Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial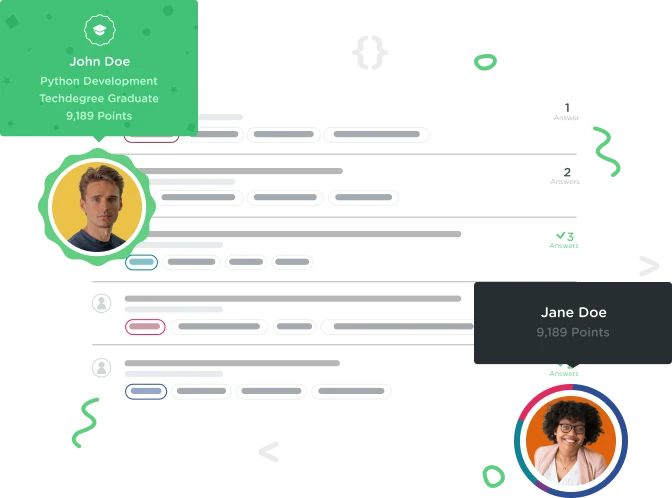

dlpuxdzztg
8,243 PointsTrouble with 'if' statement.
Hello.
I have tried executing this multiple times, but no matter what I do it still doesn't work. I want a DialogFragment to open when the EditText's fields are blank or null:
if (mNoteTitleEdit.equals(null)) {
error();
}
if (mNoteDescriptionEdit.equals(null)) {
error();
}
if (mNoteTitleEdit == null) {
error();
}
if (mNoteDescriptionEdit == null) {
error();
}
These just give me errors:
if (mNoteTitleEdit && mNoteDescriptionEdit.equals(null)) {
error();
}
if (mNoteTitleEdit && mNoteDescriptionEdit == null) {
error();
}
Full code (if necessary):
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment_new_note, container, false);
mNoteTitleEdit = (EditText) view.findViewById(R.id.NoteTitleEdit);
mNoteDescriptionEdit = (EditText) view.findViewById(R.id.NoteDescriptionEdit);
mConfirmImageNN = (ImageView) view.findViewById(R.id.ConfirmImageNN);
mConfirmImageNN.setOnClickListener(this);
mCancelImageNN = (ImageView) view.findViewById(R.id.CancelImageNN);
mCancelImageNN.setOnClickListener(this);
return view;
}
@Override
public void onClick(View v) {
if (v == mConfirmImageNN) {
String titleText = mNoteTitleEdit.getText().toString();
String descriptionText = mNoteDescriptionEdit.getText().toString();
if (titleText.equals(null)) {
error();
}
if (descriptionText.equals(null)) {
error();
}
}
if (v == mCancelImageNN) {
goBack();
}
}
I can't figure out why it gives me an error and why it does nothing with the first two.
Please help!
1 Answer

Seth Kroger
56,416 PointsIf those two member variables, mNoteTitleEdit and mNoteDescriptionEdit, refer to the EditText's themselves they won't be null unless they don't exist on the Activity (or weren't initialized by findViewById() yet) The EditText is the UI widget that takes text input, not the input text itself. If you want the text you would use EditText.getText().toString().
In the last example, mNoteTitleEdit
is just an object reference, not a boolean expression which Java requires in an if statement. Some other languages can use that style of syntax for checking null/empty using truthy/falsey values, but Java is more strict in using only true/false.
dlpuxdzztg
8,243 Pointsdlpuxdzztg
8,243 PointsThanks, but for some reason, even with .getText().toString(), the code still doesn't execute. I edited the post to include a little more code if it helps.
Seth Kroger
56,416 PointsSeth Kroger
56,416 PointsYou should check equality with an empty string, "", instead of null. Null is if the string doesn't exist, but the string can exist and be empty.
dlpuxdzztg
8,243 Pointsdlpuxdzztg
8,243 PointsGot it working, thanks!