Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial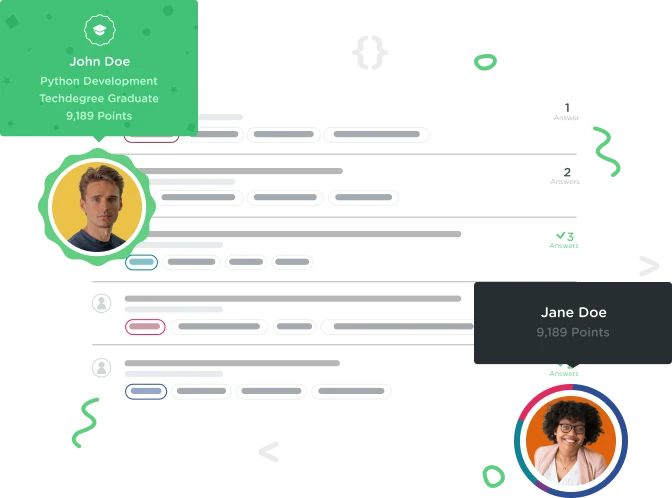

Ilija Marinkovic
6,456 PointsThis is my solution for the quiz game from the JavaScript Basics course.
/*
1. Store correct answers
- When quiz begins, no answers are correct
*/
const bestUmbralMonster = "NIGHTSTALKER"
const eliFavoriteWeapon = "REPEATERS"
const bogdanFavoriteWeapon = "AXE"
const distinguishedGentleman = "ALYRA"
const theNibbler = "DRASK"
let correctAnswers = 0;
// 2. Store the rank of a player
const zero = "Nobodys Slayer"
const baby = "Baby Slayer"
const novice = "Novice Slayer"
const intermediate = "Intermediate Slayer"
const gilded = "Gilded Slayer"
const platinum = "Platinum Slayer"
// 3. Select the <main> HTML element
const main = document.querySelector('main')
/*
4. Ask at least 5 questions
- Store each answer in a variable
- Keep track of the number of correct answers
*/
const question1 = prompt("What is the best Umbral Monster?")
if ( question1.toUpperCase() === bestUmbralMonster ) {
correctAnswers += 1
console.log( correctAnswers )
} else {
console.log( correctAnswers )
}
const question2 = prompt("What is Elijah's favourite weapon?")
if ( question2.toUpperCase() === eliFavoriteWeapon ) {
correctAnswers += 1
console.log( correctAnswers )
} else {
console.log( correctAnswers )
}
const question3 = prompt("What is Bogdan's favourite weapon?")
if ( question3.toUpperCase() === bogdanFavoriteWeapon ) {
correctAnswers += 1
console.log( correctAnswers )
} else {
console.log( correctAnswers )
}
const question4 = prompt("Who does Elijah refer to as a 'distinguished gentleman'?")
if ( question4.toUpperCase() === distinguishedGentleman ) {
correctAnswers += 1
console.log( correctAnswers )
} else {
console.log( correctAnswers )
}
const question5 = prompt("Who does Elijah mock for his bites?")
if ( question5.toUpperCase() === theNibbler ) {
correctAnswers += 1
console.log( correctAnswers )
} else {
console.log( correctAnswers )
}
/*
5. Rank player based on number of correct answers
- 5 correct = Gold
- 3-4 correct = Silver
- 1-2 correct = Bronze
- 0 correct = No crown
*/
if ( correctAnswers === 0 ) {
main.innerHTML = `<h3>Uh.. do you.. know ANYTHING ABOUT Dauntless?</h3t>
<h2>Your rank is: <strong>${zero}</strong></h2>`
} else if ( correctAnswers === 1 ) {
main.innerHTML = `<h3>Well, at least you knew something!</h3t>
<h2>Your rank is: <strong>${baby}</strong></h2>`
} else if ( correctAnswers === 2 ) {
main.innerHTML = `<h3>Decent! Think you can brush up a bit?</h3t>
<h2>Your rank is: <strong>${novice}</strong></h2>`
} else if ( correctAnswers === 3 ) {
main.innerHTML = `<h3>You were honestly a real one..!</h3t>
<h2>Your rank is: <strong>${intermediate}</strong></h2>`
} else if ( correctAnswers === 4 ) {
main.innerHTML = `<h3>Sick! You're pretty knowledgable.</h3t>
<h2>Your rank is: <strong>${gilded}</strong></h2>`
}
else if ( correctAnswers === 5 ) {
main.innerHTML = `<h3>Damn! You knew EVERYTHING! It's like you made this..</h3t>
<h2>Your rank is: <strong>${platinum}</strong></h2>`
}
// 6. Output results to the <main> element -- Already done above.