Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial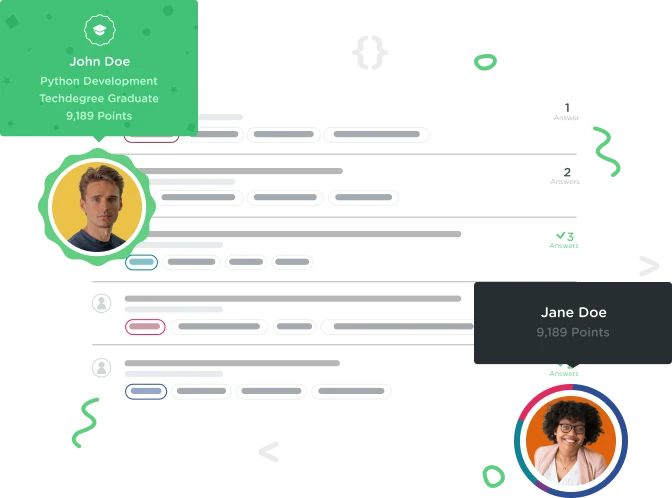

Nitin Gupta
735 PointsTry and Except Challenge
For the last step in the challenge, I have written the below code
def add(num1, num2):
try:
num1 = float(num1)
num2 = float(num2)
except ValueError:
return None
else:
return (num1 + num2)
sum = add(2, 2)
print(sum)
I want to know where I am making a mistake.
3 Answers
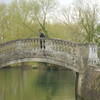
Rufus Mathews
3,653 PointsThis is what I did. Slightly different but still applicable.
def add(num1, num2):
try:
return(float(num1) + float(num2))
except ValueError:
return None
else:
return(float(num1) + float(num2))
hope this helps ;)

Nitin Gupta
735 PointsThanks a lot for the help :-)
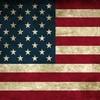
Steven Turturo
6,890 PointsHave you tried indenting your statements for your try and except code blocks?
def add(num1, num2):
try:
num1 = float(num1)
num2 = float(num2)
except ValueError:
return None
else:
return (num1 + num2)
sum = add(2, 2)
print(sum)

Nitin Gupta
735 PointsThanks a lot for the help.
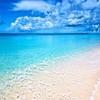
eodell
26,386 Points↓↓This also worked...↓↓
【code challenge 1/3】
def add(x, y): return(x + y)
【code challenge 2/3】
def add(x, y): x = float(x) y = float(y) return(x + y)
【code challenge 3/3】
def add(x, y): try: x = float(x) b = float(y) except ValueError: return None else: return(x + y)
John Simmons
1,247 PointsJohn Simmons
1,247 PointsCheck you indentations...