Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial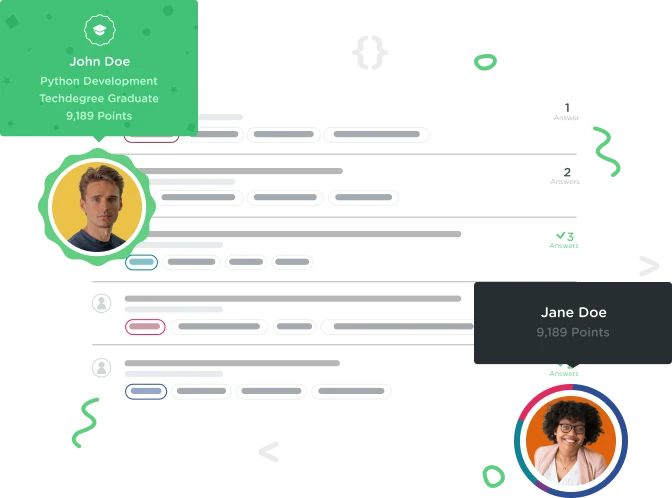

Kenton Hubner
6,314 PointsThis may be a little long, but it works! I do have one question though.
I tried to add .toUpperCase() in the if statements so I could save time writing out the many different variations of how someone may answer but was unsuccessful. I attempted to make a var with the answer to upper case such as
var one = 'blue'.toUpperCase();
but that did not work. Also I tried it in the conditional statement as in:
firstQuestion === 'blue'.toUpperCase()
which did not work as well. Any pointers on that?
Here is the code:
/*
Simple quiz application:
1. Ask at least 5 questions.
2. Keep track of answers correct
3. let user know # of questions right
4. Rank the player
a. all 5 right, Gold Crown
b. 3-4 right, Silver Crown
c. 1-2 right, Bronze Crown
d. 0 right, No crown at all
*/
// Questions to be asked
var firstQuestion = prompt('What color is the sky?');
var secondQuestion = prompt('What day of the week is christmas on?');
var thirdQuestion = prompt('What is the capitol of Texas?');
var fourthQuestion = prompt('Who is Ned Starks bastard son?');
var fifthQuestion = prompt('Is JavaScript a good tool for websites?');
var correct = 0;
var score = 0;
//Checks multiple variations of answers and adds a point if correct or none if wrong
if (firstQuestion === 'Blue' || firstQuestion === 'BLUE' || firstQuestion === 'blue') {
score += 1;
correct += 1;
} else {
score += 0;
correct += 0;
}
console.log(score);
//Checks multiple variations of answers and adds a point if correct or none if wrong
if (secondQuestion === '25' || secondQuestion === 25 || secondQuestion === 'twenty five' || secondQuestion === 'Twenty Five' || secondQuestion === 'TWENTY FIVE') {
score += 1;
correct += 1;
} else {
score += 0;
correct += 0;
}
console.log(score);
//Checks multiple variations of answers and adds a point if correct or none if wrong
if (thirdQuestion === 'Austin' || thirdQuestion === 'austin' || firstQuestion === 'AUSTIN') {
score += 1;
correct += 1;
} else {
score += 0;
correct += 0;
}
console.log(score);
//Checks multiple variations of answers and adds a point if correct or none if wrong
if (fourthQuestion === 'John Snow' || fourthQuestion === 'john snow' || fourthQuestion === 'JOHN SNOW') {
score += 1;
correct += 1;
} else {
score += 0;
correct += 0;
}
console.log(score);
//Checks multiple variations of answers and adds a point if correct or none if wrong
if (fifthQuestion === 'yes' || fifthQuestion === 'Yes' || fifthQuestion === 'YES') {
score += 1;
correct += 1;
} else {
score += 0;
correct += 0;
}
console.log(score);
// check score count to award Crown
if (score === 5 && correct === 5) {
document.write('Congrats, you received a Gold Crown for answering all ' + correct + ' questions correctly!');
} else if (score === 4 || score === 3 && correct === 4 || correct === 3) {
document.write('Congrats, you received a Silver Crown for getting ' + correct + ' questions correct!');
} else if (score === 2 || score === 1 && correct === 2 || correct === 1){
document.write('Congrats, you received a Silver Crown for getting ' + correct + ' questions correct!');
} else {
document.write('I am sorry, you answered ' + correct + ' questions right. Thank you for playing!');
}

Warren Amos
4,530 PointsTry this out. Sorry if its hard to read. I edited your code in my own editor and just pasted into here.
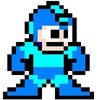
Robert Richey
Courses Plus Student 16,352 PointsHi Warren,
I added markdown to your code, to help make it more readable.
Cheers

Warren Amos
4,530 PointsThanks Robert.
2 Answers

Kenton Hubner
6,314 PointsThanks! I saw on the next video that I was applying it towards the wrong part of the code as it should have been applied to the variable. Also I saw that my score and answer were sort of redundant and that truly I only needed to use one of those.

Nicholas Woods
6,260 PointsI'm not sure if you were doing it to just play with the 'and operator' (&&), but isn't having the variables 'score' and 'correct' together redundant when one can achieve the same result? Not asking to be rude, just curious as to why you chose this.
Warren Amos
4,530 PointsWarren Amos
4,530 Points