Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial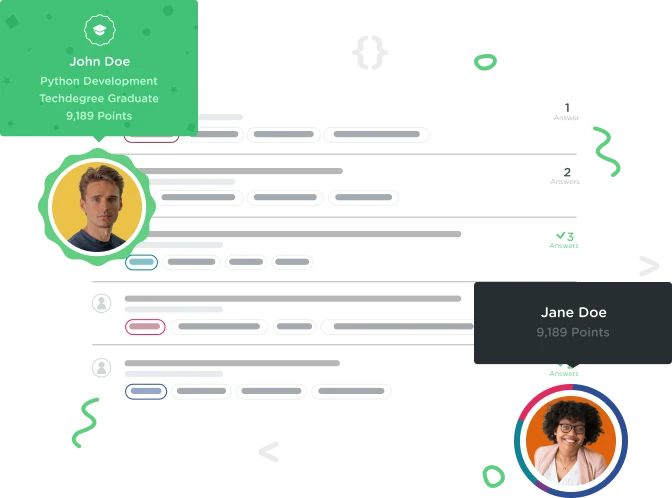

Jvalant Dave
4,483 PointsTrouble retrieving JSON Data?
I've triple checked my code for any syntax errors and matched it with the code provided under the video. Everything seems fine but when I run the app, the alertUserAboutError dialog is called and I can't seem to figure out why. I've tried running it on my phone as well as the simulator and both times, the dialog comes up.
I also created a new account with Forecast.io and used a new API key, no change. I've checked the Android Manifest and I have entered the permissions for INTERNET and ACCESS_NETWORK_STATE.
Here is my code for the main activity:
public class MainActivity extends AppCompatActivity {
public static final String TAG = MainActivity.class.getSimpleName();
private CurrentWeather mCurrentWeather;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
String apiKey = "c52e1d1ae23ca2a0b45a95023f752699";
double latitude = 37.8267 ;
double longitude = -122.423;
String forecastUrl = "https://api.forecast.io/forecast/" + apiKey + "/" + latitude + longitude;
if(isNetworkAvailable()) {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(forecastUrl)
.build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
}
@Override
public void onResponse(Call call, Response response) throws IOException {
try {
String jsonData = response.body().string();
Log.v(TAG, jsonData);
if (response.isSuccessful()) {
mCurrentWeather = getCurrentDetails(jsonData);
} else {
alertUserAboutError();
}
}
catch (IOException e) {
Log.e(TAG, "Exception caught: ", e);
}
catch (JSONException e) {
Log.e(TAG, "Exception caught: ", e);
}
}
});
}
else {
Toast.makeText(this, R.string.network_unavailable_message, Toast.LENGTH_LONG).show();
}
Log.d(TAG, "Main UI code is running ");
}
private CurrentWeather getCurrentDetails(String jsonData) throws JSONException {
JSONObject forecast = new JSONObject(jsonData);
String timezone = forecast.getString("timezone");
Log.i(TAG, "From JSON: " + timezone);
return new CurrentWeather();
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager) getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if (networkInfo != null && networkInfo.isConnected()){
isAvailable = true;
}
return isAvailable;
}
private void alertUserAboutError() {
AlertDialogFragment dialog = new AlertDialogFragment();
dialog.show(getFragmentManager(), "error_dialog");
}
}
2 Answers

aakarshrestha
6,509 PointsYou need to change String forecastUrl = "https://api.forecast.io/forecast/" + apiKey + "/" + latitude + longitude;
To
String forecastUrl = "https://api.forecast.io/forecast/" + apiKey + "/" + latitude + ", " + longitude;
It should work.
Happy coding!

aakarshrestha
6,509 PointsGlad it helped!
Happy coding!
Jvalant Dave
4,483 PointsJvalant Dave
4,483 PointsThat did it! I feel silly haha Thanks!