Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial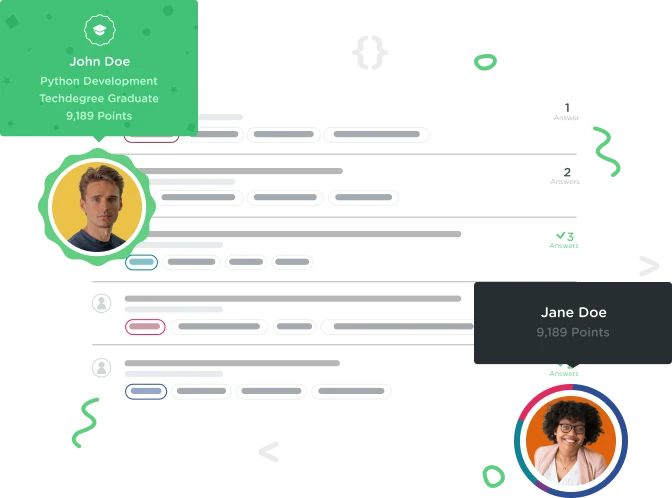

Dylan Ryan
4,855 PointsThis code works, Just wondering how viable the if/else is inside of the for in loop. Is there a reason not to do this?
for(i=0; i<students.length; i+= 1)
{
for(var prop in students[i]){
if(prop === "Name"){
print("<p><strong>Student: " + students[i].Name + "</strong></p>");
} else{
print("<p>" + prop + ": " + students[i][prop] + "</p>");
}
}
}
Like i said in the question, when i test this along with my students array, it works fine. But i was wondering if any problem could arouse when using an if/else within a for in.
2 Answers
Seth Shober
30,240 PointsHi Dylan,
What you are doing is completely normal, and it's good you are being aware of this, as it can eventually become unmaintainable and inefficient to nest multiple times, but you have only nested once. I think what you are asking is, "Is this acceptable code, or is there a better way to do it?"
Generally, the first thing you want to do is get it to work. That is always the top priority. After that you might choose to do some refactoring to either make the code more maintainable and readable or to optimize for speed. If you're lucky you'll get both. The only thing I might do here is call a function in your loop to make it more readable. I don't see a need to filter since it appears you want to do something for each item in the array. Most of the built in array methods just call a for loop for you anyway underneath the hood. If you are interested in optimizing you will like jsperf. Not much has changed here, but a quick example:
var seth
, students
seth = {
Name: "Seth",
Status: "average-joe",
Work: "New Relic"
}
students = [seth]
function logStudents () {
for ( var prop in students[i] ) {
if ( prop == "Name" ) console.log( "<p><strong>Student: " + students[i].Name + "</strong></p>" )
else console.log( "<p>" + prop + ": " + students[i][prop] + "</p>" )
}
}
for ( var i = 0; i < students.length; i++) {
logStudents()
}
Hope this was helpful!
Cheers.
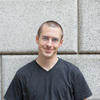
Nathan Brenner
35,844 PointsWhat do you mean by 'viable'? I think it's pretty common to loop through an array and use a conditional statement to find something specific. It would probably be cleaner to use array.indexOf() or array.filter().