Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial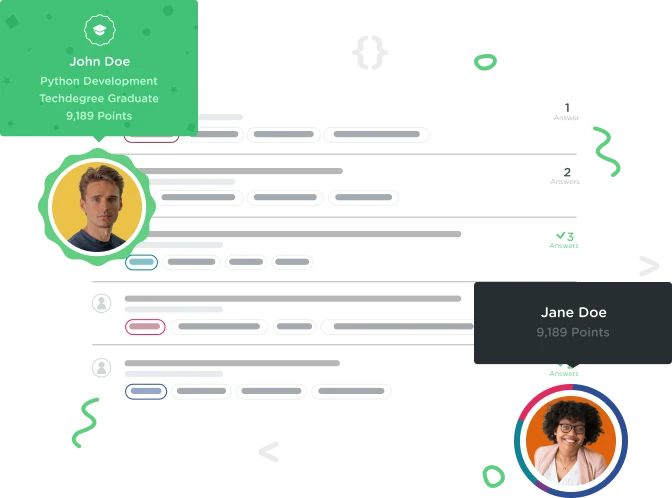

Bhargav Chava
1,706 PointsThis challenge is similar to an earlier one. Remember, though, I want you to practice! You'll probably want to use try a
This challenge is similar to an earlier one. Remember, though, I want you to practice! You'll probably want to use try and except on this one. You might have to not use the else block, though. Write a function named squared that takes a single argument. If the argument can be converted into an integer, convert it and return the square of the number (num ** 2 or num * num). If the argument cannot be turned into an integer (maybe it's a string of non-numbers?), return the argument multiplied by its length. Look in the file for examples.
def squared(num):
try:
c=int(num)
return c*2
except:
l=len(num)
return l*num
1 Answer
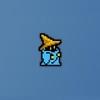
alekseibingham
4,491 Pointsallow me to show you the answer AND explain it so i dont leave you confused!
def squared(argument):
try:
number = int(argument)
return(number * number)
except ValueError:
return(argument * len(argument))
We need a try: because the probblem states that there will be 2 possible answers, one for if it can be converted to an int and one if it cannot. Then the except ValueError is for incase it dose not workout with turning the arugment into an int. I stored the argument after turning it into an int in a variable called number, to make life easy, all we have to do is return number squared!
as for multiplying the argument by its length if it cannot be turned into an int is pretty self explanitory, arugment * len(argument) if you dont know, len is the length of what ever follows!
hope this helps and if it does please mark this down as best answer!

ryan smith
687 PointsWhy wouldn't something like this work?
def squared(argument):
try:
if argument == int(argument):
return(argument * argument)
except ValueError:
return(argument * len(argument))
Nthulane Makgato
Courses Plus Student 19,602 PointsNthulane Makgato
Courses Plus Student 19,602 PointsYou need two asterisks, rather than one, in order to signal that you are squaring the variable "c":
return c**2