Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial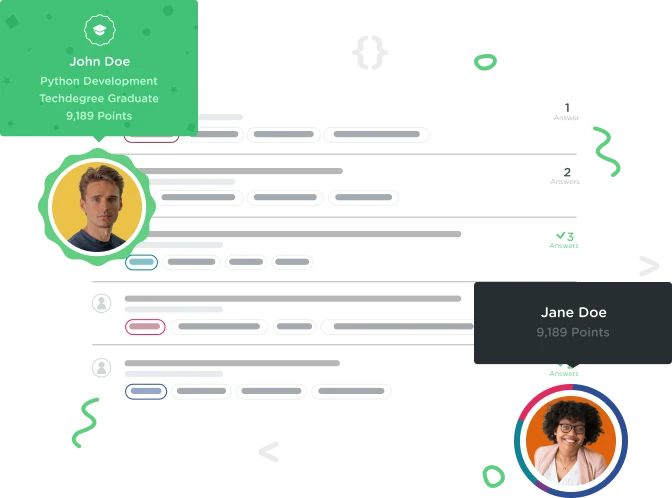

Pat Dudar
2,490 Pointstrial.py insert issues
Hi, there! I'm trying to give the argument values x, y the option of being interpreted as strings or integers in my code under a try: statement (see below).
Can this be accomplished with int or str as the name of the function? I don't think this is too complicated, I just think i'm missing something basic. Thanks for your help!
def add(x, y):
try:
x = int(insert(""))
y = int(insert(""))
except ValueError:
a = float(x)
b = float(y)
return(a + b)
3 Answers
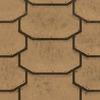
Wesley Trayer
13,812 PointsI'm sorry if I don't understand your question, but your goal, as stated in the quiz, is to Try to turn "x" and "y" into floats.
try:
a = float(x)
b = float(y)
# More code goes here
return a + b
Hope this helps! :~)

Pat Dudar
2,490 PointsHi, Wesley. The main issue is in question three of the quiz regarding the try: block. I'm not sure whether I need to use string or integer to figure out what I'm doing wrong. Again I think I'm overcomplicating it
Challenge Task 3 of 3 You're doing great! Just one more task but it's a bigger one. Right now, we turn everything into a float. That's great so long as we're getting numbers or numbers as a string. We should handle cases where we get a non-number, though. Add a try block before where you turn your arguments into floats. Then add an except to catch the possible ValueError. Inside the except block, return None. If you're following the structure from the videos, add an else: for your final return of the added floats.

Pat Dudar
2,490 PointsHere is my script:
def add(x, y): try: a = float(x) b = float(y) except ValueError: return None else: return(a + b)
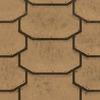
Wesley Trayer
13,812 PointsSorry I took a while to reply.
It looks like your script is correct, at least, I don't see anything wrong. But here is my code, perhaps you can spot a difference.
def add(x, y):
try: # This "try" will check to see if "x" and "y" can be converted to floats
a = float(x) # "trying" to assign a float equal to "x" to "a"
b = float(y) # "trying" to assign a float equal to "y" to "b"
except ValueError: # If there is a ValueError, eg. x or y is not an integer then...
return None # return None
else: # If there is no ValueError...
return a + b # return a + b, which are equal to the floats of x and y.