Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial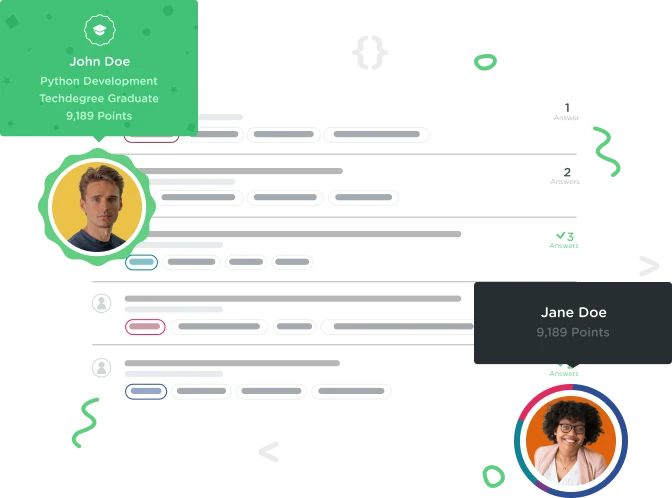

Cody Munster
1,710 PointsTrying to find an odd/even number using a function
Hi Guys,
I am stumped on this. I have run this as a program outside of a function and it returns a True for an even number and a False for an odd number. What i don't understand is why it won't work here?
The only thing i can think of is it has something to do with the argument in the function?
Thanks
def even_odd(num):
x = int(input("Please enter a number: "))
num = (x % 2)
if num == 0:
print(True)
else:
print(False)
1 Answer

Manish Giri
16,266 PointsThere are some problems with your code.
Look at the first instruction - "Write a function named even_odd that takes a single argument, a number.". You need to check whether this argument is even or odd. This argument is represented in your code by the variable
num
in this line -def even_odd(num):
.-
Now look at these lines -
x = int(input("Please enter a number: ")) num = (x % 2)
You're taking a input from the user, and assigning it to
num
. Thereby, you're overwriting the argument that was actually passed in to your function. Instead of this, you should directly be checking ifnum % 2
is 0 or not. -
For the final step, the challenge asks you to "return" a value - "return True if the number is even, or False if the number is odd.". You're instead printing
True
orFalse
-if num == 0: print(True) else: print(False)
Cody Munster
1,710 PointsCody Munster
1,710 PointsThanks a lot Manish. I ended up submitting the following
def even_odd(num):
Your help is much appreciated!
Christian A. Castro
30,501 PointsChristian A. Castro
30,501 PointsManish Giri Any idea what I might be doing wrong on my end?
Manish Giri
16,266 PointsManish Giri
16,266 PointsChristian A. Castro The problem in your code is the placement of the
start
variable. If I run your code, I get this error -That's because in the
even_odd
function,start
is considered as alocal
variable. You're usingstart
to execute awhile
loop, but inside the function, there's no local variablestart
with a value assigned to it, before it's used. Python doesn't know you're trying to refer to the global variablestart
.I'd recommend using this approach -