Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial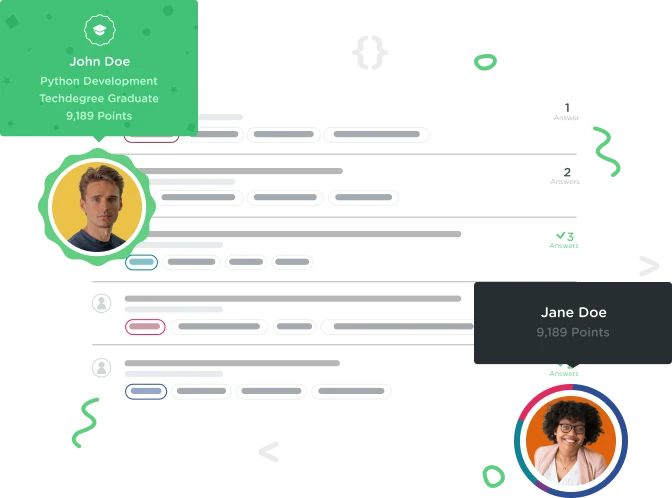

Ronnie Barua
17,665 PointsTrouble solving word_count.
Here is what I did so far
def word_count(sentence): dict_count = {} sentence = sentence.split()
for words in sentence: if words in dict_count: dict_count += 1 else: dict_count = 1
return word_count
# E.g. word_count("I am that I am") gets back a dictionary like:
# {'i': 2, 'am': 2, 'that': 1}
# Lowercase the string to make it easier.
# Using .split() on the sentence will give you a list of words.
# In a for loop of that list, you'll have a word that you can
# check for inclusion in the dict (with "if word in dict"-style syntax).
# Or add it to the dict with something like word_dict[word] = 1.
def word_count(sentence):
dict_count = {}
sentence = sentence.split()
for words in sentence:
if words in dict_count:
dict_count += 1
else:
dict_count = 1
return word_count
7 Answers
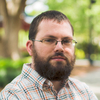
Kenneth Love
Treehouse Guest TeacherYour problem is that you never add the word to dict_count
(and you try to increment the numerical value of a dictionary which doesn't have a numerical value because it's, well, not a number).
def word_count(sentence):
dict_count = {}
sentence = sentence.split()
for words in sentence:
if words in dict_count:
dict_count[words] += 1
else:
dict_count[words] = 1
return word_count
There are still a couple of problems in here (hints: a mis-named variable and some bad indentation), but I'll leave those as problems for you to solve.
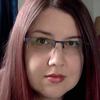
Tree Casiano
16,436 PointsTry eliminating the variable declaration of sentence=sentence.split() and instead use
for words in sentence.split():

Ronnie Barua
17,665 PointsThanks! That's what I did the first time but didn't pass.
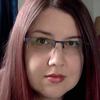
Tree Casiano
16,436 PointsHere's what I used for this challenge. I kept a copy of the code because it stumped me.
def word_count(a_string):
string_dict = {}
for word in a_string.split():
if word in string_dict:
string_dict[word] += 1
else:
string_dict[word] = 1
return string_dict
If you look at my conditional statement, you see that I loop the value of each key and used string_dict[word], which in your code would be dict_count[words]. Also, you need to make sure you are returning the name of your dictionary, not the name of your function.

Ronnie Barua
17,665 PointsThanks a lot. I'll use yours and let you know soon.
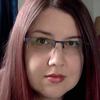
Tree Casiano
16,436 PointsI just got yours to pass by making the changes I noted above and by correcting the indentation.

Ronnie Barua
17,665 PointsThanks you again. It did pass.
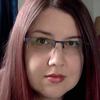
Tree Casiano
16,436 PointsYay! :-)

Ronnie Barua
17,665 PointsThanks for pointing that out. I keep an eye on indentation but it always betrays me for some unknown reason. I did pass. Thank you Mr. Love!