Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial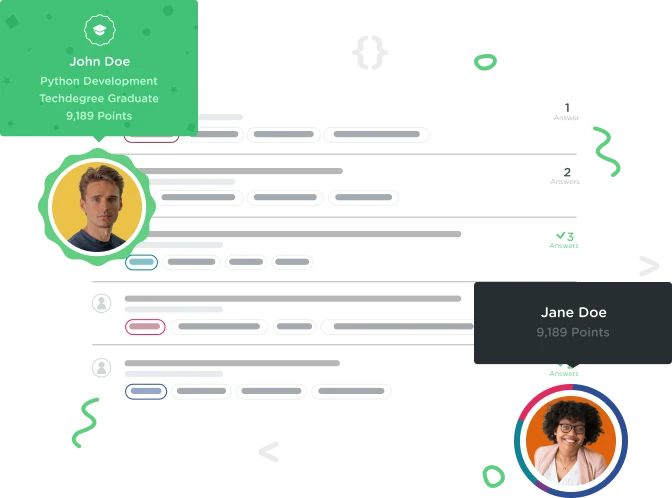
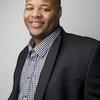
Sahmad Nakumbe
3,423 PointsThis is the code we used in the last code challenge. After learning about do...while loops, don't you think this would w
Need help?
do ( secret !== "sesame" ) {
secret = prompt("What is the secret password?");
}
while ( "You know the secret password. Welcome." );
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
3 Answers
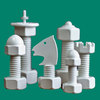
Steven Parker
241,954 PointsIn a "do...while" loop, the conditional expression still follows the "while". There's nothing between "do" and the brace that starts the code block. And a couple more hints:
- "secret" will need to be declared (but not assigned) before the loop starts
- the line to write the message on the page should be left as it was originally
UPDATE: Perhaps comments directly in the code would be more clear:
var secret; // declare but don't assign
do { // nothing between "do" and brace
secret = prompt("What is the secret password?");
} while ( secret !== "sesame" ); // the test goes with the "while"
document.write("You know the secret password. Welcome."); // this line stays the same

Ross Kendall-Selwyn
12,813 PointsHi Sahmad,
Before writing your 'do.. while' function, you'll need declare the secret variable (without assigning it).
Then using "do", your function will run the prompt when you pass it "secret = prompt()".
Then you'll need to write your 'While' function so that "While (secret does not equal sesame") it loops the prompt.
I hope this helps a little, feel free to contact me if you need clarification :) Happy coding!
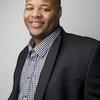
Sahmad Nakumbe
3,423 PointsExplain?

Ross Kendall-Selwyn
12,813 PointsOk so.. i'll try my best.
var secret; By not assigning the variable secret, you're able to set it equal to the prompt answer which is the same as the prompt being equal to secret. If you don't create a variable called secret the function has nothing to check an input against.
the do function asks the user for a prompt: do (secret = prompt{"what is the secret password"}); so it's saying do this prompt and take an input from the user, then set the users input to equal secret. replacing the prompt with the input.
This is the equivalent to : do (secret = user input);
the while part of the function is saying: while (secret !== 'sesame'); while secret is not equal to 'sesame' run the loop over and over until it is.
The function needs to take the users input before it can check that is or isn't equal to 'sesame'.
once the function has checked the user has entered an input, and that input is equal to sesame it will then write to the document "You know the secret password. Welcome.".
The code you've posted above is saying: do: secret does not equal sesame. But it has nothing to check against no defined variable or input. You've also made the answer part of the loop, but you need it set so once the loop is finished it then writes the answer.
Let me know if this clears things up a little for you, this is a tricky one!