Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial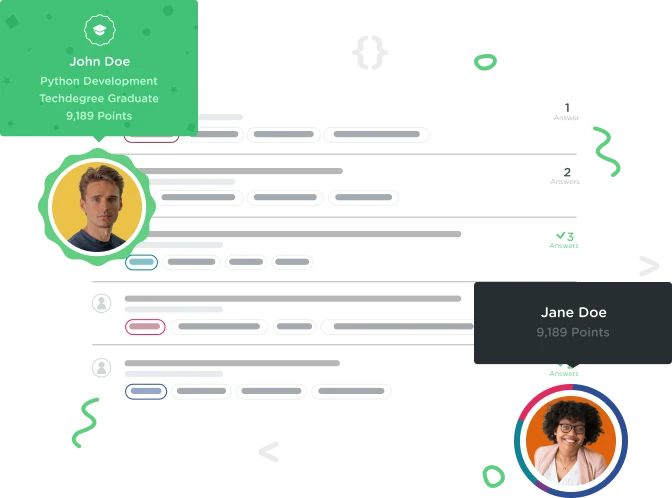

Krzysztof Jędras
Courses Plus Student 3,365 PointsThis one solves a thing even if first or second number is higher than another ;)
Hi everyone, I believe it will return undefined if we give a number2 higher value than number1, so I wrote a code to make it clear. Tell me if I'm right or not. I'm total beginner with any programming language.
// give name to variables but, we recieve it as a string from a user
var number1string = prompt('Please select any number between 0 and 100');
var number2string = prompt('Please select any number between 0 and 100');
//convert string to numbers
var number1 = parseInt(number1string);
var number2 = parseInt(number2string);
//create a function that gives a random number
function randomNumber(number1, number2) {
// if statement is to select a higher number automaticly, even if number1 is higher than number2
if (number1 >= number2){
return Math.floor(Math.random() * (number1 - number2 + 1)) + number2
}
else if (number1 <= number2) {
return Math.floor(Math.random() * (number2 - number1 + 1)) + number1
}
}
// print a function to a user
alert('the random number is ' + randomNumber(number1, number2));
3 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Krzysztof,
It looks like that would work out fine.
I wanted to mention that you won't get undefined if the numbers are in the wrong order but you won't get the correct range either.
This is just a suggestion but I think it would be better to make the correction after you get the numbers from the user. I also think it makes the code and formula more readable if you use names like lower
and upper
or even min
and max
It would also make your randomNumber function cleaner because you would only have the one return statement.
Another thing to think about is what if the program is bigger and there's another part where it's important to know which number is bigger. You'll have to repeat that same kind of conditional checking that you have in your randomNumber function.
If you make the correction once at the beginning then the rest of your program can use these 2 variables and you'll always know which one is bigger.
Something like this:
var max = parseInt(prompt('Please select a maximum number'));
var min = parseInt(prompt('Please select a minumum number'));
// check if the user entered them in the wrong order
if (max < min) {
// swap the 2 values so that max is the bigger number
}
// Now the rest of your program doesn't need to do any checking on which number is bigger.
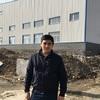
ali mur
UX Design Techdegree Student 23,377 Pointsvar number1string = prompt('Please select any number between 0 and 100');
var number2string = prompt('Please select any number between 0 and 100');
//convert string to numbers
var num1 = parseInt(number1string);
var num2 = parseInt(number2string);
if (num1>num2){
var result=Math.floor(Math.random() * (num1-num2)) + num2;
alert(result);
}
else if(num1<num2){
var result=Math.floor(Math.random() * (num2 - num1)) + num1;
alert(result);
}

Krzysztof Jędras
Courses Plus Student 3,365 PointsPretty much the same, but you stored random number in variable called result. And according to task we need to make a function.

Caleb Brewster
3,566 PointsI believe it doesn't matter either way. If you switch around the higher and lower numbers and subtract them, you will end up with a negative integer. When adding that negative integer to your higher number, it will subtract the random number from the higher number, producing the same result as if you were adding the positive integer to the lower one.

Jason Anello
Courses Plus Student 94,610 PointsHi Caleb,
I recommend that you try it out with some numbers and I think you'll find that you don't get the correct range when they're in the wrong order.
You could try it with 3 and 5 for example. When you put them into the formula the correct way, you will get a roughly equal distribution of the integers 3, 4 and 5.
If you put the endpoints into the formula in the wrong order, then you'll find that you can never get a 3. You'll get 4 most of the time and on rare occasion you'll get a 5.