Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial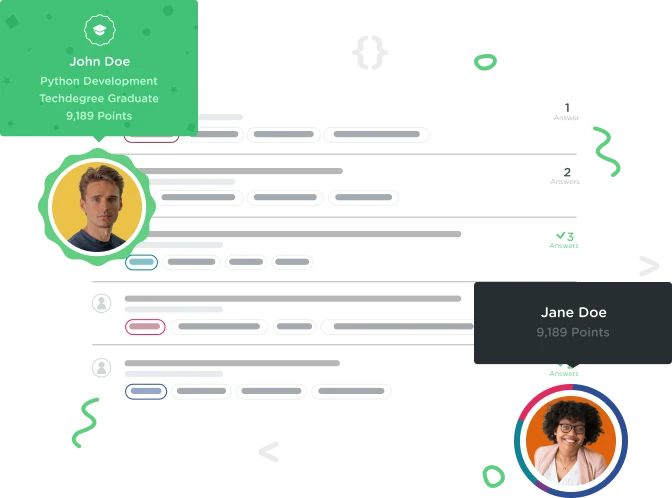

dlpuxdzztg
8,243 PointsTrouble with Replacing Fragment in MainActivity
Hello.
So, I want to replace my fragment in my MainActivity with, of course, another fragment. However, I'm using Interface implementation, I want to replace the fragment using an ImageView, and I'm not using a list. Here is my code for the fragment (MainListFragment) I want to replace:
OnButtonSelectedListener mCallback;
private ImageView mAddItemImage;
public interface OnButtonSelectedListener {
void onButtonSelected(int position);
}
@Nullable
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment_recyclerviewmain, container, false);
mAddItemImage = (ImageView) view.findViewById(R.id.addItemImage);
return view;
}
@Override
public void onClick(View v) {
mCallback.onButtonSelected(mAddItemImage);
}
Here is the code for the MainActivity:
public static final String MAIN_LIST_FRAGMENT = "main_list_fragment";
public static final String NEW_ITEM_FRAGMENT = "new_item_fragment";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
MainListFragment mainListFragment = new MainListFragment();
FragmentManager fragmentManager = getSupportFragmentManager();
FragmentTransaction fragmentTransaction = fragmentManager.beginTransaction();
fragmentTransaction.add(R.id.placeHolder, mainListFragment, MAIN_LIST_FRAGMENT);
fragmentTransaction.commit();
}
@Override
public void onButtonSelected(int position) {
NewItemFragment newItemFragment = new NewItemFragment();
FragmentManager fragmentManager = getSupportFragmentManager();
FragmentTransaction fragmentTransaction = fragmentManager.beginTransaction();
fragmentTransaction.replace(R.id.placeHolder, newItemFragment);
fragmentTransaction.addToBackStack(null);
fragmentTransaction.commit();
}
So where do I go from here? How do I make it so that the data sends properly?
Thank you.
2 Answers

Seth Kroger
56,413 PointsYou won't be able to pass an ImageView directly. Use a Bundle to pass the data representing the image you want, be it a resource id, URI string or even a Bitmap to recreate it in the ImageView of the new fragment.

Seth Kroger
56,413 PointsAhh, then just set an OnClickListener for that ImageView.

dlpuxdzztg
8,243 PointsBut I still want to send data from my fragment to the MainActivity. (Keep in mind that the ImageView is in the fragment I want to replace.)

Seth Kroger
56,413 PointsThe question is what sort of data and are you going to pass that data on to other fragments or components? You should be able to pass an arbitrary object through a callback, though passing a View attached to the fragment can get dicey when you remove the fragment. If you're passing in along, then the data either needs to be a basic type (int, String, etc) or Parcelable. In other words a type you can put into an Intent extra or Bundle argument.

dlpuxdzztg
8,243 PointsThe data I want to send back to the MainActivity is the fragment I want to replace the current fragment with. I used interface implementation because I was told that it's the best way to send data from a fragment to an activity.

Seth Kroger
56,413 PointsIt there is a choice of fragments you could replace it with, you could define constants in MainActivity for each choice and construct the appropriate fragment based of that. You could also pass the fragment's Class through the callback and use the newInstance() method to construct the fragment.

dlpuxdzztg
8,243 PointsI already have multiple constants for each of my fragments, displaying them when I want is the problem. Should I also create constants for the buttons in my fragment?

Seth Kroger
56,413 PointsYou should be able to choose the corresponding constant for each Button or ImageView that was clicked
dlpuxdzztg
8,243 Pointsdlpuxdzztg
8,243 PointsOh, what I meant was I want a certain ImageView to be pressed in order for my new fragment to replace the other.