Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial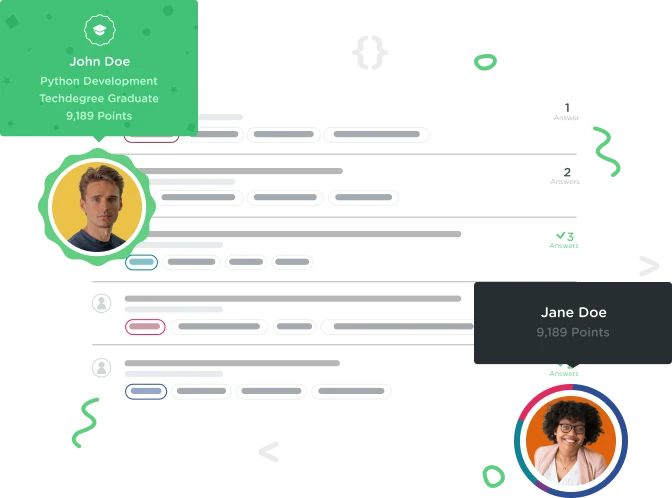

Curtis Crawley
550 PointsTrying to Break code!! When i put in a non-integer in for guess it always comes back as invalid literal for int().
Am I missing something? Heres my code. Please help.
import random
def game():
secret_num = random.randint(1, 10)
guesses = []
while len(guesses) < 5:
try:
guess = int(input("Guess a number between 1 and 10: " ))
except ValueError:
print("{} isn't a number!".format(guess))
break
else:
if guess == secret_num:
print("You got it! My number was {}".format(secret_num))
break
elif guess < secret_num:
print("My number is higher than {}".format(guess))
else:
print("My number is lower than {}".format(guess))
guesses.append(guess)
else:
print("You didn't get it! My number was {}".format(secret_num))
play_again = input("Do you want to play again? Y/N ")
if play_again.lower() != "N":
game()
else:
print("Bye")
game()
2 Answers
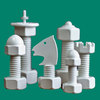
Steven Parker
230,995 Points
I also got that error, but only because it was accompanied by this one:
During handling of the above exception, another exception occurred:
UnboundLocalError: local variable 'guess' referenced before assignment
Your handler tried to reference guess, but the exception occurred before it was assigned. You may want to change your message to something simpler like this:
print("That isn't a number!")
Otherwise, you'd need to store the string from the prompt in a separate variable, and then convert it to int on another line.
Also, I noticed this line which can never be false, because anything lowered can never be upper case:
if play_again.lower() != "N":
You can either change lower to upper or change "N" to "n".
