Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial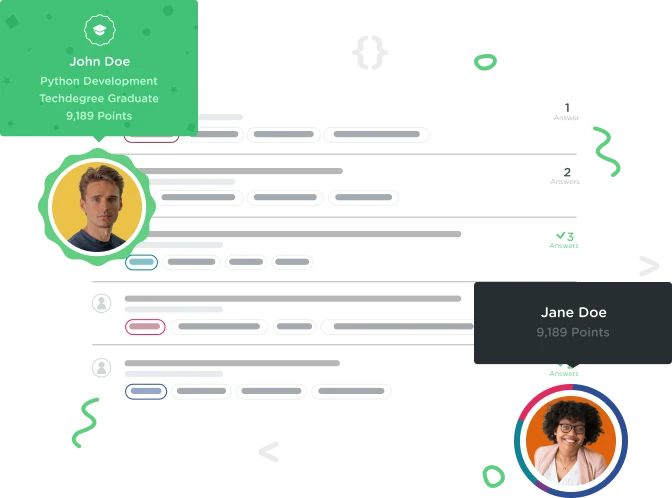

Vladimir Klindić
19,262 PointsThis is my solution.
Is it good enough? Are there too many lines of code? It does the work, but I wonder is it simple and clean enough?
var question1 = prompt("What is the capital of France?");
var question2 = prompt("What is the capital of Germany?");
var question3 = prompt("What is the capital of Belgium?");
var question4 = prompt("What is the capital of Italy?");
var question5 = prompt("What is the capital of Spain?");
var answer1 = 'paris';
var answer2 = 'berlin';
var answer3 = 'brussels';
var answer4 = 'rome';
var answer5 = 'madrid';
var score = 0;
if(question1.toLowerCase() == answer1) {
score += 1;
}
if(question2.toLowerCase() == answer2) {
score += 1;
}
if(question3.toLowerCase() == answer3) {
score += 1;
}
if(question4.toLowerCase() == answer4) {
score += 1;
}
if(question5.toLowerCase() == answer5) {
score += 1;
}
if (score == 5) {
document.write("You've earned a Gold crown!");
} else if (score == 4 || score == 3) {
document.write("You've earned a Silver crown!");
} else if (score == 2 || score == 1) {
document.write("You've earned a Bronze crown!");
} else {
document.write("You have to do it better next time!");
}
4 Answers

Thomas Fildes
22,687 PointsHi Vladimir,
The only things I can suggest doing to decrease the amount of code is to delete the answer variables and add strings to the if statements as a replacement. Also you could use the '>=' parameter instead of the '||' Here is the code below to show you what I mean if you are unsure.
if ( question1.toLowerCase() === 'paris' ) {
score += 1;
}
if ( question2.toLowerCase() === 'berlin' ) {
score += 1;
}
Doing this above for every if statement the outcome will be the same and simplifies your application.
if ( score == 5 ) {
document.write("You've earned a Gold crown!");
} else if ( score >= 3 ) {
document.write("You've earned a Silver crown!");
} else if ( score >= 1 ) {
document.write("You've earned a Bronze crown!");
} else {
document.write("You have to do it better next time!");
}
The else if statement containing the '>=' shown above will work as JavaScript will run the statements in order so if they score 3 it works for the first else if statement and will run that. If they get 1 right then the script skips the if and first else if statement and runs the next else if.
Happy Coding!

Anjali Pasupathy
28,883 PointsThis code looks like it would be a bit more DRY (Don't Repeat Yourself) if you used arrays and a for loop. I constructed a solution on the fly in the console, and it seems to function fine. I also reworded your final if-else statements at the end to make the conditions a bit more compact, and to make the code a bit more DRY.
var countries = ["France", "Germany", "Belgium", "Italy", "Spain"];
var answers = ["paris", "berlin", "brussels", "rome", "madrid"];
var score = 0;
var question = "";
for (i = 0; i < countries.length; i++) {
question = prompt("What is the capital of " + countries[i] + "?");
if (question.toLowerCase() == answers[i]) {
score += 1;
}
}
var message = "You've earned a ";
if (score == 5) {
message += "Gold crown!";
} else if (score > 2) {
message += "Silver crown!";
} else if (score > 0) {
message += "Bronze crown!";
} else {
message = "You have to do it better next time!";
}
document.write(message);
I hope this helps!

Vladimir Klindić
19,262 PointsThanks for your answers and suggestions :)

Nathan Crum
15,183 PointsIn addition to arrays you could also use Embedded Expressions to combine some of the logic into the prompts and reduce your code as well:
Here is my solution with the comments or you can see below with just the code.
var questions = ["What is your dogs name?","What is your favorite food?","What is your mothers maiden name?","Where is your hometown?","What is your daughters middle name?"];
var answers = ["Sam","Pizza","Terry","Wilmore","Grace"];
var score = 0;
var achievemnts = ["Wooden Crown", "Bronze Crown", "Silver Crown", "Gold Crown", "Platinum Crown"];
for(var i = 0; i < 5; i++) {
var guess = prompt(`${i+1} of 5: ` + questions[i])
if(guess.toLowerCase() === answers[i].toLowerCase()) {
score++
alert(`Correct! The answer is ${answers[i]}`);
} else {
alert(`False! The correct answer is ${answers[i]}`);
}
}
alert(`You got ${score} of 5 questions right! You have been awarded the ${achievemnts[(score === 0 ? score : score - 1)]}!`)
Cindy Lea
Courses Plus Student 6,497 PointsCindy Lea
Courses Plus Student 6,497 PointsYou could probably put the questions & answers into an array. Then loop through the indexes to check the answers. If question[0] = answer[0] then its correct, etc. It simplifies the code. If you added more questions later then the code will get more crowded.