers[name], len(teachers[item]))
There're 3 opening parenthesis VS 2 closing parenthesis, this is a syntax error; also, elements enclosed with () is tuple in Python, but this part of challenge is asking for list within list, so you need to use [] literal instead.
One way to fix that is
def stats(teachers):
newlist = []
for name in teachers:
newlist.append([name, len(teachers[name])])
return newlist
Alternatively, if you really want to retrieve both the key and value each time you loop through the dictionary, you may use the .items() method on the dictionary.
def stats(teachers):
newlist = []
for key, value in teachers.items():
newlist.append([key, len(value)]) # append [<name>, <number of classes>] to newlist
return newlist
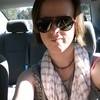
karis hutcheson
7,036 PointsThe problem you're having is that he's requesting a nested list. So it should output something like: [ ["kenneth love", 5], ["jason seifer", 3] ]. You were trying to create a list of names and numbers stored in tuples. However, I don't believe that you can nest the append method that way, either. Here's how I solved the problem:
def stats(dic):
big_list = []
for key in dic:
little_list = []
little_list.append(key)
little_list.append( len(dic[key]) )
big_list.append(little_list)
return big_list
For every entry in the dictionary, I created a new list and appended the name and, then, the number of classes. Lastly, I appended the smaller list to the larger one. You could probably condense this a little but I wanted it to be easy to read.

Frank Pizzuta
Courses Plus Student 4,834 PointsGot this one working (but stuck on last question):
def stats(my_dict): my_list = [] for key in my_dict: num_classes = len(my_dict[key]) my_list.append([key,num_classes]) return my_list
The number of classes is simply the length of the values associated with each key. Then append the key with the length value to a temp list and return the result. I think i could combine the two lines in the for loop but I kept them separate to make it easier to read and understand. Hope this helps.
Piraveen Partheepan
Full Stack JavaScript Techdegree Student 1,130 PointsPiraveen Partheepan
Full Stack JavaScript Techdegree Student 1,130 Pointswhat does teacher.get do