Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial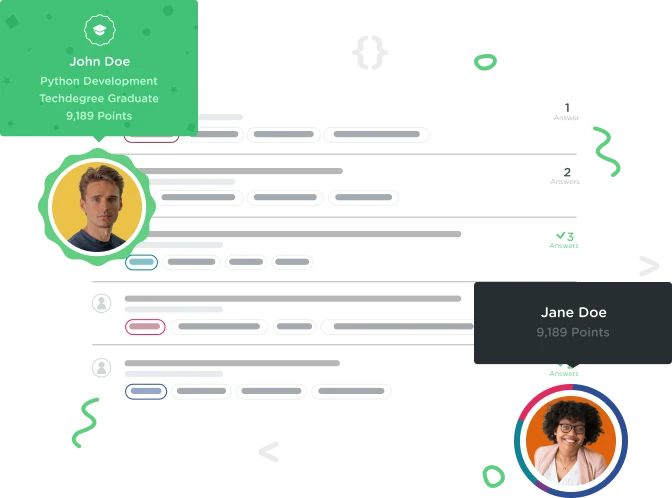

Hoessein Abd
Python Web Development Techdegree Graduate 19,107 PointsThis is how I did the reverse game! I want to add 1 more functionality. Can anyone give me a tip?
I made a loop. In the loop a random_number will be generated and tested if it is equal to my secret_number argument. If the guess is wrong I print out the random_number that the computer guessed and whether it is higher or lower than my secret_number. How can I depending on the first iteration make the computer guess a lower/higher random_number than the random_number in the next iteration?
import random
def game(secret_number):
guesses_left = 5
print("Hey computer, can you beat me by guessing the nummer I have in mind? You will receive {} guesses, good luck!".format(guesses_left))
while guesses_left > 1:
random_number = random.randint(1, 10)
if random_number == secret_number:
print("Yo computer, {} is the correct answer! Good job!".format(random_number))
break
elif random_number < secret_number:
guesses_left -= 1
print("{} is lower than what I have in mind, try again! You have {} guesses left.".format(random_number, guesses_left))
else:
guesses_left -= 1
print("{} is higher than what I have in mind, try again! You have {} guesses left.".format(random_number, guesses_left))
else:
print("Game over! You didn't guess the number in the amount of guesses available.")
play_again = input("Fancy another game? Enter yes or no: ")
if play_again.lower() == "yes":
game(6)
else:
print("Okay! Have a nice day!")
game(6)
1 Answer
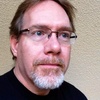
Chris Freeman
Treehouse Moderator 68,441 PointsOne way would be to track the range of targeted guesses with variables, such as, lower_limit
and upper_limit
.
- Initially set these values to the limits of your initial range: 1 and 10
- Then replace the random guess to use these limits:
random_number = random.randint(lower_limit, upper_limit)
- Finally, based on the guess being too low or too high, use the previous guess to update the appropriate value of
lower_limit
orupper_limit
Post back if you have more questions. Good luck!!
Hoessein Abd
Python Web Development Techdegree Graduate 19,107 PointsHoessein Abd
Python Web Development Techdegree Graduate 19,107 PointsDo I need to declare the lower and upper like this: lower_limit = 1 upper_limit = 10
then : random_number = random.randint(lower_limit, upper_limit)
Isn't that the same as passing 1, 10? I can't see how it will give me a lower or higher random number?
Also I have no idea how i can apply your third bulletpoint. Specifically I don't know how I can do something else with the next iteration.
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsDo I need to declare the lower and upper like this: lower_limit = 1 upper_limit = 10 then : random_number = random.randint(lower_limit, upper_limit)
. Correct.Isn't that the same as passing 1, 10? I can't see how it will give me a lower or higher random number?
Be sure to place the original assignment tolower_limit
andupper_limit
outside of the loop. That way as these limits get changed, the random number will too.Also I have no idea how i can apply your third bulletpoint. Specifically I don't know how I can do something else with the next iteration.
If the random_number is less that the secret number setlower_limit = random_number
. If the random number is higher, sethigher_limit = random_number
.Hoessein Abd
Python Web Development Techdegree Graduate 19,107 PointsHoessein Abd
Python Web Development Techdegree Graduate 19,107 PointsAwesome, it worked. the logic is still a bit vague to me though. I'm not sure how a lower/higher number is generated depending on the previous guess.
The random_number sometimes gives the same number but less/higher than the previous number. so i changed the operater accordingly.
thanks for the help!