Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial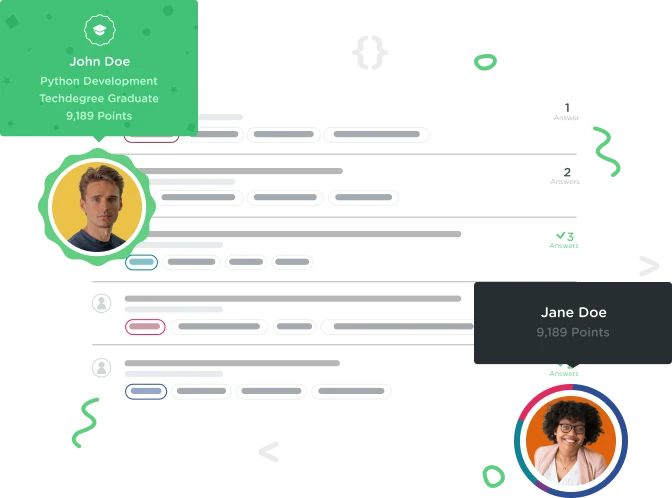

Prisca Egbua
1,167 PointsThis is my code so far but it has a bug which I have not been able to fix. I state this bug in the comment.
I am trying to create a function disemvowel that can remove the vowel from any iterable. Please, any suggestion on how to fix this bug? THANKS
""" the bug is that when more than one vowel appear consequently in a word,
it picks the first and skip the second vowel """
def disemvowel(word):
word = word.lower()
print(word)
word=list(word)
vowel = ['a','e','i','o','u']
for letter in word:
for sound in vowel:
if letter == sound:
word.remove(letter)
print(vowel)
word = "".join(word)
print(word)
1 Answer

Kent Åsvang
18,823 PointsSo this is what is happening - I'll show you my debugging process:
-
First I added print-statements to check exactly how your algorithm worked:
def disemvowel(word): word = word.lower() word=list(word) vowels = ['a','e','i','o','u'] for letter in word: print("Checking %s" % letter) for vowel in vowels: print("checking vowel %s against letter %s" % (vowel, letter)) if letter == vowel: print("%s is in %s" % (letter, vowel)) word.remove(letter) word = "".join(word) print("word that remains is %s" % word) disemvowel("casaa")
And the output was:
```terminal
Checking c
checking vowel a against letter c
checking vowel e against letter c
checking vowel i against letter c
checking vowel o against letter c
checking vowel u against letter c
Checking a
checking vowel a against letter a
a is in a
checking vowel e against letter a
checking vowel i against letter a
checking vowel o against letter a
checking vowel u against letter a
Checking a
checking vowel a against letter a
a is in a
checking vowel e against letter a
checking vowel i against letter a
checking vowel o against letter a
checking vowel u against letter a
word that remains is csa
When you look through the output, you see that each time you remove a letter, your algorithm skips checking the next letter because the queue gets skewed - since you are changing the list that gets iterated through.
With that in mind, I created this algorithm:
def disemvowel(word):
""" prints any given string without its vowels """
vowels = ["a", "e", "i", "o", "u"]
word = list(word.lower())
no_vowels = ""
for letter in word:
if letter not in vowels:
no_vowels += letter
print(no_vowels)
Hope this helped
Prisca Egbua
1,167 PointsPrisca Egbua
1,167 PointsThank you, it did help. After understanding your debugging process, I made a copy of the word and remove the vowel from the copy, so that the queue is not skewed.
def disemvowel(word): word = word.lower() word=list(word) word_copy = word[:] vowel = ['a','e','i','o','u'] for letter in word: for sound in vowel: if letter == sound: word_copy.remove(letter) word = "".join(word_copy) print(word)
Kent Åsvang
18,823 PointsKent Åsvang
18,823 PointsThat's a nice solution.